一、常见电路
1、 分频电路
1)二分频电路
library ieee;
use ieee.std_logic_1164.all;
entity example is
port(clock : in std_logic;
clkout : out std_logic
);
end example;
architecture behave of example is
signal clk: std_logic;
begin
process(clock)
begin
if rising_edge(clock) then
clk<=not clk;
end if;
end process;
clkout<=clk;
end behave;
2)偶数倍分频电路(8分频)
library ieee;
use ieee.std_logic_1164.all;
entity FreDevider is
port
(clkin : in std_logic;
clkout : out std_logic
);
end;
architecture Devider of FreDevider is
constant N :integer := 3;
signal counter : integer range 0 to N;
signal clk : std_logic;
begin
process(clkin)
begin
if rising_edge(clkin) then
if counter=N then
counter<=0;
clk<=not clk;
else
counter<=counter+1;
end if;
end if;
end process
clout<=clk;
end;
3) 奇数倍分频(3分频)
library ieee;
use ieee.std_logic_1164.all;
entity Fredevider3 is
port
(clkin : in std_logic;
clkout : out std_logic
);
end;
architecture Devider of Fredevider3 is
signal counter :integer range 0 to 2;
signal temp1,temp2 : std_logic;
begin
process(clkin)
begin
if rising_edge(clkin) then
if counter=2 then
counter<=0;
temp1<=not temp1;
else
counter<=counter+1;
end if;
end if;
if falling_edge(clkin) then
if counter=1 then
temp2<=not temp2;
end if;
end if;
end process;
clkout<=temp1 xor temp2;
end;
2、计数器
library ieee;
use ieee.std_logic_1164.all;
entity example is
port(clk : in std_logic;
reset : in std_logic;
num : buffer integer range 0 to 3
);
end example;
architecture behave of example is
begin
process(clk)
begin
if reset='1' then
num<=0;
elsif rising_edge(clk) then
if num=3 then
num<=0;
else
num<=num+1;
end if;
end if;
end process;
end behave;
3、简单并-串转换
library ieee;
use ieee.std_logic_1164.all;
entity example is
port(clk : in std_logic;
reset : in std_logic;
parallelnum : in std_logic_vector(31 downto 0);
serialout :out std_logic
);
end example;
architecture behave of example is
begin
process(clk)
variable i :integer range 0 to 31;
begin
if reset='1' then
i:=0;
elsif rising_edge(clk) then
serialout<=parallelnum(i);
if i<31 then
i:=i+1;
end if;
end if;
end process;
end behave;
4、时钟产生
(1)方法1
process
begin
clk <= not clk;
wait for 10ns;
end process;
(2)方法2
process
begin
clk <= not clk after 10ns;
wait on clk;
end process;
(3)方法3
process(clk)
begin
clk <= not clk after 10ns;
end process;
(4)方法4
clk <= not clk after 10ns;
5、七段数码管驱动电路
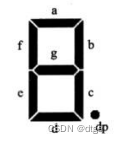
1111110 | 0 |
0110000 | 1 |
1101101 | 2 |
1111001 | 3 |
0110011 | 4 |
1011011 | 5 |
1011111 | 6 |
1110000 | 7 |
1111111 | 8 |
1111011 | 9 |
library ieee;
use ieee.std_logic_1164.all;
entity example is
port(num : in integer range 0 to 15;
display : out std_logic_vector(6 downto 0)
);
end;
architecture behave of example is
begin
with num select
display<="1111110" when 0,
"0110000" when 1,
"1101101" when 2,
"1111001" when 3,
"0110011" when 4,
"1011011" when 5,
"1011111" when 6,
"1110000" when 7,
"1111111" when 8,
"1111011" when 9,
"0000000" when others;
end
6、同步整形电路
(1)上升沿输出脉冲信号
library ieee;
use ieee.std_logic_1164.all;
entity SignalLatch is
port(clk : in std_logic;
signalin : in std_logic;
signalout : out std_logic
);
end;
architecture Dataflow of SignalLatch is
signal q0,q1 : std_logic;
begin
process(clk)
begin
if rising_edge(clk) then
q0<=signalin;
q1<=q0;
end if;
end process;
signalout<=q0 and (not q1);
end;
(2)下降沿处输出脉冲信号
library ieee;
use ieee.std_logic_1164.all;
entity SignalLatch is
port(clk : in std_logic;
signalin : in std_logic;
signalout : out std_logic
);
end;
architecture Dataflow of SignalLatch is
signal clear,s : std_logic;
begin
process(signalin)
begin
if clear='1' then
s<='0';
elsif rising_edge(signalin) then
s<='1';
end if;
end process;
process(clk)
begin
if falling_edge(clk) then
if s='1' then
signalout<='1';
clear<='1';
else
signalout<='0';
clear<='0';
end if;
end if;
end process;
end;
7、键盘扫描
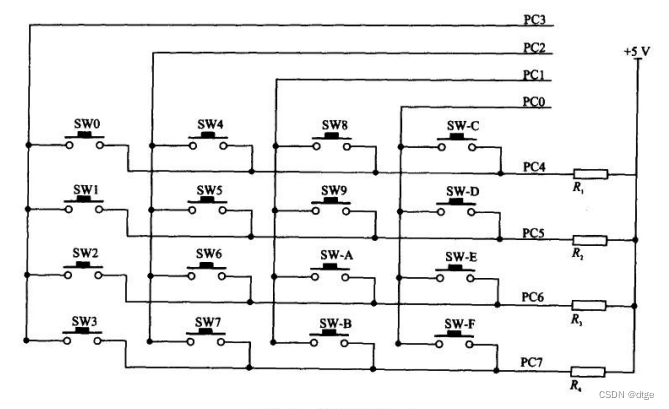
列/PC3~PC0(输出) | 行/PC7~PC4(输入) | 按键 |
0111 | 1110 | 0 |
0111 | 1101 | 1 |
0111 | 1011 | 2 |
0111 | 0111 | 3 |
1011 | 1110 | 4 |
1011 | 1101 | 5 |
1011 | 1011 | 6 |
1011 | 0111 | 7 |
1101 | 1110 | 8 |
1101 | 1101 | 9 |
1101 | 1011 | A |
1101 | 0111 | B |
1110 | 1110 | C |
1110 | 1101 | D |
1110 | 1011 | E |
1110 | 0111 | F |
library ieee;
use ieee.std_logic_1164.all;
entity Keyboard is
port
(
clk : in std_logic;
kin : in std_logic_vector(0 to 3);
scansignal : out std_logic_vector(0 to 3);
num : out integer range 0 to 15
);
end;
architecture scan of Keyboard is
signal scans : std_logic_vector(0 to 7);
signal scn : std_logic_vector(0 to 3);
signal counter : integer range 0 to 3;
signal counterB : integer range 0 to 3;
begin
process(clk)
begin
if rising_edge(clk) then
if counter=3 then
counter<=0;
else
counter<=counter+1;
end if;
case counter is --产生扫描信号
when 0 =>scn<="1000";
when 1 =>scn<="0100";
when 2 =>scn<="0010";
when 3 =>scn<="0001";
end case;
end if;
end process;
pocess(clk)
begin
if falling_edge(clk) then --上升沿产生扫描信号,下降沿读入行码
if kin="0000" then --“0000”表示无按下
if counterB=3 then
num<=15; --15为无效值
counterB<=0;
else
counterB<=counterB+1;
end if;
else
counterB<=0;
case scans is
when "10000001"=>num<=0;
when "10000010"=>num<=1;
when "10000100"=>num<=2;
when "10001000"=>num<=3;
when "01000001"=>num<=4;
when "01000010"=>num<=5;
when "01000100"=>num<=6;
when "01001000"=>num<=7;
when "00100001"=>num<=8;
when "00100010"=>num<=9;
when "00100100"=>num<=10;
when "00101000"=>num<=11;
when "00010001"=>num<=12;
when "00010010"=>num<=13;
when "00010100"=>num<=14;
when others=>num<=num;
end case;
end if;
end if;
end process;
scans<=scn&kin;
scansignal<=scn;
end;
8、键盘消抖电路
library ieee;
use ieee.std_logic_1164.all;
entity Antitwitter is
port( clk : in std_logic;
numin : in integer range 0 to 15;
numout : out integer range 0 to 15);
end;
architecture Behavior of Antitwitter is
signal tempnum integer range 0 to 15;
signal counter : integer range 0 to 31;
signal start : std_logic;
begin
process(clk)
begin
if rising_edge(clk) then
if start='0' then
tempnum<=15;
numout<=15;
start<='1'
else
if numin /= tempnum then
tempnum<=numin;
counter<=0;
else
if counter=31 then
numout<=numin;
counter<=0;
else
counter<=counter+1;
end if;
end if;
end if;
end if;
end process;
end;