#include <winternl.h>
#include <windows.h>
#include <iostream>
#define _CRT_SECURE_NO_WARNINGS
using namespace std;
#define SystemHandleInformation 0x10
#define STATUS_INFO_LENGTH_MISMATCH 0x004
#define HANDLE_TOTAL_COUNT 0X100000
#define MAX_FILENAME_SIZE 1024
typedef struct _SYSTEM_HANDLE_INFORMATION
{
ULONG ProcessId;
UCHAR ObjectTypeNumber;
UCHAR Flags;
USHORT Handle;
PVOID Object;
ACCESS_MASK GrantedAccess;
}SYSTEM_HANDLE_INFORMATION, * PSYSTEM_HANDLE_INFORMATION;
typedef struct _SYSTEM_HANDLE_INFORMATION_EX
{
ULONG NumberOfHandles;
SYSTEM_HANDLE_INFORMATION Information[HANDLE_TOTAL_COUNT];
}SYSTEM_HANDLE_INFORMATION_EX, * PSYSTEM_HANDLE_INFORMATION_EX;
typedef struct _FILE_NAME_INFORMATION {
ULONG FileNameLength;
WCHAR FileName[MAX_FILENAME_SIZE];
} FILE_NAME_INFORMATION, * PFILE_NAME_INFORMATION;
typedef struct _NM_INFO
{
HANDLE hFile;
FILE_NAME_INFORMATION Info;
} NM_INFO, * PNM_INFO;
typedef enum _RFILE_INFORMATION_CLASS {
FileDirectoryInformation1 = 1,
FileFullDirectoryInformation,
FileBothDirectoryInformation,
FileBasicInformation,
FileStandardInformation,
FileInternalInformation,
FileEaInformation,
FileAccessInformation,
FileNameInformation,
FileRenameInformation,
FileLinkInformation,
FileNamesInformation,
FileDispositionInformation,
FilePositionInformation,
FileFullEaInformation,
FileModeInformation,
FileAlignmentInformation,
FileAllInformation,
FileAllocationInformation,
FileEndOfFileInformation,
FileAlternateNameInformation,
FileStreamInformation,
FilePipeInformation,
FilePipeLocalInformation,
FilePipeRemoteInformation,
FileMailslotQueryInformation,
FileMailslotSetInformation,
FileCompressionInformation,
FileObjectIdInformation,
FileCompletionInformation,
FileMoveClusterInformation,
FileQuotaInformation,
FileReparsePointInformation,
FileNetworkOpenInformation,
FileAttributeTagInformation,
FileTrackingInformation,
FileIdBothDirectoryInformation,
FileIdFullDirectoryInformation,
FileValidDataLengthInformation,
FileShortNameInformation,
FileIoCompletionNotificationInformation,
FileIoStatusBlockRangeInformation,
FileIoPriorityHintInformation,
FileSfioReserveInformation,
FileSfioVolumeInformation,
FileHardLinkInformation,
FileProcessIdsUsingFileInformation,
FileNormalizedNameInformation,
FileNetworkPhysicalNameInformation,
FileIdGlobalTxDirectoryInformation,
FileIsRemoteDeviceInformation,
FileUnusedInformation,
FileNumaNodeInformation,
FileStandardLinkInformation,
FileRemoteProtocolInformation,
FileRenameInformationBypassAccessCheck,
FileLinkInformationBypassAccessCheck,
FileVolumeNameInformation,
FileIdInformation,
FileIdExtdDirectoryInformation,
FileReplaceCompletionInformation,
FileHardLinkFullIdInformation,
FileIdExtdBothDirectoryInformation,
FileMaximumInformation
} FILE_INFORMATION_CLASS, * PFILE_INFORMATION_CLASS;
typedef struct _IO_STATUS_BLOCK {
union {
NTSTATUS Status;
PVOID Pointer;
} DUMMYUNIONNAME;
ULONG_PTR Information;
} IO_STATUS_BLOCK, * PIO_STATUS_BLOCK;
typedef NTSTATUS(WINAPI* ZWQUERYINFORMATIONFILE)(HANDLE, PIO_STATUS_BLOCK, PVOID, ULONG, FILE_INFORMATION_CLASS);
typedef DWORD(WINAPI* NTQUERYSYSTEMINFORMATION)(DWORD, PVOID, DWORD, PDWORD);
NTQUERYSYSTEMINFORMATION NtQuerySystemInformation = 0;
ZWQUERYINFORMATIONFILE ZwQueryInformationFile = 0;
string GetFileName(PNM_INFO lpParameter)
{
PNM_INFO NmInfo = (PNM_INFO)lpParameter;
IO_STATUS_BLOCK IoStatus;
ZwQueryInformationFile(NmInfo->hFile, &IoStatus, &NmInfo->Info, MAX_FILENAME_SIZE, FILE_INFORMATION_CLASS::FileNameInformation);
if (NmInfo->Info.FileNameLength != 0)
{
char ascfn[1024];
int asclen = WideCharToMultiByte(CP_ACP, 0, NmInfo->Info.FileName, NmInfo->Info.FileNameLength, ascfn, 1024, 0, 0);
if (asclen > 0)
{
*(ascfn + asclen) = 0;
return string(ascfn);
}
}
return "";
}
int main(int argc, char** argv)
{
int result = 0;
HMODULE hNtDll = LoadLibrary(L"ntdll.dll");
if (hNtDll == 0) {
return FALSE;
}
NtQuerySystemInformation = (NTQUERYSYSTEMINFORMATION)GetProcAddress(hNtDll, "ZwQuerySystemInformation");
ZwQueryInformationFile = (ZWQUERYINFORMATIONFILE)GetProcAddress(hNtDll, "ZwQueryInformationFile");
FILE* fp = fopen("test.txt", "ab+");
const char* mystring = "hello world!\r\n";
fwrite(mystring, 1, strlen(mystring), fp);
ULONG cbBuffer = sizeof(SYSTEM_HANDLE_INFORMATION_EX);
LPVOID pBuffer = (LPVOID)malloc(cbBuffer);
if (pBuffer)
{
NtQuerySystemInformation(SystemHandleInformation, pBuffer, cbBuffer, NULL);
PSYSTEM_HANDLE_INFORMATION_EX pInfo = (PSYSTEM_HANDLE_INFORMATION_EX)pBuffer;
for (ULONG r = 0; r < pInfo->NumberOfHandles; r++)
{
ULONG ProcessId;
UCHAR ObjectTypeNumber;
UCHAR Flags;
USHORT Handle;
PVOID Object;
ACCESS_MASK GrantedAccess;
if (pInfo->Information[r].ObjectTypeNumber /*== 35*/)
{
NM_INFO nmInfo = { 0 };
nmInfo.hFile = (HANDLE)pInfo->Information[r].Handle;
string fileName = GetFileName(&nmInfo);
if (fileName != "")
{
printf("name:%s\r\n", fileName.c_str());
}
}
}
free(pBuffer);
}
FreeModule(hNtDll);
fclose(fp);
result = getchar();
return 0;
}
获取本进程所有句柄和资源
于 2023-03-09 18:39:28 首次发布
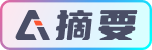