from maya import cmds
def get_quad_count(mesh_name):
# Check if the given object exists
if not cmds.objExists(mesh_name):
print("Error: Object '{}' does not exist.".format(mesh_name))
return None
# Check if the object has a child polygon mesh
shapes = cmds.listRelatives(mesh_name, shapes=True, type='mesh')
if not shapes:
print("Error: '{}' does not have a polygon mesh child.".format(mesh_name))
return None
# Get the name of the polygon mesh child
quad_count = 0
for mesh_node in shapes:
# Only deal with mesh node
node_type = cmds.nodeType(mesh_node)
if node_type != "mesh":
continue
# Get the polygon faces of the mesh
faces = cmds.polyListComponentConversion(mesh_node, toVertexFace=True)
cmds.select(faces, replace=True)
# Convert the selection to quads (4-sided faces)
quads = cmds.polySelectConstraint(mode=3, type=0x0008, size=4)
# Get the count of quads
quad_count += cmds.polyEvaluate(f=True)
# Clear the selection
cmds.select(clear=True)
return quad_count
# Example usage:
if __name__ == '__main__':
object_name = 'pCube24'
_quad_count = get_quad_count(object_name)
if _quad_count is not None:
print("Quad count of '{}': {}".format(object_name, _quad_count))
maya获得物体的四边面数量
最新推荐文章于 2024-10-13 11:18:28 发布
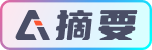