Microsoft Azure has a Redis Cache as a Service. There's two tiers. Basic is a single cache node, and Standard is as a complete replicated Cache (two nodes, with automatic failover). Microsoft manages automatic replication between the two nodes, and offers a high-availability SLA. The Premium tier can use up to a half-terabyte of RAM and tens of thousands of client connections and be clustered and scaled out to even bigger units. Sure, I could manage your own Redis in my own VM if I wanted to, but this is SAAS (Software as a Service) that I don't have to think about - I just use it and the rest is handled.
Microsoft Azure具有Redis缓存即服务。 有两层。 Basic是单个缓存节点,而Standard是完整的复制缓存(两个节点,具有自动故障转移功能)。 Microsoft管理两个节点之间的自动复制,并提供高可用性SLA。 Premium层最多可以使用半TB的RAM和数万个客户端连接,并且可以集群并扩展到更大的单元。 当然,如果需要的话,我可以在自己的VM中管理自己的Redis,但这是我不必考虑的SAAS(软件即服务),我只需使用它,其余的就可以处理了。
I blogged about Redis on Azure last year but wanted to try it in a new scenario now, using it as a cache for ASP.NET web apps. There's also an interesting open source Redis Desktop Manager I wanted to try out. Another great GUI for Redis is Redsmin.
去年,我在Azure上发布了有关Redis的博客,但现在想在新的情况下尝试将其用作ASP.NET Web应用程序的缓存。 还有一个有趣的开源Redis Desktop Manager 我想尝试一下。 Redis的另一个出色GUI是Redsmin 。
For small apps and sites I can make a Basic Redis Cache and get 250 megs. I made a Redis instance in Azure. It takes a minute or two to create. It's SSL by default. I can talk to it programmatically with something like StackExchange.Redis or ServiceStack.Redis or any of a LOT of other great client libraries.
对于小型应用程序和网站,我可以进行基本Redis缓存并获得250兆。 我在Azure中创建了Redis实例。 创建需要一两分钟。 默认情况下为SSL。 我可以使用StackExchange.Redis或ServiceStack.Redis之类的程序或许多其他出色的客户端库中的任何程序来与之交谈。
However, there's now great support for caching and Redis in ASP.NET. There's a library called Microsoft.Web.RedisSessionStateProvider that I can get from NuGet:
但是,现在ASP.NET中对缓存和Redis提供了强大的支持。 我可以从NuGet获得一个名为Microsoft.Web.RedisSessionStateProvider的库:
Install-Package Microsoft.Web.RedisSessionStateProvider
It uses the StackExchange library under the covers, but it enables ASP.NET to use the Session object and store the results in Redis, rather than in memory on the web server. Add this to your web.config:
它在后台使用了StackExchange库,但是它使ASP.NET可以使用Session对象,并将结果存储在Redis中,而不是存储在Web服务器上的内存中。 将此添加到您的web.config:
<sessionState mode="Custom" customProvider="FooFoo">
<providers>
<add name="MySessionStateStore"
type="Microsoft.Web.Redis.RedisSessionStateProvider"
host="hanselcache.redis.cache.windows.net"
accessKey="THEKEY"
ssl="true"
port="1234" />
</providers>
</sessionState>
Here's a string from ASP.NET Session stored in Redis as viewed in the Redis Desktop Manager. It's nice to use the provider as you don't need to change ANY code.
这是Redis桌面管理器中查看的来自Redis的ASP.NET Session中的字符串。 使用提供程序很好,因为您不需要更改任何代码。
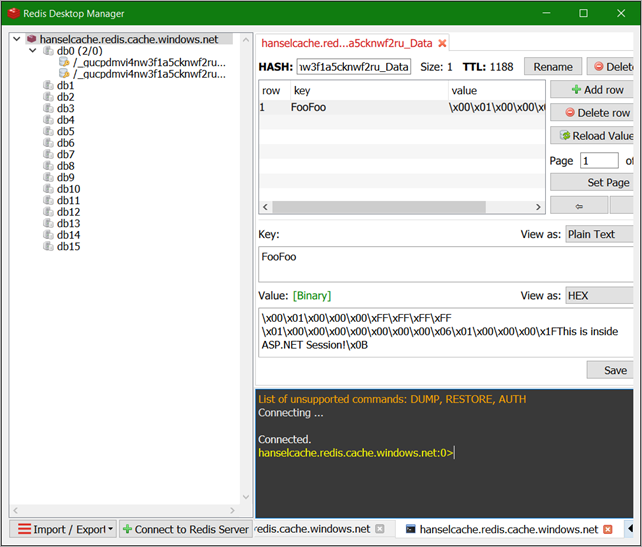
You can turn off SSL and connect to Azure Redis Cache over the open internet but you really should use SSL. There's instructions for using Redis Desktop Manager with SSL and Azure Redis. Note the part where you need a .pem file which is the Azure Redis Cache SSL public key. You can get that SSL key here as of this writing.
您可以关闭SSL并通过开放的Internet连接到Azure Redis缓存,但实际上应该使用SSL。 有关将Redis Desktop Manager与SSL和Azure Redis一起使用的说明。 请注意您需要.pem文件的部分,该文件是Azure Redis缓存SSL公共密钥。 在撰写本文时,您可以在此处获取该SSL密钥。
Not only can you use Redis for Session State, but you can also use it for a lightning fast Output Cache. That means caching full HTTP responses. Setting it up in ASP.NET 4.x is very similar to the Session State Provider:
您不仅可以将Redis用于会话状态,还可以将其用于闪电般的快速输出缓存。 这意味着缓存完整的HTTP响应。 在ASP.NET 4.x中进行设置与会话状态提供程序非常相似:
Install-Package Microsoft.Web.RedisOutputCacheProvider
Now when you use [OutputCache] attributes in MVC Controllers or OutputCache directives in Web Forms like <%@ OutputCache Duration="60" VaryByParam="*" %> the responses will be handled by Redis. With a little thought about how your query strings and URLs work, you can quickly take an app like a Product Catalog, for example, and make it 4x or 10x faster with caching. It's LOW effort and HIGH upside. I am consistently surprised even in 2015 how often I see folks going to the database on EVERY HTTP request when the app's data freshness needs just doesn't require the perf hit.
现在,当您在MVC控制器中使用[OutputCache]属性或在Web窗体中使用诸如<%@ OutputCache Duration =“ 60” VaryByParam =“ *”%>之类的OutputCache指令时,响应将由Redis处理。 稍微考虑一下查询字符串和URL的工作原理,您就可以快速开发一个像Product Catalog这样的应用程序,并通过缓存使其速度提高4倍或10倍。 这是省力而又高的上行空间。 即使在2015年,我也始终感到惊讶,当应用程序的数据新鲜度需求不要求使用性能优异时,我经常看到人们在每个HTTP请求中都去数据库。
You can work with Redis directly in code, of course. There's docs for .NET, Node.js, Java and Python on Azure. It's a pretty amazing project and having it be fully managed as a service is nice. From the Azure Redis site:
当然,您可以直接在代码中使用Redis。 在Azure上有.NET , Node.js , Java和Python的文档。 这是一个非常了不起的项目,可以很好地将其作为服务进行全面管理。 从Azure Redis网站:
Redis is an advanced key-value store, where keys can contain data structures such as strings, hashes,lists, sets and sorted sets. Redis supports a set of atomic operations on these data types.
Redis是高级键值存储,其中键可以包含数据结构,例如字符串,哈希,列表,集合和排序集合。 Redis支持对这些数据类型的一组原子操作。
Redis also supports trivial-to-setup master-slave replication, with very fast non-blocking first synchronization, auto-reconnection on net split and so forth.
Redis还支持琐碎的设置主从复制,具有非常快速的非阻塞第一次同步,网络拆分时自动重新连接等功能。
Other features include Transactions, Pub/Sub, Lua scripting, Keys with a limited time-to-live, and configuration settings to make Redis behave like a cache.
其他功能包括Transactions , Pub / Sub , Lua脚本,具有有限生存时间的键以及使Redis表现得像缓存一样的配置设置。
You can use Redis from most programming languages used today.
您可以从当今使用的大多数编程语言中使用Redis。
- Azure Redis Cache leverages Redis authentication and also supports SSL connections to Redis. Azure Redis缓存利用Redis身份验证,还支持与Redis的SSL连接。
Perhaps you're interested in Redis but you don't want to run it on Azure, or perhaps even on Linux. You can run Redis via MSOpenTech's Redis on Windows fork. You can install it from NuGet, Chocolatey or download it directly from the project github repository. If you do get Redis for Windows (super easy with Chocolatey), you can use the redis-cli.exe at the command line to talk to the Azure Redis Cache as well (of course!).
也许您对Redis感兴趣,但是您不想在Azure甚至Linux上运行它。 您可以在Windows fork上通过MSOpenTech的Redis运行Redis 。 您可以从NuGet , Chocolatey进行安装,也可以直接从项目github存储库下载。 如果确实获得了Windows版Redis(对Chocolatey来说非常容易),则可以在命令行上使用redis-cli.exe与Azure Redis缓存进行对话(当然!)。
It's easy to run a local Redis server with redis-server.exe, test it out in development, then change your app's Redis connection string when you deploy to Azure. Check it out. Within 30 min you may be able to configure your app to use a cache (Redis or otherwise) and see some really significant speed-up.
使用redis-server.exe运行本地Redis服务器很容易,在开发中对其进行测试,然后在部署到Azure时更改应用程序的Redis连接字符串。 看看这个。 在30分钟内,您可能可以将您的应用程序配置为使用缓存(Redis或其他方式),并看到一些非常明显的加速。
Sponsor: Big thanks to my friends at Octopus Deploy for sponsoring the feed this week. Build servers are great at compiling code and running tests, but not so great at deployment. When you find yourself knee-deep in custom scripts trying to make your build server do something it wasn't meant to, give Octopus Deploy a try.
赞助者:非常感谢我在Octopus Deploy上的朋友们为本周的提要提供赞助。 构建服务器擅长于编译代码和运行测试,但不适用于部署。 当您发现自己陷入定制脚本的深处,试图使您的构建服务器执行原本不想要的操作时,请尝试使用Octopus Deploy 。
翻译自: https://www.hanselman.com/blog/using-redis-as-a-service-in-azure-to-speed-up-aspnet-applications