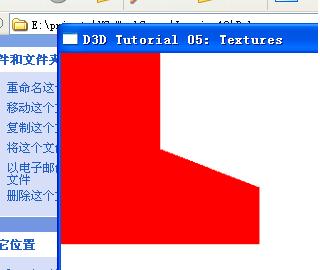
#include
<
Windows.h
>
#include < d3d9.h >
#include < d3dx9.h >
#pragma comment(lib, " d3d9.lib " )
#pragma comment(lib, " d3dx9.lib " )
#pragma comment(lib, " winmm.lib " )
LPDIRECT3D9 g_pd3d = 0 ;
LPDIRECT3DDEVICE9 g_pd3dDevice = 0 ;
LPDIRECT3DVERTEXBUFFER9 g_pVB = 0 ;
const int g_width = 1024 ;
const int g_height = 768 ;
struct CUSTOMVERTEX
{
FLOAT x, y, z, rhw;
DWORD color;
// CUSTOMVERTEX(FLOAT _x, FLOAT _y, FLOAT _z, FLOAT _rhw, DWORD _color)
// :x(_x)
// ,y(_y)
// ,z(_z)
// ,rhw(_rhw)
// ,color(_color){}
};
#define D3DFVF_CUSTOMVERTEX (D3DFVF_XYZRHW | D3DFVF_DIFFUSE)
HRESULT InitD3D(HWND hwnd)
{
if (NULL == (g_pd3d = Direct3DCreate9(D3D_SDK_VERSION)))
{
MessageBox(NULL, " Create D3D Failed! " , " Lession13 " , MB_OK);
return E_FAIL;
}
D3DCAPS9 caps;
int vp = 0 ;
g_pd3d -> GetDeviceCaps(D3DADAPTER_DEFAULT, D3DDEVTYPE_HAL, & caps);
if (caps.DevCaps & D3DDEVCAPS_HWTRANSFORMANDLIGHT)
vp = D3DCREATE_HARDWARE_VERTEXPROCESSING;
else
vp = D3DCREATE_SOFTWARE_VERTEXPROCESSING;
D3DPRESENT_PARAMETERS d3dpp;
::ZeroMemory( & d3dpp, sizeof (d3dpp));
d3dpp.AutoDepthStencilFormat = D3DFMT_D24S8;
d3dpp.BackBufferCount = 1 ;
d3dpp.BackBufferFormat = D3DFMT_A8R8G8B8;
d3dpp.BackBufferHeight = g_height;
d3dpp.BackBufferWidth = g_width;
d3dpp.EnableAutoDepthStencil = true ;
d3dpp.Flags = 0 ;
d3dpp.FullScreen_RefreshRateInHz = D3DPRESENT_RATE_DEFAULT;
d3dpp.hDeviceWindow = hwnd;
d3dpp.MultiSampleQuality = 0 ;
d3dpp.MultiSampleType = D3DMULTISAMPLE_NONE;
d3dpp.PresentationInterval = D3DPRESENT_INTERVAL_IMMEDIATE;
d3dpp.SwapEffect = D3DSWAPEFFECT_DISCARD;
d3dpp.Windowed = true ;
if (FAILED(g_pd3d -> CreateDevice(D3DADAPTER_DEFAULT, D3DDEVTYPE_HAL, hwnd, vp, & d3dpp, & g_pd3dDevice)))
{
MessageBox(NULL, " Create Device Failed! " , " Lession13 " , MB_OK);
return E_FAIL;
}
// D3DXMATRIX matProj;
// D3DXMatrixIdentity(&matProj);
// D3DXMatrixPerspectiveFovLH(&matProj, D3DX_PI/4, 1, 1, 1000);
// g_pd3dDevice->SetRenderState(D3DRS_FILLMODE, D3DFILL_SOLID);
return S_OK;
}
HRESULT InitVB(FLOAT angle, LPRECT rt, DWORD dwColor)
{
// float angle = (360*0.01)*nPercent;
int nArea = angle / 45 ;
int nTriangles = nArea + 1 ;
int nPoints = nArea + 2 ;
// 规定中心点
int ndivX = (rt -> right - rt -> left) >> 1 ;
int ndivY = (rt -> bottom - rt -> top) >> 1 ;
// 默认的赋值
CUSTOMVERTEX pVerteics[] =
{
{ndivX, ndivY, 0 , 0 .f, dwColor}, // 1
{ndivX, rt -> top, 0 , 0 .f, dwColor}, // 2
{rt -> left, rt -> top, 0 , 0 .f, dwColor}, // 3
{rt -> left, ndivY, 0 , 0 .f, dwColor}, // 4
{rt -> left, rt -> bottom, 0 , 0 .f, dwColor}, // 5
{ndivX, rt -> bottom, 0 , 0 .f, dwColor}, // 6
{rt -> right, rt -> bottom, 0 , 0 .f, dwColor}, // 7
{rt -> right, ndivY, 0 , 0 .f, dwColor}, // 8
{rt -> right, rt -> top, 0 , 0 .f, dwColor}, // 9
{ndivX, rt -> top, 0 , 0 .f, dwColor}, // 2
};
int leave_angle = ( int )angle % 45 ;
if ( ! leave_angle)
{
nTriangles -= 1 ;
}
// 计算此时秒针的向量
D3DXVECTOR2 v = * (D3DXVECTOR2 * )(pVerteics + nTriangles);
D3DXVECTOR2 v2 = * (D3DXVECTOR2 * )(pVerteics + nPoints);
D3DXVECTOR2 nor = * (D3DXVECTOR2 * )(pVerteics);
D3DXVECTOR2 vResult;
if ((angle != 45 ) || (angle != 225 ))
{
D3DXVECTOR2 vDummy1 = v - nor;
D3DXVECTOR2 vDummy2 = v2 - nor;
// D3DXVec2Lerp(&vResult, &vDummy1, &vDummy2, leave_angle);
switch (nArea)
{
case 0 :
vResult.y = 0 ;
vResult.x = ndivX - ndivY * tanf((leave_angle) * D3DX_PI / 180 );
break ;
case 1 :
vResult.x = rt -> left;
vResult.y = ndivY - ndivX * tanf(( 45 - leave_angle) * D3DX_PI / 180 );
break ;
case 2 :
vResult.x = rt -> left;
vResult.y = ndivY + ndivX * tanf((leave_angle) * D3DX_PI / 180 );
break ;
case 3 :
vResult.y = rt -> bottom;
vResult.x = ndivX - ndivY * tanf(( 45 - leave_angle) * D3DX_PI / 180 );
break ;
case 4 :
vResult.y = rt -> bottom;
vResult.x = ndivX + ndivY * tanf((leave_angle) * D3DX_PI / 180 );
break ;
case 5 :
vResult.x = rt -> right;
vResult.y = ndivY + ndivX * tanf(( 45 - leave_angle) * D3DX_PI / 180 );
break ;
case 6 :
vResult.x = rt -> right;
vResult.y = ndivY - ndivX * tanf((leave_angle) * D3DX_PI / 180 );
break ;
case 7 :
vResult.x = ndivX + ndivY * tanf(( 45 - leave_angle) * D3DX_PI / 180 );
vResult.y = rt -> top;
break ;
}
* (D3DXVECTOR2 * )(pVerteics + nPoints) = vResult;
}
else
{
D3DXVec2Lerp( & vResult, & v, & v2, leave_angle / 45 );
* (D3DXVECTOR2 * )(pVerteics + nPoints) = vResult;
}
// g_pd3dDevice->CreateVertexBuffer((nPoints+1)*sizeof(CUSTOMVERTEX), D3DUSAGE_WRITEONLY, D3DFVF_CUSTOMVERTEX, D3DPOOL_MANAGED, &g_pVB, 0);
// void * pV;
// if (SUCCEEDED(g_pVB->Lock(0, 0, (void **)&pV, 0)))
// {
// memcpy(pV, pVerteics, (nPoints+1)*sizeof(CUSTOMVERTEX));
// g_pVB->Unlock();
// }
g_pd3dDevice -> SetRenderState(D3DRS_CULLMODE, D3DCULL_NONE);
g_pd3dDevice -> SetRenderState(D3DRS_LIGHTING, FALSE);
g_pd3dDevice -> Clear( 0 , NULL, D3DCLEAR_TARGET | D3DCLEAR_ZBUFFER, 0xffffffff , 1 .f, 0 );
g_pd3dDevice -> BeginScene();
// g_pd3dDevice->SetStreamSource(0, g_pVB, 0, sizeof(CUSTOMVERTEX));
g_pd3dDevice -> SetFVF(D3DFVF_CUSTOMVERTEX);
// g_pd3dDevice->DrawPrimitive(D3DPT_TRIANGLEFAN, nTriangles, nPoints);
g_pd3dDevice -> DrawPrimitiveUP(D3DPT_TRIANGLEFAN,nTriangles,pVerteics, sizeof (CUSTOMVERTEX));
g_pd3dDevice -> EndScene();
g_pd3dDevice -> Present( 0 , 0 , 0 , 0 );
return S_OK;
}
VOID Cleanup()
{
if (g_pVB)
{
g_pVB -> Release();
g_pVB = 0 ;
}
if (g_pd3dDevice)
{
g_pd3dDevice -> Release();
g_pd3dDevice = 0 ;
}
if (g_pd3d)
{
g_pd3d -> Release();
g_pd3d = 0 ;
}
}
int timeCounter = 0 ;
LRESULT WINAPI MsgProc( HWND hWnd, UINT msg, WPARAM wParam, LPARAM lParam )
{
switch ( msg )
{
case WM_CREATE:
SetTimer(hWnd, 1 , 25 , NULL);
return 0 ;
case WM_DESTROY:
Cleanup();
PostQuitMessage( 0 );
return 0 ;
case WM_TIMER:
{
timeCounter ++ ;
timeCounter %= 360 ;
RECT rt = { 0 , 0 , 200 , 200 };
InitVB( 360 - timeCounter, & rt, 0xffff0000 );
}
return 0 ;
}
return DefWindowProc( hWnd, msg, wParam, lParam );
}
// -----------------------------------------------------------------------------
// Name: WinMain()
// Desc: The application's entry point
// -----------------------------------------------------------------------------
INT WINAPI WinMain( HINSTANCE hInst, HINSTANCE, LPSTR, INT )
{
// Register the window class
WNDCLASSEX wc = { sizeof (WNDCLASSEX), CS_CLASSDC, MsgProc, 0L , 0L ,
GetModuleHandle(NULL), NULL, NULL, NULL, NULL,
" D3D Tutorial " , NULL };
RegisterClassEx( & wc );
// Create the application's window
HWND hWnd = CreateWindow( " D3D Tutorial " , " D3D Tutorial 05: Textures " ,
WS_OVERLAPPEDWINDOW, 100 , 100 , g_width, g_height,
NULL, NULL, wc.hInstance, NULL );
// Initialize Direct3D
if ( SUCCEEDED( InitD3D( hWnd ) ) )
{
/// / Create the scene geometry
// if( SUCCEEDED( InitVB() ) )
// {
// Show the window
ShowWindow( hWnd, SW_SHOWDEFAULT );
UpdateWindow( hWnd );
// Enter the message loop
MSG msg;
ZeroMemory( & msg, sizeof (msg) );
while ( msg.message != WM_QUIT )
{
if ( PeekMessage( & msg, NULL, 0U , 0U , PM_REMOVE ) )
{
TranslateMessage( & msg );
DispatchMessage( & msg );
}
else
{
}
}
// }
}
UnregisterClass( " D3D Tutorial " , wc.hInstance );
return 0 ;
}
#include < d3d9.h >
#include < d3dx9.h >
#pragma comment(lib, " d3d9.lib " )
#pragma comment(lib, " d3dx9.lib " )
#pragma comment(lib, " winmm.lib " )
LPDIRECT3D9 g_pd3d = 0 ;
LPDIRECT3DDEVICE9 g_pd3dDevice = 0 ;
LPDIRECT3DVERTEXBUFFER9 g_pVB = 0 ;
const int g_width = 1024 ;
const int g_height = 768 ;
struct CUSTOMVERTEX
{
FLOAT x, y, z, rhw;
DWORD color;
// CUSTOMVERTEX(FLOAT _x, FLOAT _y, FLOAT _z, FLOAT _rhw, DWORD _color)
// :x(_x)
// ,y(_y)
// ,z(_z)
// ,rhw(_rhw)
// ,color(_color){}
};
#define D3DFVF_CUSTOMVERTEX (D3DFVF_XYZRHW | D3DFVF_DIFFUSE)
HRESULT InitD3D(HWND hwnd)
{
if (NULL == (g_pd3d = Direct3DCreate9(D3D_SDK_VERSION)))
{
MessageBox(NULL, " Create D3D Failed! " , " Lession13 " , MB_OK);
return E_FAIL;
}
D3DCAPS9 caps;
int vp = 0 ;
g_pd3d -> GetDeviceCaps(D3DADAPTER_DEFAULT, D3DDEVTYPE_HAL, & caps);
if (caps.DevCaps & D3DDEVCAPS_HWTRANSFORMANDLIGHT)
vp = D3DCREATE_HARDWARE_VERTEXPROCESSING;
else
vp = D3DCREATE_SOFTWARE_VERTEXPROCESSING;
D3DPRESENT_PARAMETERS d3dpp;
::ZeroMemory( & d3dpp, sizeof (d3dpp));
d3dpp.AutoDepthStencilFormat = D3DFMT_D24S8;
d3dpp.BackBufferCount = 1 ;
d3dpp.BackBufferFormat = D3DFMT_A8R8G8B8;
d3dpp.BackBufferHeight = g_height;
d3dpp.BackBufferWidth = g_width;
d3dpp.EnableAutoDepthStencil = true ;
d3dpp.Flags = 0 ;
d3dpp.FullScreen_RefreshRateInHz = D3DPRESENT_RATE_DEFAULT;
d3dpp.hDeviceWindow = hwnd;
d3dpp.MultiSampleQuality = 0 ;
d3dpp.MultiSampleType = D3DMULTISAMPLE_NONE;
d3dpp.PresentationInterval = D3DPRESENT_INTERVAL_IMMEDIATE;
d3dpp.SwapEffect = D3DSWAPEFFECT_DISCARD;
d3dpp.Windowed = true ;
if (FAILED(g_pd3d -> CreateDevice(D3DADAPTER_DEFAULT, D3DDEVTYPE_HAL, hwnd, vp, & d3dpp, & g_pd3dDevice)))
{
MessageBox(NULL, " Create Device Failed! " , " Lession13 " , MB_OK);
return E_FAIL;
}
// D3DXMATRIX matProj;
// D3DXMatrixIdentity(&matProj);
// D3DXMatrixPerspectiveFovLH(&matProj, D3DX_PI/4, 1, 1, 1000);
// g_pd3dDevice->SetRenderState(D3DRS_FILLMODE, D3DFILL_SOLID);
return S_OK;
}
HRESULT InitVB(FLOAT angle, LPRECT rt, DWORD dwColor)
{
// float angle = (360*0.01)*nPercent;
int nArea = angle / 45 ;
int nTriangles = nArea + 1 ;
int nPoints = nArea + 2 ;
// 规定中心点
int ndivX = (rt -> right - rt -> left) >> 1 ;
int ndivY = (rt -> bottom - rt -> top) >> 1 ;
// 默认的赋值
CUSTOMVERTEX pVerteics[] =
{
{ndivX, ndivY, 0 , 0 .f, dwColor}, // 1
{ndivX, rt -> top, 0 , 0 .f, dwColor}, // 2
{rt -> left, rt -> top, 0 , 0 .f, dwColor}, // 3
{rt -> left, ndivY, 0 , 0 .f, dwColor}, // 4
{rt -> left, rt -> bottom, 0 , 0 .f, dwColor}, // 5
{ndivX, rt -> bottom, 0 , 0 .f, dwColor}, // 6
{rt -> right, rt -> bottom, 0 , 0 .f, dwColor}, // 7
{rt -> right, ndivY, 0 , 0 .f, dwColor}, // 8
{rt -> right, rt -> top, 0 , 0 .f, dwColor}, // 9
{ndivX, rt -> top, 0 , 0 .f, dwColor}, // 2
};
int leave_angle = ( int )angle % 45 ;
if ( ! leave_angle)
{
nTriangles -= 1 ;
}
// 计算此时秒针的向量
D3DXVECTOR2 v = * (D3DXVECTOR2 * )(pVerteics + nTriangles);
D3DXVECTOR2 v2 = * (D3DXVECTOR2 * )(pVerteics + nPoints);
D3DXVECTOR2 nor = * (D3DXVECTOR2 * )(pVerteics);
D3DXVECTOR2 vResult;
if ((angle != 45 ) || (angle != 225 ))
{
D3DXVECTOR2 vDummy1 = v - nor;
D3DXVECTOR2 vDummy2 = v2 - nor;
// D3DXVec2Lerp(&vResult, &vDummy1, &vDummy2, leave_angle);
switch (nArea)
{
case 0 :
vResult.y = 0 ;
vResult.x = ndivX - ndivY * tanf((leave_angle) * D3DX_PI / 180 );
break ;
case 1 :
vResult.x = rt -> left;
vResult.y = ndivY - ndivX * tanf(( 45 - leave_angle) * D3DX_PI / 180 );
break ;
case 2 :
vResult.x = rt -> left;
vResult.y = ndivY + ndivX * tanf((leave_angle) * D3DX_PI / 180 );
break ;
case 3 :
vResult.y = rt -> bottom;
vResult.x = ndivX - ndivY * tanf(( 45 - leave_angle) * D3DX_PI / 180 );
break ;
case 4 :
vResult.y = rt -> bottom;
vResult.x = ndivX + ndivY * tanf((leave_angle) * D3DX_PI / 180 );
break ;
case 5 :
vResult.x = rt -> right;
vResult.y = ndivY + ndivX * tanf(( 45 - leave_angle) * D3DX_PI / 180 );
break ;
case 6 :
vResult.x = rt -> right;
vResult.y = ndivY - ndivX * tanf((leave_angle) * D3DX_PI / 180 );
break ;
case 7 :
vResult.x = ndivX + ndivY * tanf(( 45 - leave_angle) * D3DX_PI / 180 );
vResult.y = rt -> top;
break ;
}
* (D3DXVECTOR2 * )(pVerteics + nPoints) = vResult;
}
else
{
D3DXVec2Lerp( & vResult, & v, & v2, leave_angle / 45 );
* (D3DXVECTOR2 * )(pVerteics + nPoints) = vResult;
}
// g_pd3dDevice->CreateVertexBuffer((nPoints+1)*sizeof(CUSTOMVERTEX), D3DUSAGE_WRITEONLY, D3DFVF_CUSTOMVERTEX, D3DPOOL_MANAGED, &g_pVB, 0);
// void * pV;
// if (SUCCEEDED(g_pVB->Lock(0, 0, (void **)&pV, 0)))
// {
// memcpy(pV, pVerteics, (nPoints+1)*sizeof(CUSTOMVERTEX));
// g_pVB->Unlock();
// }
g_pd3dDevice -> SetRenderState(D3DRS_CULLMODE, D3DCULL_NONE);
g_pd3dDevice -> SetRenderState(D3DRS_LIGHTING, FALSE);
g_pd3dDevice -> Clear( 0 , NULL, D3DCLEAR_TARGET | D3DCLEAR_ZBUFFER, 0xffffffff , 1 .f, 0 );
g_pd3dDevice -> BeginScene();
// g_pd3dDevice->SetStreamSource(0, g_pVB, 0, sizeof(CUSTOMVERTEX));
g_pd3dDevice -> SetFVF(D3DFVF_CUSTOMVERTEX);
// g_pd3dDevice->DrawPrimitive(D3DPT_TRIANGLEFAN, nTriangles, nPoints);
g_pd3dDevice -> DrawPrimitiveUP(D3DPT_TRIANGLEFAN,nTriangles,pVerteics, sizeof (CUSTOMVERTEX));
g_pd3dDevice -> EndScene();
g_pd3dDevice -> Present( 0 , 0 , 0 , 0 );
return S_OK;
}
VOID Cleanup()
{
if (g_pVB)
{
g_pVB -> Release();
g_pVB = 0 ;
}
if (g_pd3dDevice)
{
g_pd3dDevice -> Release();
g_pd3dDevice = 0 ;
}
if (g_pd3d)
{
g_pd3d -> Release();
g_pd3d = 0 ;
}
}
int timeCounter = 0 ;
LRESULT WINAPI MsgProc( HWND hWnd, UINT msg, WPARAM wParam, LPARAM lParam )
{
switch ( msg )
{
case WM_CREATE:
SetTimer(hWnd, 1 , 25 , NULL);
return 0 ;
case WM_DESTROY:
Cleanup();
PostQuitMessage( 0 );
return 0 ;
case WM_TIMER:
{
timeCounter ++ ;
timeCounter %= 360 ;
RECT rt = { 0 , 0 , 200 , 200 };
InitVB( 360 - timeCounter, & rt, 0xffff0000 );
}
return 0 ;
}
return DefWindowProc( hWnd, msg, wParam, lParam );
}
// -----------------------------------------------------------------------------
// Name: WinMain()
// Desc: The application's entry point
// -----------------------------------------------------------------------------
INT WINAPI WinMain( HINSTANCE hInst, HINSTANCE, LPSTR, INT )
{
// Register the window class
WNDCLASSEX wc = { sizeof (WNDCLASSEX), CS_CLASSDC, MsgProc, 0L , 0L ,
GetModuleHandle(NULL), NULL, NULL, NULL, NULL,
" D3D Tutorial " , NULL };
RegisterClassEx( & wc );
// Create the application's window
HWND hWnd = CreateWindow( " D3D Tutorial " , " D3D Tutorial 05: Textures " ,
WS_OVERLAPPEDWINDOW, 100 , 100 , g_width, g_height,
NULL, NULL, wc.hInstance, NULL );
// Initialize Direct3D
if ( SUCCEEDED( InitD3D( hWnd ) ) )
{
/// / Create the scene geometry
// if( SUCCEEDED( InitVB() ) )
// {
// Show the window
ShowWindow( hWnd, SW_SHOWDEFAULT );
UpdateWindow( hWnd );
// Enter the message loop
MSG msg;
ZeroMemory( & msg, sizeof (msg) );
while ( msg.message != WM_QUIT )
{
if ( PeekMessage( & msg, NULL, 0U , 0U , PM_REMOVE ) )
{
TranslateMessage( & msg );
DispatchMessage( & msg );
}
else
{
}
}
// }
}
UnregisterClass( " D3D Tutorial " , wc.hInstance );
return 0 ;
}