//Enable TIMER2 clock
rcu_periph_clock_enable(RCU_TIMER2);
gpio_init(GPIOB,GPIO_MODE_AF_PP,GPIO_OSPEED_50MHZ,GPIO_PIN_4);
gpio_pin_remap_config(GPIO_SWJ_SWDPENABLE_REMAP,ENABLE);
//gpio_pin_remap_config(GPIO_SWJ_NONJTRST_REMAP,DISABLE);
gpio_pin_remap_config(GPIO_TIMER2_PARTIAL_REMAP,ENABLE); //
//PWM CP
/*
brief configure the TIMER peripheral
param[in] none
param[out] none
retval none
*/
void timer2_init(void)
{
/* TIMER1 configuration: generate PWM signals with different duty cycles:
TIMER1CLK = SystemCoreClock / 120 = 1MHz */
/* 定义一个定时器初始化结构体 */
timer_parameter_struct timer_init_struct;
/* 定义一个定时器输出比较参数结构体*/
timer_oc_parameter_struct timer_oc_init_struct;
/* PWM信号电平跳变值 */
uint16_t CH0CV_Val = 500;
// uint16_t CH1CV_Val = 375;
// uint16_t CH2CV_Val = 250;
// uint16_t CH3CV_Val = 125;
/* -----------------------------------------------------------------------
TIMER2 Channel0 duty cycle = (TIMER2_CH0CV/ TIMER2_CAR+1)* 100% = 50%
TIMER2 Channel1 duty cycle = (TIMER2_CH1CV/ TIMER2_CAR+1)* 100% = 37.5%
TIMER2 Channel2 duty cycle = (TIMER2_CH2CV/ TIMER2_CAR+1)* 100% = 25%
TIMER2 Channel3 duty cycle = (TIMER2_CH3CV/ TIMER2_CAR+1)* 100% = 12.5%
----------------------------------------------------------------------- */
//Enable TIMER2 clock
rcu_periph_clock_enable(RCU_TIMER2);
/* 开启复用功能时钟 */
timer_deinit(TIMER2);
/* TIMER2 configuration */
timer_init_struct.prescaler = 0;
timer_init_struct.alignedmode = TIMER_COUNTER_EDGE;
timer_init_struct.counterdirection = TIMER_COUNTER_UP;
timer_init_struct.period = 999;
timer_init_struct.clockdivision = TIMER_CKDIV_DIV1;
timer_init_struct.repetitioncounter = 0;
timer_init(TIMER2, &timer_init_struct);
/* PWM初始化 */
timer_oc_init_struct.outputstate = TIMER_CCX_ENABLE; /* 通道使能 */
timer_oc_init_struct.outputnstate = TIMER_CCXN_DISABLE; /* 通道互补输出使能(定时器2无效) */
timer_oc_init_struct.ocpolarity = TIMER_OC_POLARITY_HIGH; /* 通道极性 */
timer_oc_init_struct.ocnpolarity = TIMER_OCN_POLARITY_HIGH;/* 互补通道极性(定时器2无效)*/
timer_oc_init_struct.ocidlestate = TIMER_OC_IDLE_STATE_LOW;/* 通道空闲状态输出(定时器2无效)*/
timer_oc_init_struct.ocnidlestate = TIMER_OCN_IDLE_STATE_LOW;/*互补通道空闲状态输出(定时器2无效) */
/* PWM Mode configuration: Channel0 */
timer_channel_output_config(TIMER2, TIMER_CH_0, &timer_oc_init_struct);
/* 通道2占空比设置 */
timer_channel_output_pulse_value_config(TIMER2, TIMER_CH_0, CH0CV_Val);
/* PWM模式0 */
timer_channel_output_mode_config(TIMER2,TIMER_CH_0,TIMER_OC_MODE_PWM0);
/* 不使用输出比较影子寄存器 */
timer_channel_output_shadow_config(TIMER2,TIMER_CH_0,TIMER_OC_SHADOW_DISABLE);
/* PWM Mode configuration: Channel1 */
// timer_channel_output_config(TIMER2, TIMER_CH_1, &timer_oc_init_struct);
//
// /* 通道2占空比设置 */
// timer_channel_output_pulse_value_config(TIMER2, TIMER_CH_1, CH1CV_Val);
// /* PWM模式0 */
// timer_channel_output_mode_config(TIMER2,TIMER_CH_1,TIMER_OC_MODE_PWM0);
// /* 不使用输出比较影子寄存器 */
// timer_channel_output_shadow_config(TIMER2,TIMER_CH_1,TIMER_OC_SHADOW_DISABLE);
// /* PWM Mode configuration: Channel2 */
// timer_channel_output_config(TIMER2, TIMER_CH_2, &timer_oc_init_struct);
//
// /* 通道2占空比设置 */
// timer_channel_output_pulse_value_config(TIMER2, TIMER_CH_2, CH2CV_Val);
// /* PWM模式0 */
// timer_channel_output_mode_config(TIMER2,TIMER_CH_2,TIMER_OC_MODE_PWM0);
// /* 不使用输出比较影子寄存器 */
// timer_channel_output_shadow_config(TIMER2,TIMER_CH_2,TIMER_OC_SHADOW_DISABLE);
// /* PWM Mode configuration: Channel3 */
// timer_channel_output_config(TIMER2, TIMER_CH_3, &timer_oc_init_struct);
//
// /* 通道2占空比设置 */
// timer_channel_output_pulse_value_config(TIMER2, TIMER_CH_3, CH3CV_Val);
// /* PWM模式0 */
// timer_channel_output_mode_config(TIMER2,TIMER_CH_3,TIMER_OC_MODE_PWM0);
// /* 不使用输出比较影子寄存器 */
// timer_channel_output_shadow_config(TIMER2,TIMER_CH_3,TIMER_OC_SHADOW_DISABLE);
/* 自动重装载影子比较器使能 */
timer_auto_reload_shadow_enable(TIMER2);
timer_interrupt_enable(TIMER2, TIMER_INT_UP);
/* TIMER2 enable */
timer_enable(TIMER2);
}
/* TIMER2 中断服务函数
* 参数:无
* 返回值:无 */
void TIMER2_IRQHandler(void)
{
static uint8_t led_flag = 0;
if(timer_interrupt_flag_get(TIMER2, TIMER_INT_FLAG_UP))
{
/* 清除TIMER5 中断标志位 */
timer_interrupt_flag_clear(TIMER2, TIMER_INT_FLAG_UP);
led_flag = !led_flag;
}
}
GD32F303-PB4作为TIMER2+PWM输出
于 2022-06-10 22:28:08 首次发布
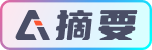