数据结构 | |
struct | osTimerAttr_t |
定时器的属性结构体。更多... | |
类型定义 | |
typedef void * | osTimerId_t |
typedef void(* | osTimerFunc_t )(void *argument) |
定时器回调函数。更多... | |
枚举 | |
enum | osTimerType_t { osTimerOnce = 0, osTimerPeriodic = 1 } |
定时器类型。更多... | |
函数 | |
osTimerId_t | osTimerNew (osTimerFunc_t func, osTimerType_t type, void *argument, const osTimerAttr_t *attr) |
创建并初始化一个定时器。更多... | |
const char * | osTimerGetName (osTimerId_t timer_id) |
获取定时器的名称。更多... | |
osStatus_t | osTimerStart (osTimerId_t timer_id, uint32_t ticks) |
启动或重新启动定时器。更多... | |
osStatus_t | osTimerStop (osTimerId_t timer_id) |
停止一个定时器。更多... | |
uint32_t | osTimerIsRunning (osTimerId_t timer_id) |
检查定时器是否正在运行。更多... | |
osStatus_t | osTimerDelete (osTimerId_t timer_id) |
删除一个定时器。更多... | |
描述
除通用等待功能外,CMSIS-RTOS 还支持虚拟定时器对象。这些定时器对象可以触发函数的执行(不是线程)。当定时器到期时,会执行回调函数以运行与定时器相关的代码。每个定时器可以配置为单次定时器或定时器。定期定时器重复其操作,直到它被删除或停止。所有定时器都可以启动,重新启动或停止。
-
注意
- RTX 在线程 osRtxTimerThread 中处理定时器。回调函数在此线程的控制下运行,并可能使用其他 CMSIS-RTOS API 调用。 osRtxTimerThread 在定时器配置中配置。
- 定时器管理功能不能从中断服务程序调用。
下图显示了周期性定时器的行为。对于单次定时器,定时器在执行回调函数后停止。
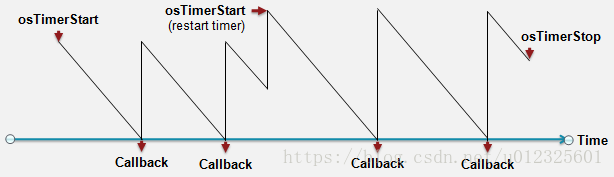
使用定时器
使用软件定时器需要以下步骤:
- 定义定时器:
osTimerId_t one_shot_id, periodic_id;
- 定义回调函数:
static void one_shot_Callback ( void *argument) {int32_t arg = (int32_t)argument; // cast back argument '0'// do something, i.e. set thread/event flags}static void periodic_Callback ( void *argument) {int32_t arg = (int32_t)argument; // cast back argument '5'// do something, i.e. set thread/event flags}
- 实例化并启动定时器:
// creates a one-shot timer:one_shot_id = osTimerNew(one_shot_Callback, osTimerOnce, ( void *)0, NULL); // (void*)0 is passed as an argument// to the callback function// creates a periodic timer:periodic_id = osTimerNew(periodic_Callback, osTimerPeriodic, ( void *)5, NULL); // (void*)5 is passed as an argument// to the callback functionosTimerStart(one_shot_id, 500);osTimerStart(periodic_id, 1500);// start the one-shot timer again after it has triggered the first time:osTimerStart(one_shot_id, 500);// when timers are not needed any longer free the resources:osTimerDelete(one_shot_id);osTimerDelete(periodic_id);
数据结构文档
struct osTimerAttr_t |
数据字段 | ||
---|---|---|
const char * | name | 定时器的名称 指向具有人类可读名称的定时器对象的字符串。 |
uint32_t | attr_bits | 属性位 保留以供将来使用(设为 '0')。 |
void * | cb_mem | 内存控制块 指向定时器控制块对象的内存位置。这可以选择用于自定义内存管理系统。 |
uint32_t | cb_size | 为控制块提供的内存大小 内存块的大小与 cb_mem 一起传递。必须是定时器控制块对象的大小或更大。 |
类型文档
void(* osTimerFunc_t)(void *argument) |
定时器回调函数每当定时器超时时被调用。
回调可以在专用的定时器线程或中断上下文中执行。因此,建议仅使用定时器回调中的 ISR 可调用函数。
-
参数
-
[in] argument 提供给 osTimerNew 的参数。
枚举类型文档
enum osTimerType_t |
osTimerType_t 指定函数 osTimerNew 的重复(周期性)或一次性定时器。
枚举 | |
---|---|
osTimerOnce | 一次性定时器。 一旦定时器超时,定时器不会自动重新启动。它可以根据需要使用 osTimerStart 手动重新启动。 |
osTimerPeriodic | 重复定时器。 定时器会自动重复并在运行过程中持续触发回调,请参阅 osTimerStart 和 osTimerStop 。 |
函数文档
osTimerId_t osTimerNew | ( | osTimerFunc_t | func, |
osTimerType_t | type, | ||
void * | argument, | ||
const osTimerAttr_t * | attr | ||
) |
-
参数
-
[in] func 函数指向回调函数。 [in] type osTimerOnce 用于一次性或 osTimerPeriodic用于定期行为。 [in] argument 定时器回调函数的参数。 [in] attr 定时器属性; NULL:默认值。
-
返回
- 定时器 ID 以供其他函数参考,或者在出现错误时为 NULL 。
函数 osTimerNew 创建一个单次或定期定时器,并将其与具有参数的回调函数关联。定时器处于停止状态,直到用 osTimerStart 启动。在 RTOS 启动(调用 osKernelStart)之前,可以安全地调用该函数,但在初始化之前(调用 osKernelInitialize)不可以安全地调用该函数。
函数 osTimerNew 返回指向定时器对象标识符的指针,或者在出现错误时返回 NULL 。
-
注意
- 该函数不能从中断服务程序调用。
代码示例
*const char * osTimerGetName | ( | osTimerId_t | timer_id | ) |
-
参数
-
[in] timer_id 定时器 ID 由 osTimerNew 获得。
-
返回
- 名称为 NULL 终止的字符串。
函数 osTimerGetName 返回指向由参数 timer_id 标识的定时器的名称字符串的指针,或者在出现错误时返回 NULL 。
-
注意
- 该函数不能从中断服务程序调用。
osStatus_t osTimerStart | ( | osTimerId_t | timer_id, |
uint32_t | ticks | ||
) |
-
返回
- 状态代码,指示该函数的执行状态。
函数 osTimerStart 启动或重新启动由参数 timer_id 指定的定时器。参数刻度指定了时间滴答的定时器的值。
可能的 osStatus_t 返回值:
- osOK: 指定的定时器已启动或重新启动。
- osErrorISR: osTimerStart 不能从中断服务例程调用。
- osErrorParameter: 参数 timer_id 是 NULL 或无效或者滴答不正确。
- osErrorResource: 由参数 timer_id 指定的定时器处于无效定时器状态。
-
注意
- 该函数不能从中断服务程序调用。
代码示例
osStatus_t osTimerStop | ( | osTimerId_t | timer_id | ) |
-
参数
-
[in] timer_id 定时器 ID 由 osTimerNew 获得。
-
返回
- 状态代码,指示该函数的执行状态。
函数 osTimerStop 停止由参数 timer_id 指定的定时器。
可能的 osStatus_t 返回值:
- osOK: 指定的定时器已停止。
- osErrorISR: osTimerStop 不能从中断服务例程调用。
- osErrorParameter: 参数 timer_id 是 NULL 或无效。
- osErrorResource: 由参数 timer_id 指定的定时器未运行(您只能停止正在运行的定时器)。
-
注意
- 该函数不能从中断服务程序调用。
代码示例
uint32_t osTimerIsRunning | ( | osTimerId_t | timer_id | ) |
-
参数
-
[in] timer_id 定时器 ID 由 osTimerNew 获得。
-
返回
- 0 没有运行,1 运行。
函数 osTimerIsRunning 检查参数 timer_id 指定的定时器是否正在运行。如果定时器正在运行,则返回 1 ,如果定时器停止或发生错误,则返回 0 。
-
注意
- 该函数不能从中断服务程序调用。
osStatus_t osTimerDelete | ( | osTimerId_t | timer_id | ) |
-
参数
-
[in] timer_id 定时器 ID 由 osTimerNew 获得。
-
返回
- 状态代码,指示该函数的执行状态。
函数 osTimerDelete 删除由参数 timer_id 指定的定时器。
可能的 osStatus_t 返回值:
- osOK: 指定的定时器已被删除。
- osErrorISR: osTimerDelete 不能从中断服务程序调用。
- osErrorParameter: 参数 timer_id 是 NULL 或无效。
- osErrorResource: 由参数 timer_id 指定的定时器处于无效定时器状态。
-
注意
- 该函数不能从中断服务程序调用。
代码示例