自成立以来,“一次编写代码–随处部署”的想法一直是Unity开发的口号。 借助Unity Technologies在引擎盖下处理的大多数艰苦的移植工作,移植游戏已在很大程度上要带来出色的用户体验。 在多个平台上保持高质量的产品意味着分辨率的独立性和对主要纵横比的支持。 (The idea of ‘Code Once – Deploy Everywhere’ has been a Unity development mantra since its inception. With most of the tough porting work handled under the hood by Unity Technologies, porting games has become largely about producing a great user experience. Maintaining a quality product across multiple platforms means resolution independence and support for major aspect ratios.)
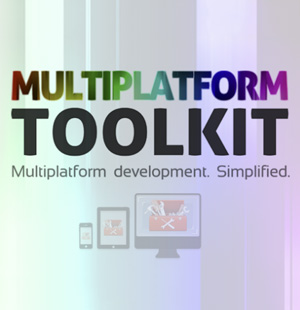
It means that the play button on Retina iPads needs to be scaled up 2x compared to non-retina devices. It means handling all of these platform-specific changes in a graceful, simple way, with a live preview of each platform right in the Unity Editor.
这意味着与非视网膜设备相比,视网膜iPad上的播放按钮需要放大2倍。 这意味着以一种优雅,简单的方式处理所有这些特定于平台的更改,并在Unity Editor中实时预览每个平台。
THAT is what Multiplatform Toolkit does best!
这就是Multiplatform Toolkit最擅长的!
讲解 (Tutorial)
So how does it do all of this? Well, lets walk through a quick example.
那么,这是怎么做的呢? 好吧,让我们来看一个简单的例子。
Let’s say you wanted to scale up a button 2x on iPhone to be large enough to hit with our big fat human fingers. The first thing we need to do is attach the PlatformSpecfics component to the button’s transform. (Fig. 1)
假设您想将iPhone上的按钮放大2倍,以至于可以用我们笨拙的大手指敲打。 我们需要做的第一件事是将PlatformSpecfics组件附加到按钮的转换中。 (图。1)
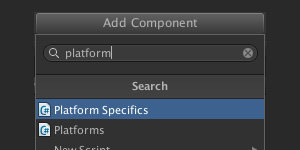
Fig 1
图。1
Then, in the inspector we can choose to tag the object with the appropriate platform-specific data. In this case we want to pick Scale by Platform. (Fig. 2)
然后,在检查器中,我们可以选择使用适当的平台特定数据标记对象。 在这种情况下,我们要选择“按平台缩放”。 (图2)
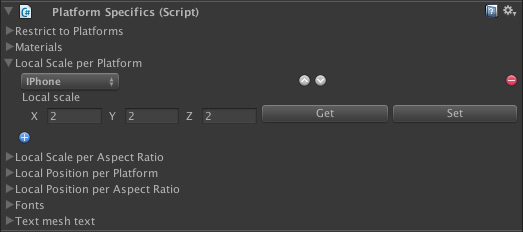
Fig. 2
图2
Hit the plus sign and choose iPhone as your platform. In our example, the button already has a (1,1,1) scale, and on iPhones we want it to be (2,2,2). You can manually input the target scale for iPhone or use the get and set buttons to either grab the current value from the transform or apply the selected value to the transform. These help avoid having to copy paste values manually, especially when using the scale tool to eyeball sizes. Make sure to apply the (2,2,2) scale to iPhone Retina as well! It’s up to you whether you want this to apply to iPhone 5. It’s as simple as adding it as a third option. (Fig. 3)
点击加号,然后选择iPhone作为平台。 在我们的示例中,按钮已经具有(1,1,1)比例,在iPhone上,我们希望其为(2,2,2)。 您可以手动输入iPhone的目标比例,或使用“获取”和“设置”按钮从转换中获取当前值,或将所选值应用于转换。 这些有助于避免必须手动复制粘贴值,尤其是在使用缩放工具查看大小时。 确保将(2,2,2)比例也应用到iPhone Retina! 是否要将其应用于iPhone 5由您自己决定。将其添加为第三个选项就很简单。 (图3)
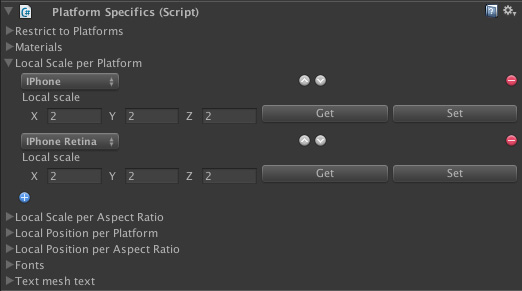
Fig. 3
图3
Now our button will scale up 2x on iPhone platforms. What? You don’t believe me? Well then, let’s test it within the Unity editor with our handy platform emulator. Go to the Window menu -> MultiPlatform Toolkit -> EasyPlatform. An editor window will appear. Dock it for easy access. This tool does one thing: lets you pick which platform you’d like to emulate in the Unity Editor. In our case, we want to pick iPhone and then hit Play in the Unity Editor. (Fig. 4)
现在,我们的按钮将在iPhone平台上放大2倍。 什么? 你不相信我吗? 那么,让我们使用方便的平台模拟器在Unity编辑器中对其进行测试。 转到窗口菜单-> MultiPlatform Toolkit-> EasyPlatform。 将出现一个编辑器窗口。 将其停靠以便于访问。 该工具做一件事:让您选择要在Unity Editor中模拟的平台。 在本例中,我们要选择iPhone,然后在Unity Editor中点击Play。 (图4)
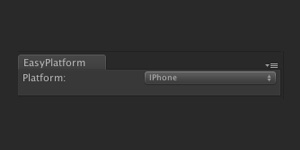
Fig. 4
图4
The platform-specific changes will occur on Awake() and you won’t need to spend minutes doing actual builds to the hardware to test out your changes. It only takes seconds! (Fig. 5)
特定于平台的更改将在Awake()上进行,您无需花费几分钟时间对硬件进行实际构建即可测试您的更改。 只需要几秒钟! (图5)
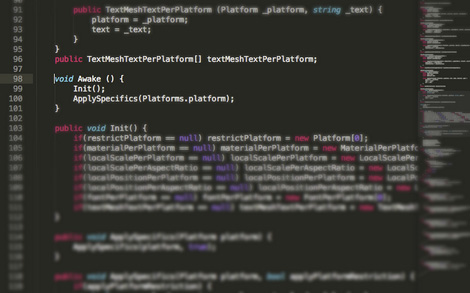
Fig. 5
图5
This is one tiny example of the ways MultiPlatform Toolkit saves hours upon hours when dealing with platform-specific changes. Let’s quickly dig into another code-centric example.
这是MultiPlatform Toolkit在处理特定于平台的更改时可以节省数小时的方式的一个小例子。 让我们快速研究另一个以代码为中心的示例。
Let’s say you wanted to load, at runtime, a small, compressed texture for use as a backdrop in your menus on iPhones, while you wanted to load a huge, 2048x2048px screen backdrop for your Retina iPad and Standalone PC/Mac builds. Easy! The quickest, most seamless way to do this is to Resources.Load() your texture from disk and pick the right one. MPTK makes it as simple as this:
假设您想在运行时加载一个小的压缩纹理,以用作iPhone菜单上的背景,而您想为您的Retina iPad和独立PC / Mac版本加载一个2048x2048px的大屏幕背景。 简单! 最快,最无缝的方法是从磁盘访问Resources.Load()纹理并选择正确的纹理。 MPTK使它变得如此简单:
1
2
3
4
5
6
7
|
if(Platforms.platform == Platform.iPadRetina || Platform.platform == Platform.Standalone) { //iPad 3, iPad 4, and PC/Mac/Linux
//load the huge texture
myRenderer.sharedMaterial.mainTexture = Resources.Load("backgrounds/hugeTexture");
} else { //All other platforms
//load the tiny texture
myRenderer.sharedMaterial.mainTexture = Resources.Load("backgrounds/tinyTexture");
}
|
1
2
3
4
5
6
7
|
if ( Platforms . platform == Platform . iPadRetina || Platform . platform == Platform . Standalone ) { //iPad 3, iPad 4, and PC/Mac/Linux
//load the huge texture
myRenderer . sharedMaterial . mainTexture = Resources . Load ( "backgrounds/hugeTexture" ) ;
} else { //All other platforms
//load the tiny texture
myRenderer . sharedMaterial . mainTexture = Resources . Load ( "backgrounds/tinyTexture" ) ;
}
|
Easy!
简单!
Customers have told us that the more they use MultiPlatform Toolkit, the more they wonder how they got by without it. If you’re doing any kind of Unity project that involves publishing to multiple targets, feel free to check out our product. We developed it because we needed it for our own games and realized it would be useful for others!
客户告诉我们,他们使用MultiPlatform Toolkit的次数越多,他们就越想知道如果没有它,该怎么办。 如果您正在从事涉及发布到多个目标的任何Unity项目,请随时查看我们的产品。 我们开发它是因为我们自己的游戏需要它,并意识到它将对其他人有用!
For more information about MultiPlatform Toolkit, check out the Unity Asset Store page or our product website. Happy publishing!
有关MultiPlatform Toolkit的更多信息,请查看Unity Asset Store页面或我们的产品网站 。 祝出版愉快!
返回资产商店 (Back to the Asset Store)
翻译自: https://blogs.unity3d.com/2013/07/18/tutorial-multiplatform-toolkit-by-owlchemy-labs/