1、全局函数作为线程参数传递
int mySonThread()
{
cout << "子线程:开始" << endl;
for (int i = 0; i < 10; i++) {
cout << "子线程执行第:" << i << " 次" << endl;
}
cout << "子线程结束" << endl;
return 0;
}
void example1()
{
thread thread1(mySonThread);
thread1.join();
}
2、类对象作为线程参数传递
class SonTheardClass
{
public:
SonTheardClass(int i) : num(i)
{
cout << "构造函数被执行,线程ID:" << this_thread::get_id() << endl;
}
SonTheardClass(const SonTheardClass &mysonthread) : num(mysonthread.num)
{
cout << "拷贝构造被执行,线程ID:" << this_thread::get_id() << endl;
}
void operator()()
{
cout << "mySonTheardClass is begining!" << endl;
cout << "作为类参数对象被执行,线程ID:" << this_thread::get_id()
<< endl;
cout << "mySonTheardClass is over!" << endl;
}
~SonTheardClass()
{
cout << "析构被执行,线程ID" << this_thread::get_id() << endl;
}
public:
int num;
};
void example2()
{
SonTheardClass mySonTheardClass(6);
thread thread2(mySonTheardClass);
thread2.join();
}
3、传参问题
- 传递值引用时:可以观察到addr_i_thread与addr_i所表示的地址不一样,因此可以断定,当线程中即使传入引用,其实并不是真实的引用,也是值传递,因为将i又额外的复制了一份存入到不同的地址中,当主线程结束,不会影响子线程
- 传递指针时: 可以观察到,当线程传入字符串指针时,addr_buff_thread与addr_buff一致,也就是说两个线程共用一个内存,如果主线程结束,那么子线程就会出现错误
- 传递字符串时:可以观察到当传入string引用时,则addr_buff_thread与addr_buff不一致,也就是两个线程没有共用一个内存;如果采用值传递的方式,则会发现addr_buff_thread与addr_buff是一致的,说明两个线程公用一个内存。因此,使用线程在传字符串时,要使用string&;
- 传递临时对象时:要保证当线程使用这个临时对象时,主线程没有将它回收。
#include <iostream>
#include <unistd.h>
#include <thread>
#include "stdio.h"
#include <string>
using namespace std;
void mytempthread(const int &i, char *buff1, const string &buff2)
{
cout << "mytempthread is begining!" << endl;
cout << "i= " << i << endl;
const int *addr_i_thread = &i;
cout
<< "addr_i_thread= " << addr_i_thread
<< endl;
printf(
"addr_buff_pointer_thread= %lx\n",
buff1);
printf(
"addr_buff_ref_thread= %lx\n",
&buff2);
cout << "mytempthread is over!" << endl;
}
void example3()
{
int i = 2;
const int *addr_i = &i;
cout << "addr_i= " << addr_i << endl;
char buff[] = "temp buff";
cout << "addr_buff= " << &buff << endl;
thread thread3(
mytempthread, i, buff,
string(
buff));
thread3.join();
}
int main(int argc, char *argv[])
{
cout << "主线程:开始"
<< endl;
example3();
cout << "主线程:结束" << endl;
return 0;
}
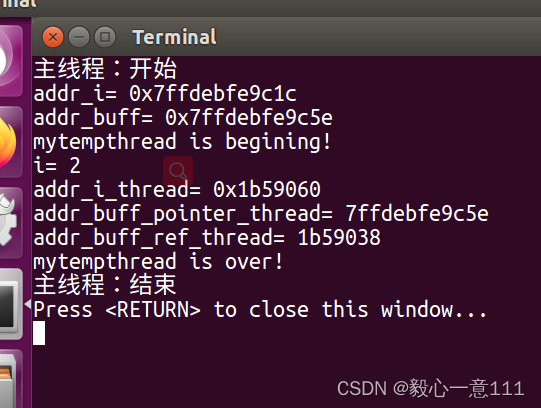