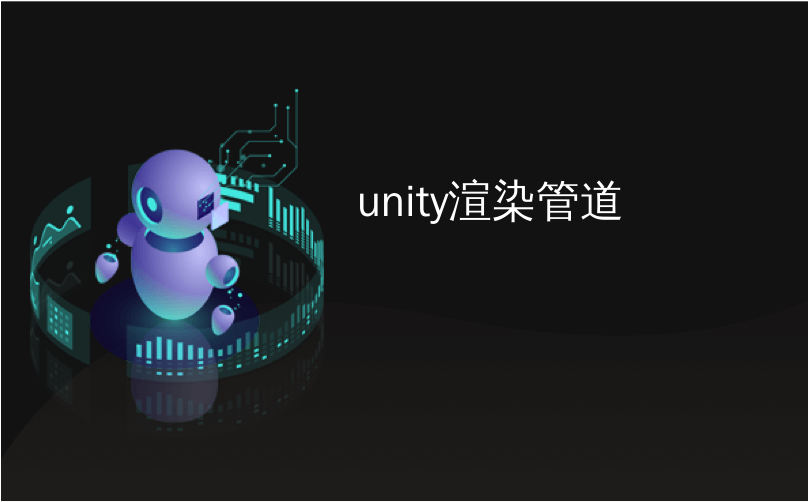
unity渲染管道
In Unity 5 we’ve been adding many user-visible graphics features (new Standard shader, realtime global illumination, reflection probes, new lightmapping workflow and so on), but we’ve also worked on rendering internals. Besides typical things like “optimizing it” (e.g. multithreaded light culling) and “making it more consistent” (e.g. more consistently between Linear & Gamma color spaces), we’ve also looked at how to make it more extensible.
在Unity 5中,我们已经添加了许多用户可见的图形功能(新的标准着色器 ,实时全局照明 ,反射探针,新的光照贴图工作流程等) ,但是我们还致力于内部渲染。 除了“优化” (例如,多线程光剔除)和“使其更加一致” (例如,在Linear和Gamma颜色空间之间更加一致)之类的典型事情之外,我们还研究了如何使其更具可扩展性 。
Internally and within the beta testing group we’ve discussed various approaches. A lot of ideas were thrown around: more script callbacks, assembling small “here’s a list of things to do” buffers, ability to create complete rendering pipelines from scratch, some sort of visual tree/graph rendering pipeline construction tools and so on. For Unity 5, we settled on ability to create “list of things to do” buffers, which we dubbed “Command Buffers“.
在Beta测试小组内部和内部,我们讨论了各种方法。 提出了很多想法:更多的脚本回调,组装小的“这里有要做的事情”缓冲区,从头开始创建完整渲染管道的能力,某种可视化树/图形渲染管道构建工具等。 对于Unity 5,我们确定了创建“待办事项列表”缓冲区的能力,我们将其称为“ 命令缓冲区 ”。
A command buffer in graphics is a low-level list of commands to execute. For example, 3D rendering APIs like Direct3D or OpenGL typically end up constructing a command buffer that is then executed by the GPU. Unity’s multi-threaded renderer also constructs a command buffer between a calling thread and the “worker thread” that submits commands to the rendering API.
图形中的命令缓冲区是要执行的命令的低级列表。 例如,像Direct3D或OpenGL这样的3D渲染API通常最终会构造一个命令缓冲区,然后由GPU执行该命令缓冲区。 Unity的多线程渲染器还在调用线程和“工作线程”之间构造命令缓冲区,该命令缓冲区将命令提交给渲染API。
In our case the idea is very similar, but the “commands” are somewhat higher level. Instead of containing things like “set internal GPU register X to value Y”, the commands are “Draw this mesh with that material” and so on.
在我们的案例中,这个想法非常相似,但是“命令”的层次更高。 而不是包含“将内部GPU寄存器X设置为值Y”之类的命令,而是“用该材质绘制此网格”等等。
From your scripts, you can create command buffers and add rendering commands to them (“set render target, draw mesh, …”). Then these command buffers can be set to execute at various points during camera rendering.
在脚本中,您可以创建命令缓冲区并向其添加渲染命令(“设置渲染目标,绘制网格,...”)。 然后,可以将这些命令缓冲区设置为在相机渲染期间的各个时间点执行。
For example, you could render some additional objects into deferred shading G-buffer after all regular objects are done. Or render some clouds immediately after skybox is drawn, but before anything else. Or render custom lights (volume lights, negative lights etc.) into deferred shading light buffer after all regular lights are done. And so on; we think there are a lot of interesting ways to use them.
例如,您可以在完成所有常规对象后,将一些其他对象渲染到延迟着色G缓冲区中。 或在绘制天空盒后立即渲染一些云,但在其他之前。 或在完成所有常规照明后,将自定义照明(体积照明,负照明等)渲染到延迟的阴影光缓冲区中。 等等; 我们认为有很多有趣的方式来使用它们。
Take a look at CommandBuffer and CameraEvent pages in the scripting API documentation.
查看脚本API文档中的CommandBuffer和CameraEvent页面。
图片还是没有发生! (Pictures or it did not happen!)
Ok, ok.
好的好的。
For example, we could do blurry refractions:
例如,我们可以进行模糊折射 :
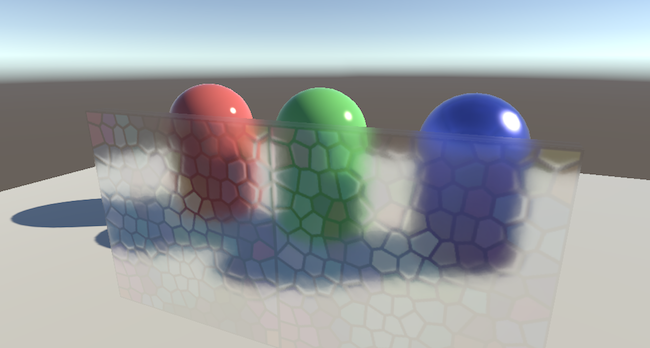
After opaque objects and skybox is rendered, current image is copied into a temporary render target, blurred and set up as a global shader property. Shader on the glass object then samples that blurred image, with UV coordinates offset based on a normal map to simulate refraction. This is similar to what shader GrabPass does does, except you can do more custom things (in this case, blurring).
渲染不透明的对象和天空盒之后,将当前图像复制到临时渲染目标中,进行模糊处理并将其设置为全局着色器属性。 然后,玻璃物体上的明暗器对模糊的图像进行采样,并根据法线贴图对UV坐标进行偏移以模拟折射。 这与着色器GrabPass所做的工作类似,不同之处在于您可以执行更多自定义操作(在这种情况下为模糊处理)。
Another example use case: custom deferred lights. Here are sphere-shaped and tube-shaped lights:
另一个示例用例: 自定义延迟的灯光 。 这是球形和管状的灯:
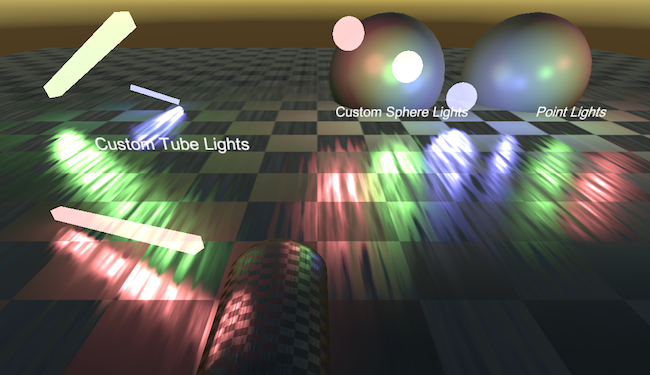
After regular deferred shading light pass is done, a sphere is drawn for each custom light, with a shader that computes illumination and adds it to the lighting buffer.
完成常规的延迟阴影光传递后,将为每个自定义光源绘制一个球体,并使用一个着色器来计算照明并将其添加到照明缓冲区中。
Yet another example: deferred decals.
又一个示例: 延期贴花 。
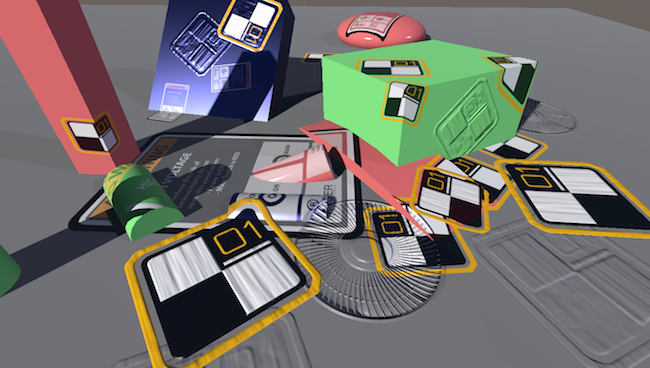
The idea is: after G-buffer is done, draw each “shape” of the decal (a box) and modify the G-buffer contents. This is very similar to how lights are done in deferred shading, except instead of accumulating the lighting we modify the G-buffer textures.
这个想法是:完成G缓冲区后,绘制贴花的每个“形状”(一个框)并修改G缓冲区的内容。 这与延迟着色中的光照非常相似,只是我们修改了G缓冲区纹理,而不是累积光照。
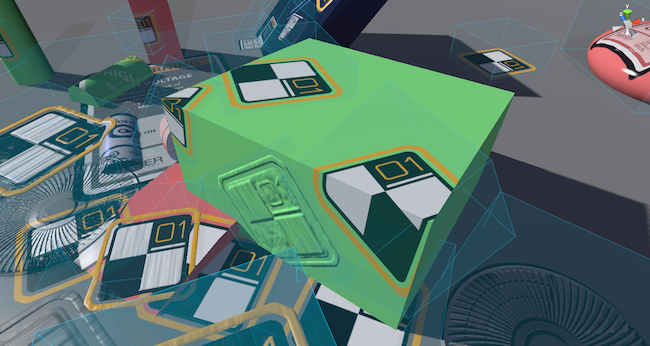
Each decal is implemented as a box here, and affects any geometry inside the box volume.
每个贴花都在此处实现为盒子,并且会影响盒子体积内的任何几何形状。
Actually, here’s a small Unity (5.0 beta 22) project folder that demonstrates everything above: RenderingCommandBuffers50b22.zip.
实际上,这是一个小的Unity(5.0 beta 22)项目文件夹,它演示了上面的所有内容: RenderingCommandBuffers50b22.zip 。
You can see that all the cases above aren’t even complex to implement – the scripts are about hundred lines of code.
您可以看到上述所有情况甚至都没有复杂实现–脚本大约有一百行代码。
I think this is exciting. Can’t wait to see what you all do with it!
我认为这很令人兴奋。 迫不及待想看看大家都在用它做什么!
翻译自: https://blogs.unity3d.com/2015/02/06/extending-unity-5-rendering-pipeline-command-buffers/
unity渲染管道