工程结构
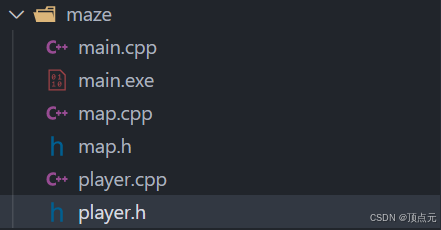
map
map.h
#ifndef __MAP_H
#define __MAP_H
#define WALL 0
#define ROAD 1
#define MAX_HEIGHT 30
#define MAX_WIDTH 30
typedef struct Point {
int x;
int y;
}Point_t;
class Map {
public:
Map();
Map(int*map, int row, int column, Point_t in);
~Map();
void draw(void);
void setRoadChar(char r);
char getRoadChar(void);
void setWallChar(char w);
bool checkWallOrNot(int x, int y);
int getHeight(void);
int getWidth(void);
private:
char road_;
char wall_;
int map_[MAX_HEIGHT][MAX_WIDTH];
int height_;
int width_;
Point_t in_;
};
#endif
map.cpp
#include "map.h"
#include <iostream>
using namespace std;
Map::Map() : wall_('$'), road_(' ') {
height_ = 5;
width_ = 10;
int default_map[5][10] = {
WALL, WALL, ROAD, WALL, WALL, WALL, WALL, ROAD, WALL, ROAD,
ROAD, WALL, ROAD, WALL, WALL, WALL, WALL, ROAD, WALL, WALL,
WALL, WALL, WALL, ROAD, ROAD, ROAD, WALL, ROAD, WALL, ROAD,
WALL, ROAD, ROAD, ROAD, WALL, ROAD, ROAD, ROAD, WALL, WALL,
WALL, ROAD, WALL, WALL, WALL, WALL, WALL, WALL, WALL, WALL
};
for(int i = 0; i