16、豌豆射手的资源导入及相关初始化

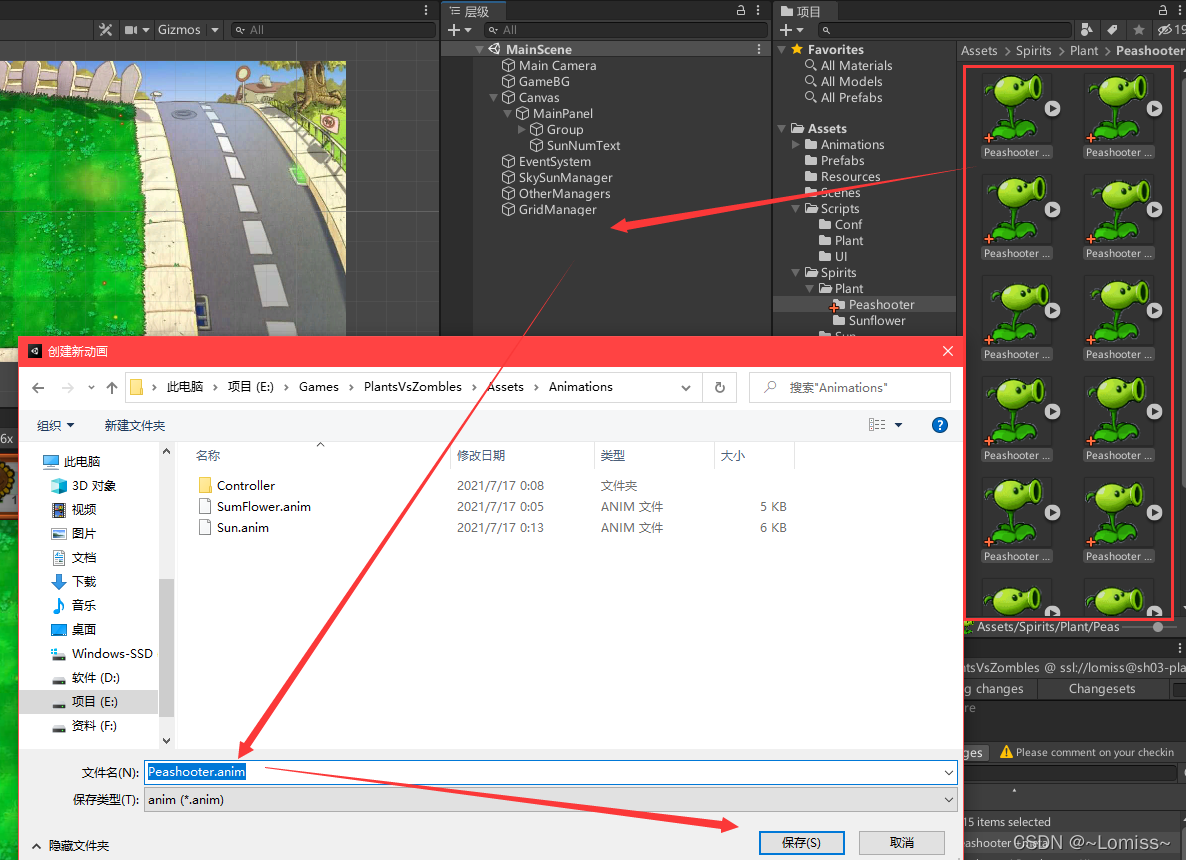
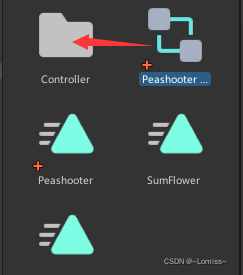
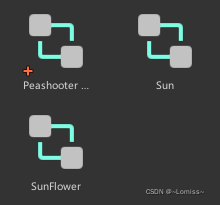
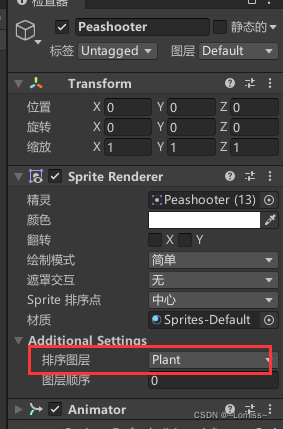
将其中一个卡片弄成豌豆射手(包括card和mask)
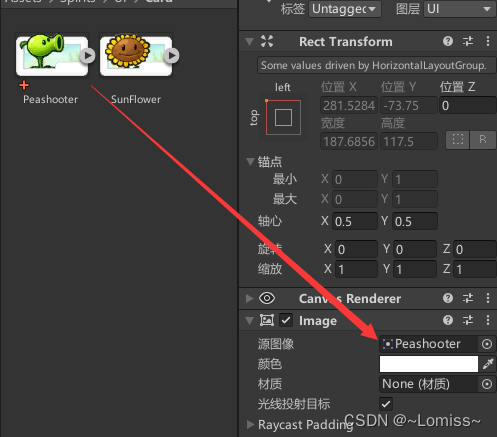
创建一个脚本并附加
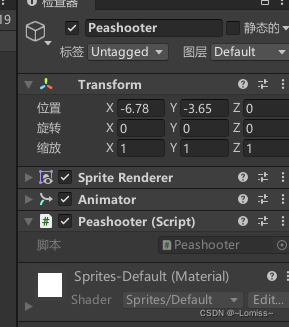
由于植物之间存在公共的部分,所以我们创建一个基类
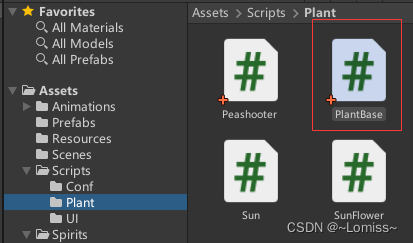
将SunFlower.cs中的一些公共代码搬到PlantBase.cs中去
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlantBase : MonoBehaviour
{
// 只能让子类调用
protected Animator animator;
protected SpriteRenderer spriteRenderer;
// 查找自身相关组件
protected void Find()
{
animator = GetComponent<Animator>();
spriteRenderer = GetComponent<SpriteRenderer>();
}
// 创建时的初始化
public void InitForCreate(bool inGrid)
{
// 获取组件
Find();
// 拖拽时不播放动画
animator.speed = 0;
if (inGrid)
{
spriteRenderer.sortingOrder = -1;
spriteRenderer.color = new Color(1, 1, 1, 0.6f);
}
}
// 放置植物的初始化
public void InitForPlace()
{
// 恢复动画
animator.speed = 1;
spriteRenderer.sortingOrder = 0;
OnInitForPlace();
}
// 创建一个虚基类
protected virtual void OnInitForPlace(){ }
}
此使SunFlower.cs的代码如下:
using System;
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class SunFlower : PlantBase
{
// 创建阳光所需要的时间
private float createSunTime = 5;
// 太阳花变红时所需要的时间
private float goldWantTime = 1.5f;
// 重载初始化放置函数,这里对于太阳花而言,需要每隔一段时间创建太阳花
protected override void OnInitForPlace()
{
InvokeRepeating("CreateSun", createSunTime, createSunTime);
}
// 创建阳光
private void CreateSun()
{
StartCoroutine(DoCreateSun());
}
IEnumerator DoCreateSun()
{
float currTime = 0;
currTime += 0.05f;
float lerp;
while (currTime < goldWantTime)
{
yield return new WaitForSeconds(0.05f);
lerp = currTime / goldWantTime;
currTime += 0.05f;
// 实现一个从白到红的插值计算,lerp为0就是白色(原色),如果为1就是Color(1,0.6f,0)
spriteRenderer.color = Color.Lerp(Color.white, new Color(1,0.6f,0),lerp);
}
// 恢复原来的附加色(白色)
spriteRenderer.color = Color.white;
// 生成的阳光父物体是太阳花本身
Sun sun = Instantiate(GameManager.instance.GameConf.Sun, transform.position, Quaternion.identity,
transform).GetComponent<Sun>();
// 生成阳光后跳跃
sun.JumpAnimation();
}
}
最后也让豌豆射手脚本继承这个植物基类
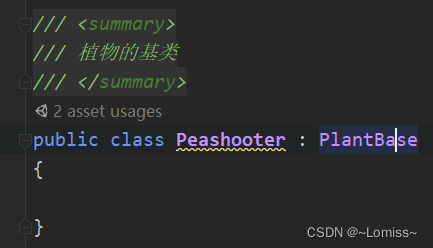
17、一些其余配置和bug修复
在GameConf中添加豌豆射手
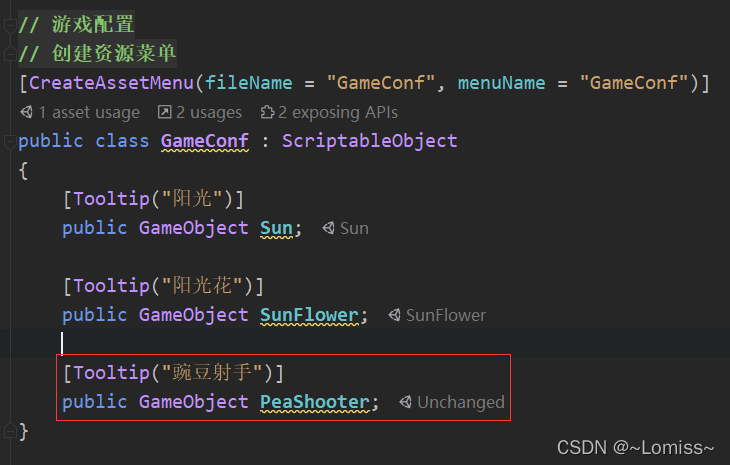
在植物类别上,将豌豆射手添加进去,在PlantManager.cs中添加代码
……
public enum PlantType
{
// 太阳花
SunFlower,
// 豌豆射手
Peashooter
}
public class PlantManager : MonoBehaviour
{
……
public GameObject GetPlantForType(PlantType type)
{
switch (type)
{
case PlantType.SunFlower:
return GameManager.instance.GameConf.SunFlower;
case PlantType.Peashooter:
return GameManager.instance.GameConf.PeaShooter;
}
return null;
}
}
最后在UI的植物卡片管理类UIPlantGrid.cs中更新代码:
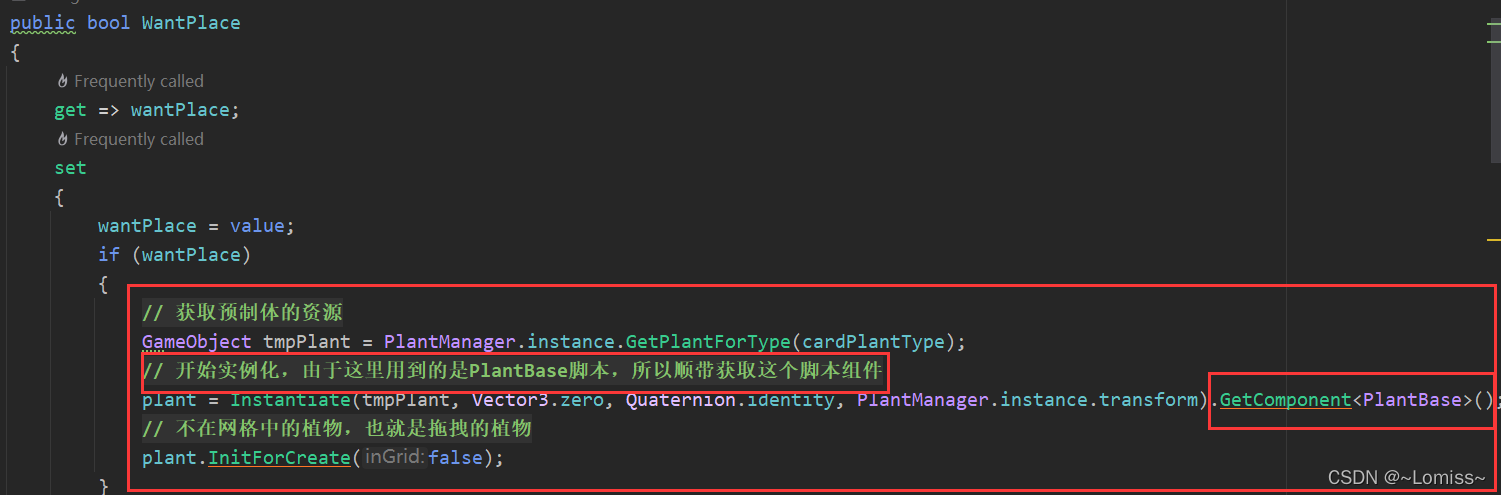
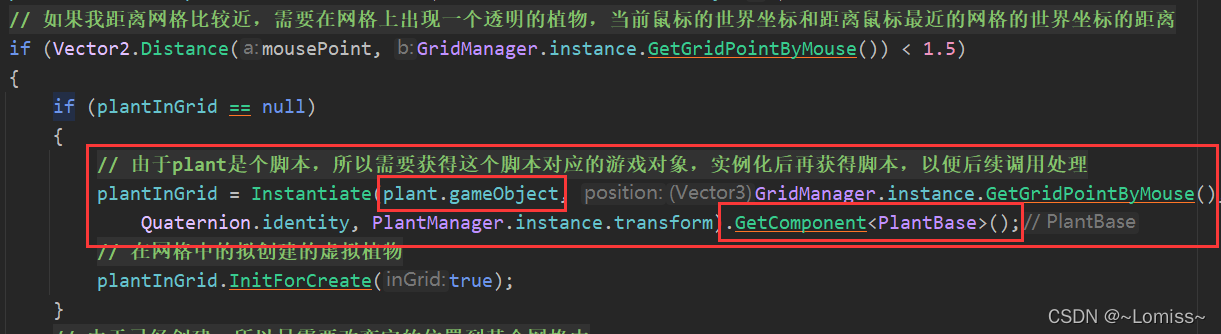
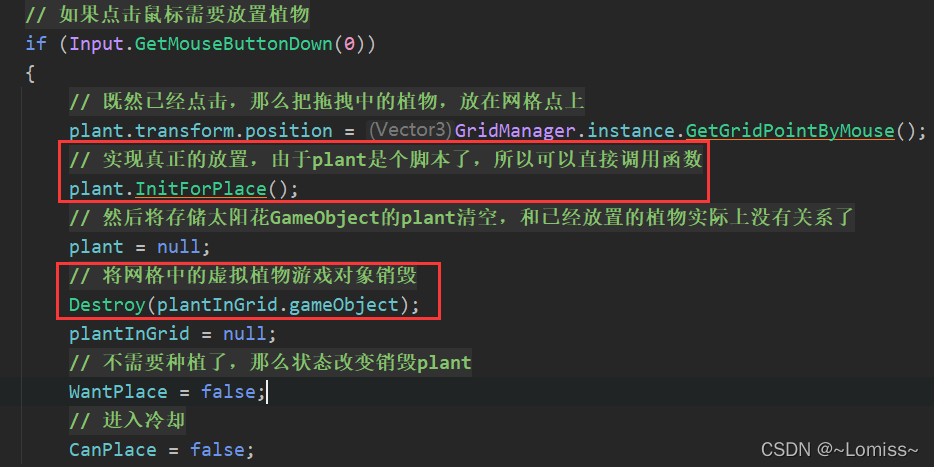
测试
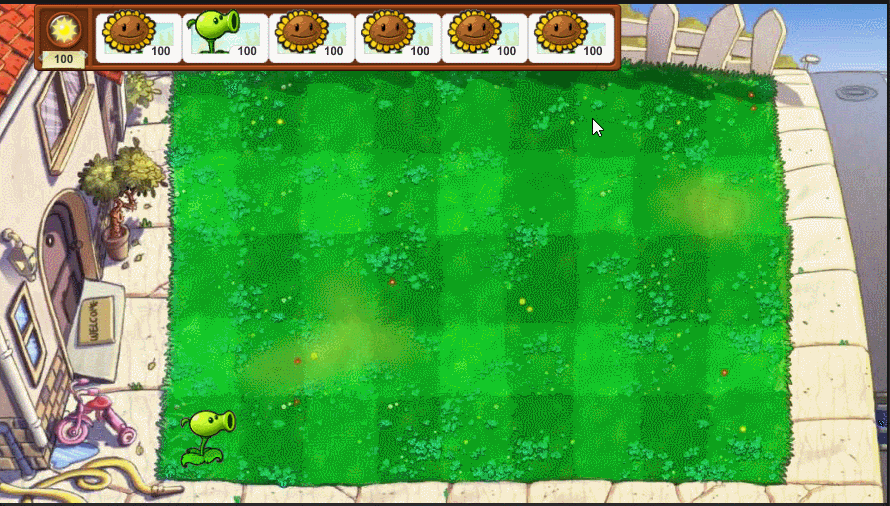
修复:同一个网格可以种植多个植物
解决:由于网格中有个hasPlant属性还没有用,所以在实例化虚拟植物的时候,就要把网格也要去出来,然后判断。在UIPlantGrid.cs中更新代码:
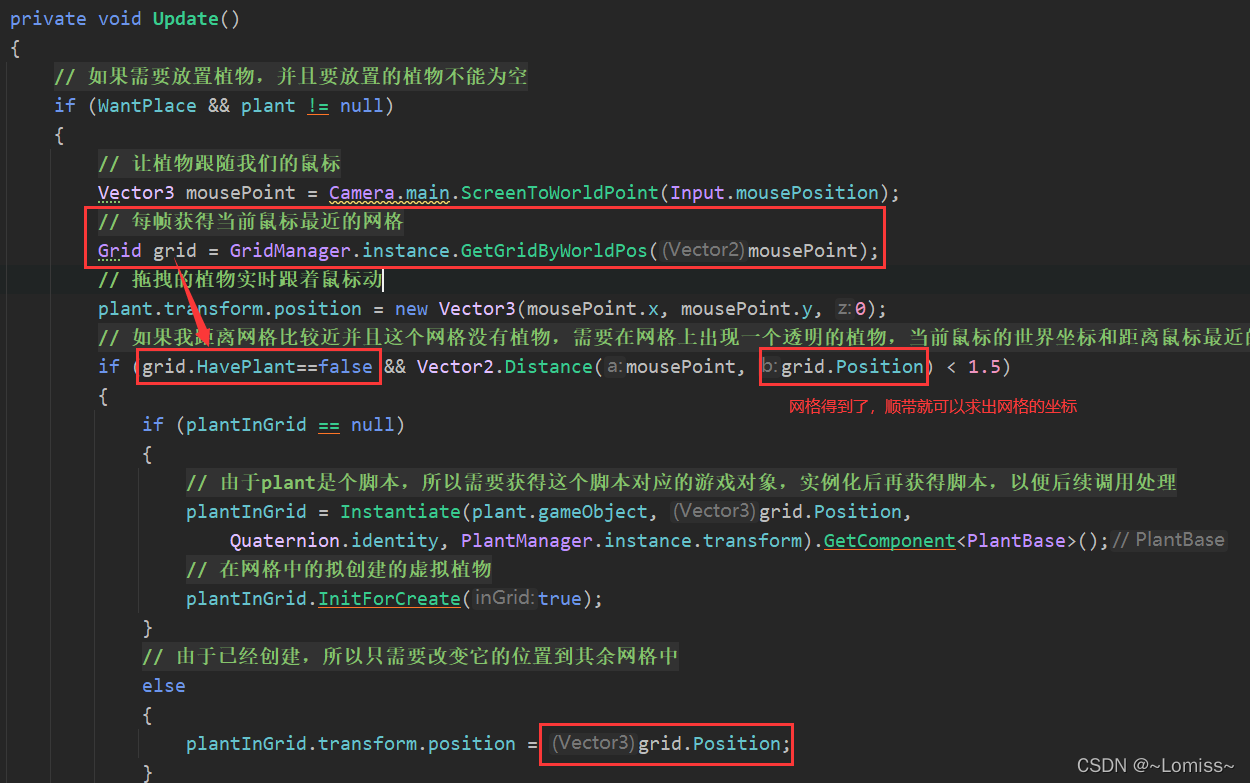
由于获取网格的函数没写,所以在GridManager.cs中更新代码
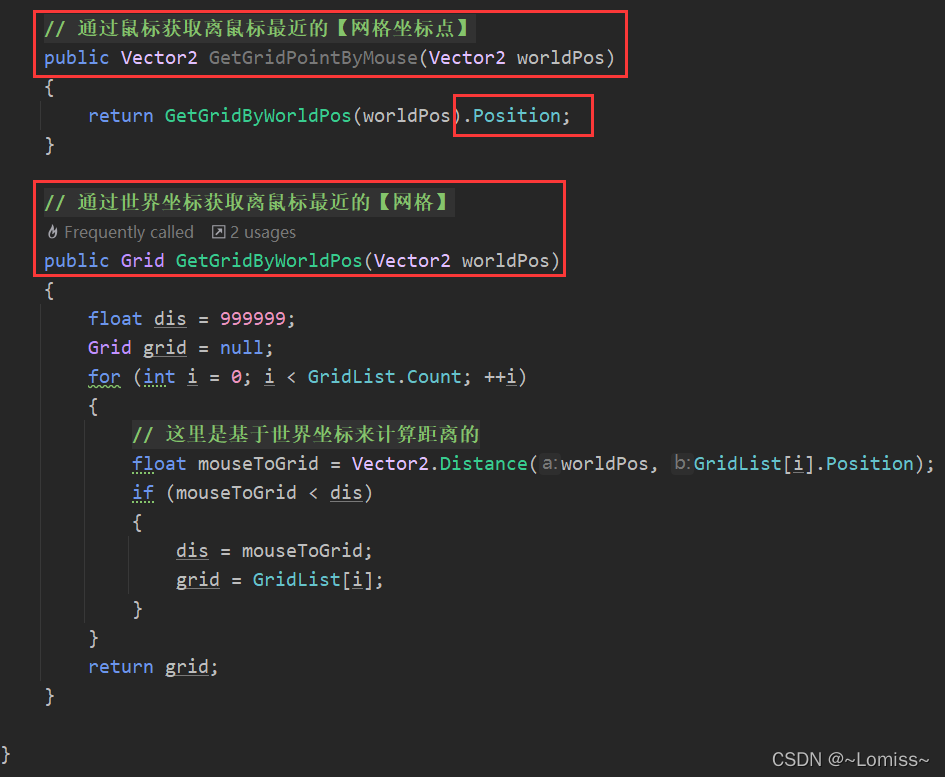
此使,如果网格里面有植物了,就不能够生成了。由于后续开发中涉及到僵尸会走到有植物的网格吃植物,所以也要在网格中新建一个属性,来获取当前网格的植物。
在Grid.cs中添加代码:
……
public class Grid
{
……
private PlantBase currPlantBase;
……
public PlantBase CurrPlantBase
{
get => currPlantBase;
set
{
currPlantBase = value;
if (currPlantBase == null)
{
HavePlant = false;
}
else
{
HavePlant = true;
}
}
}
}
在UIPlantGrid.cs中更新代码:
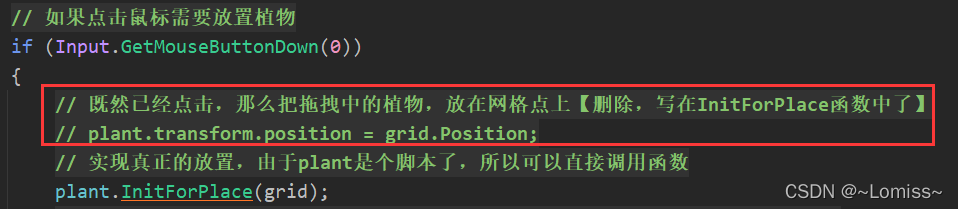
在PlantBase.cs中更新代码:
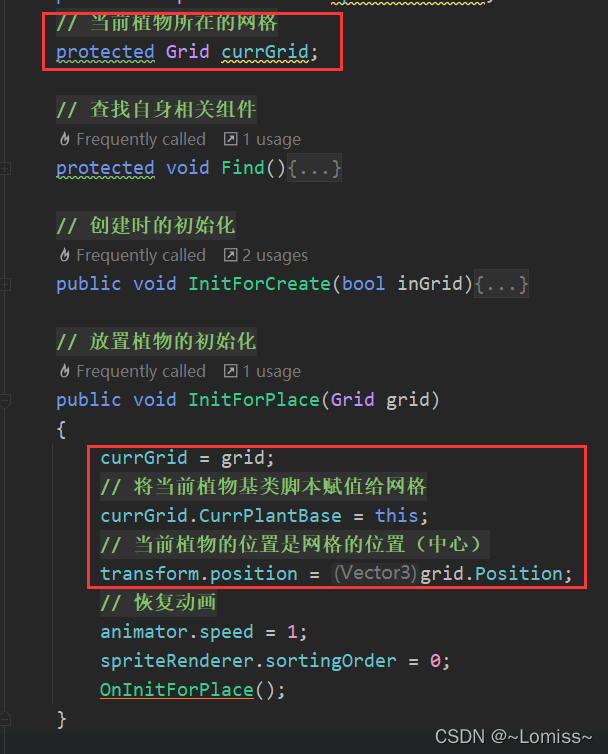
测试
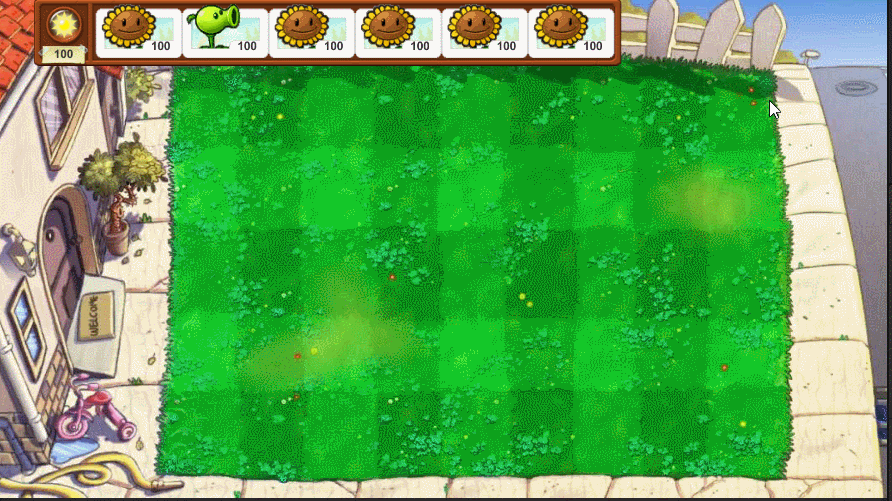