函数积分图
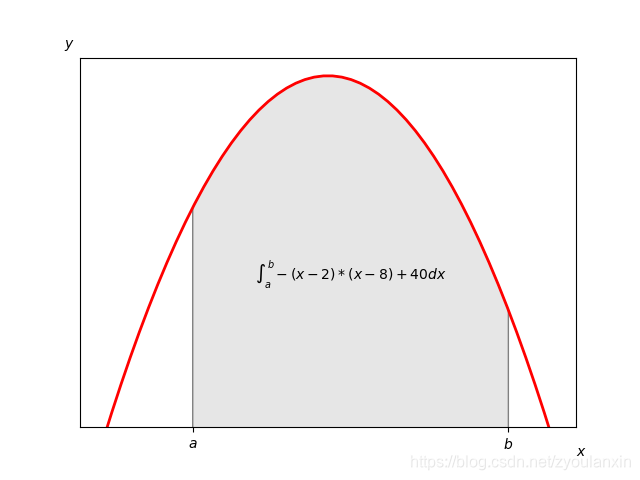
import numpy as np
import matplotlib.pyplot as plt
import matplotlib as mpl
from matplotlib.patches import Polygon
def func(x):
return -(x-2)*(x-8) + 40
x = np.linspace(0,10)
y = func(x)
fig,ax = plt.subplots()
plt.plot(x,y,"r",linewidth=2)
a = 2
b = 9
ax.set_xticks([a,b])
ax.set_yticks([])
ax.set_xticklabels([r"$a$",r"$b$"])
plt.figtext(0.9,0.05,r"$x$")
plt.figtext(0.1,0.9,r"$y$")
ix = np.linspace(a,b)
iy = func(ix)
ixy = zip(ix,iy)
verts = [(a,0)]+list(ixy)+[(b,0)]
print(verts)
poly =Polygon(verts,facecolor="0.9",edgecolor="0.5")
ax.add_patch(poly)
x_math = (a+b)/2
y_math = 35
plt.text(x_math,y_math,r"$\int_a^b -(x-2)*(x-8) + 40dx$",fontsize=10,
horizontalalignment="center")
plt.ylim(25)
plt.show()
散点-条形图
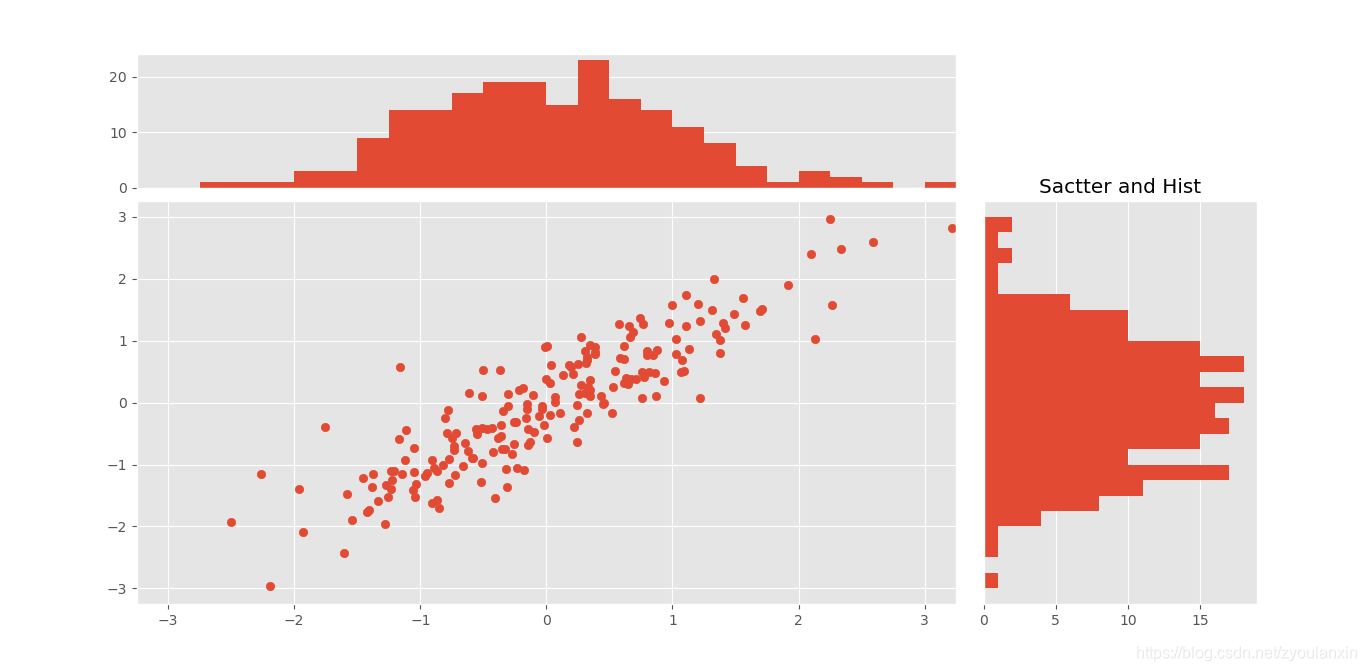
import numpy as np
import matplotlib.pyplot as plt
plt.style.use("ggplot")
x = np.random.randn(200)
y = x + np.random.randn(200)*0.5
margin_border = 0.1
width = 0.6
margin_between = 0.02
height = 0.2
left_s = margin_border
bottom_s = margin_border
height_s = width
width_s = width
left_x = margin_border
bottom_x = margin_border + width + margin_between
height_x = height
width_x = width
left_y = margin_border + width + margin_between
bottom_y = margin_border
height_y = width
width_y = height
plt.figure(figsize=(8,8))
rect_s = [left_s,bottom_s,width_s,height_s]
rect_x = [left_x,bottom_x,width_x,height_x]
rect_y = [left_y,bottom_y,width_y,height_y]
axScatter = plt.axes(rect_s)
axHistX = plt.axes(rect_x)
axHistY = plt.axes(rect_y)
axHistX.set_xticks([])
axHistY.set_yticks([])
axScatter.scatter(x,y)
bin_width = 0.25
xymax = np.max([np.max(np.fabs(x)),np.max(np.fabs(y))])
lim = int(xymax/bin_width+1)*bin_width
axScatter.set_xlim(-lim,lim)
axScatter.set_ylim(-lim,lim)
bins = np.arange(-lim,lim+width,bin_width)
axHistX.hist(x,bins=bins)
axHistY.hist(y,bins=bins,orientation = "horizontal")
print(xymax)
axHistX.set_xlim(axScatter.get_xlim())
axHistY.set_ylim(axScatter.get_ylim())
plt.title("Sactter and Hist")
plt.show()
球员能力图
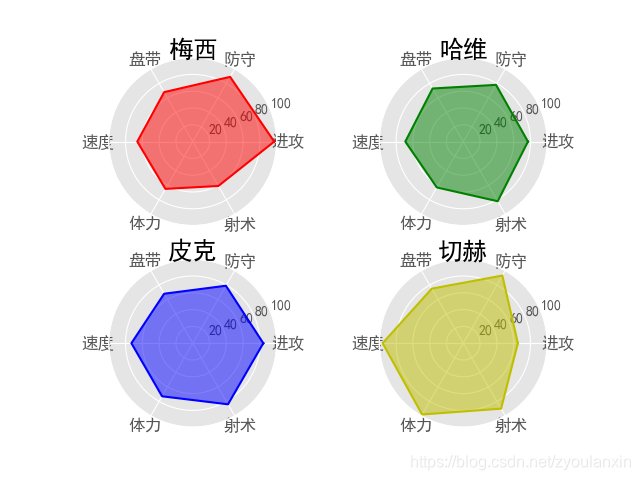
import numpy as np
import matplotlib.pyplot as plt
plt.rcParams['font.sans-serif']=['SimHei']
plt.rcParams['axes.unicode_minus']=False
plt.style.use("ggplot")
ability_size = 6
ability_label = [u"进攻",u"防守",u"盘带",u"速度",u"体力",u"射术"]
ax1 = plt.subplot(221,projection = "polar")
ax2 = plt.subplot(222,projection = "polar")
ax3 = plt.subplot(223,projection = "polar")
ax4 = plt.subplot(224,projection = "polar")
player = {
"M": np.random.randint(size=ability_size,low=60, high=99),
"H": np.random.randint(size=ability_size, low=60, high=99),
"P": np.random.randint(size=ability_size, low=60, high=99),
"Q": np.random.randint(size=ability_size, low=60, high=99)
}
theta = np.linspace(0,2*np.pi,6,endpoint=False)
theta = np.append(theta,theta[0])
player["M"] = np.append(player["M"],player["M"][0])
ax1.plot(theta,player["M"],"r")
ax1.fill(theta,player["M"],"r",alpha=0.5)
ax1.set_xticks(theta)
ax1.set_xticklabels(ability_label,y=0.1,size=12)
ax1.set_title(u"梅西",size=18,position = (0.5,0.95))
ax1.set_yticks([20,40,60,80,100])
player["H"] = np.append(player["H"],player["H"][0])
ax2.plot(theta,player["H"],"g")
ax2.fill(theta,player["H"],"g",alpha=0.5)
ax2.set_xticks(theta)
ax2.set_xticklabels(ability_label,y=0.1,size=12)
ax2.set_title(u"哈维",size=18,position = (0.5,0.95))
ax2.set_yticks([20,40,60,80,100])
player["P"] = np.append(player["P"],player["P"][0])
ax3.plot(theta,player["P"],"b")
ax3.fill(theta,player["P"],"b",alpha=0.5)
ax3.set_xticks(theta)
ax3.set_xticklabels(ability_label,y=0.1,size=12)
ax3.set_title(u"皮克",size=18,position = (0.5,0.95))
ax3.set_yticks([20,40,60,80,100])
player["Q"] = np.append(player["Q"],player["Q"][0])
ax4.plot(theta,player["Q"],"y")
ax4.fill(theta,player["Q"],"y",alpha=0.5)
ax4.set_xticks(theta)
ax4.set_xticklabels(ability_label,y=0.1,size=12)
ax4.set_title(u"切赫",size=18,position = (0.5,0.95))
ax4.set_yticks([20,40,60,80,100])
plt.show()