using UnityEngine;
using UnityEngine.UI;
using System.Collections;
using UnityEngine.EventSystems;
public class ColorManager : MonoBehaviour, IDragHandler
{
//本脚本上的物体的区域
RectTransform rt;
//滑动条上的脚本
private ColorRGB CRGB;
//面板上的脚本
private ColorPanel CP;
//圆环上的脚本
private ColorCircle CC;
//滑动条 gameobject
public Slider sliderCRGB;
//显示面板 gameobjcet
public Image colorShow;
//物体销毁时调用 关闭时调用
void OnDisable()
{
//事件移除
CC.getPos -= CC_getPos;
}
//委托调用
private void CC_getPos(Vector2 pos)
{
Color getColor= CP.GetColorByPosition(pos);
colorShow.color = getColor;
}
// Use this for initialization
void Start () {
//获取 此脚本对象的 (RectTransform)有效区域
rt = GetComponent<RectTransform>();
//获取 子类下的脚本对象
CRGB = GetComponentInChildren<ColorRGB>();
CP = GetComponentInChildren<ColorPanel>();
CC = GetComponentInChildren<ColorCircle>();
//滑动条 事件
sliderCRGB.onValueChanged.AddListener(OnCRGBValueChanged);
//事件添加
CC.getPos += CC_getPos;
}
public void OnDrag(PointerEventData eventData)
{
//Vector3 wordPos;
将UGUI的坐标转为世界坐标
//if (RectTransformUtility.ScreenPointToWorldPointInRectangle(rt, eventData.position, eventData.pressEventCamera, out wordPos))
// rt.position = wordPos;
}
//滑动条颜色 付给面板
void OnCRGBValueChanged(float value)
{
Color endColor=CRGB.GetColorBySliderValue(value);
CP.SetColorPanel(endColor);
CC.setShowColor();
}
}
using UnityEngine;
using System.Collections.Generic;
using UnityEngine.UI;
using UnityEngine.EventSystems;
public class ColorPanel : MonoBehaviour, IPointerClickHandler, IDragHandler
{
//接收面板Rawimage 中的texture
Texture2D tex2d;
//面板 Rawimage
public RawImage ri;
//面板尺寸
int TexPixelLength = 256;
//颜色矩阵
Color[,] arrayColor;
RectTransform rt;
//圆环
public RectTransform circleRect;
// Use this for initialization
void Start()
{
arrayColor = new Color[TexPixelLength, TexPixelLength];
tex2d = new Texture2D(TexPixelLength, TexPixelLength, TextureFormat.RGB24, true);
ri.texture = tex2d;
rt = GetComponent<RectTransform>();
SetColorPanel(Color.red);
}
// Update is called once per frame
void Update()
{
if (Input.GetKeyDown(KeyCode.Space))
{
Color end = new Color(Random.Range(0.0f, 1.0f), Random.Range(0.0f, 1.0f), Random.Range(0.0f, 1.0f), 1.0f);
SetColorPanel(end);
}
}
//面板颜色添加
public void SetColorPanel(Color endColor)
{
Color[] CalcArray = CalcArrayColor(endColor);
tex2d.SetPixels(CalcArray);
tex2d.Apply();
}
//面板颜色布局
Color[] CalcArrayColor(Color endColor)
{
Color value = (endColor - Color.white) / (TexPixelLength - 1);
for (int i = 0; i < TexPixelLength; i++)
{
arrayColor[i, TexPixelLength - 1] = Color.white + value * i;
}
for (int i = 0; i < TexPixelLength; i++)
{
value = (arrayColor[i, TexPixelLength - 1] - Color.black) / (TexPixelLength - 1);
for (int j = 0; j < TexPixelLength; j++)
{
arrayColor[i, j] = Color.black + value * j;
}
}
List<Color> listColor = new List<Color>();
for (int i = 0; i < TexPixelLength; i++)
{
for (int j = 0; j < TexPixelLength; j++)
{
listColor.Add(arrayColor[j, i]);
}
}
return listColor.ToArray();
}
/// <summary>
/// 获取颜色by坐标
/// </summary>
/// <param name="pos"></param>
/// <returns></returns>
public Color GetColorByPosition(Vector2 pos)
{
Texture2D tempTex2d = (Texture2D)ri.texture;
Color getColor = tempTex2d.GetPixel((int)pos.x, (int)pos.y);
return getColor;
}
//点击事件
public void OnPointerClick(PointerEventData eventData)
{
Vector3 wordPos;
//将UGUI的坐标转为世界坐标
if (RectTransformUtility.ScreenPointToWorldPointInRectangle(rt, eventData.position, eventData.pressEventCamera, out wordPos))
circleRect.position = wordPos;
//调用 自定义事件
circleRect.GetComponent<ColorCircle>().setShowColor();
}
//拖拽事件
public void OnDrag(PointerEventData eventData)
{
Vector3 wordPos;
//将UGUI的坐标转为世界坐标
if (RectTransformUtility.ScreenPointToWorldPointInRectangle(rt, eventData.position, eventData.pressEventCamera, out wordPos))
circleRect.position = wordPos;
//调用 自定义事件
circleRect.GetComponent<ColorCircle>().setShowColor();
}
}
using UnityEngine;
using System.Collections;
using UnityEngine.EventSystems;
public class ColorCircle : MonoBehaviour, IDragHandler
{
//委托事件
public delegate void RetureTextuePosition(Vector2 pos);
//事件
public event RetureTextuePosition getPos;
RectTransform rt;
//活动区域
public int width = 256;
public int height = 256;
// Use this for initialization
void Start () {
rt = GetComponent<RectTransform>();
}
// Update is called once per frame
void Update () {
}
//调用事件
public void OnDrag(PointerEventData eventData)
{
Vector3 wordPos;
//将UGUI的坐标转为世界坐标
if (RectTransformUtility.ScreenPointToWorldPointInRectangle(rt, eventData.position, eventData.pressEventCamera, out wordPos))
rt.position = wordPos;
if (rt.anchoredPosition.x <= 0)
rt.anchoredPosition = new Vector2(0, rt.anchoredPosition.y);
if (rt.anchoredPosition.y <= 0)
rt.anchoredPosition = new Vector2(rt.anchoredPosition.x, 0);
if (rt.anchoredPosition.x >= width-1)
rt.anchoredPosition = new Vector2(width-1,rt.anchoredPosition.y);
if (rt.anchoredPosition.y >= height-1)
rt.anchoredPosition = new Vector2(rt.anchoredPosition.x, height-1);
getPos(rt.anchoredPosition);
}
//滑动条 调用委托
public void setShowColor()
{
getPos(rt.anchoredPosition);
}
}
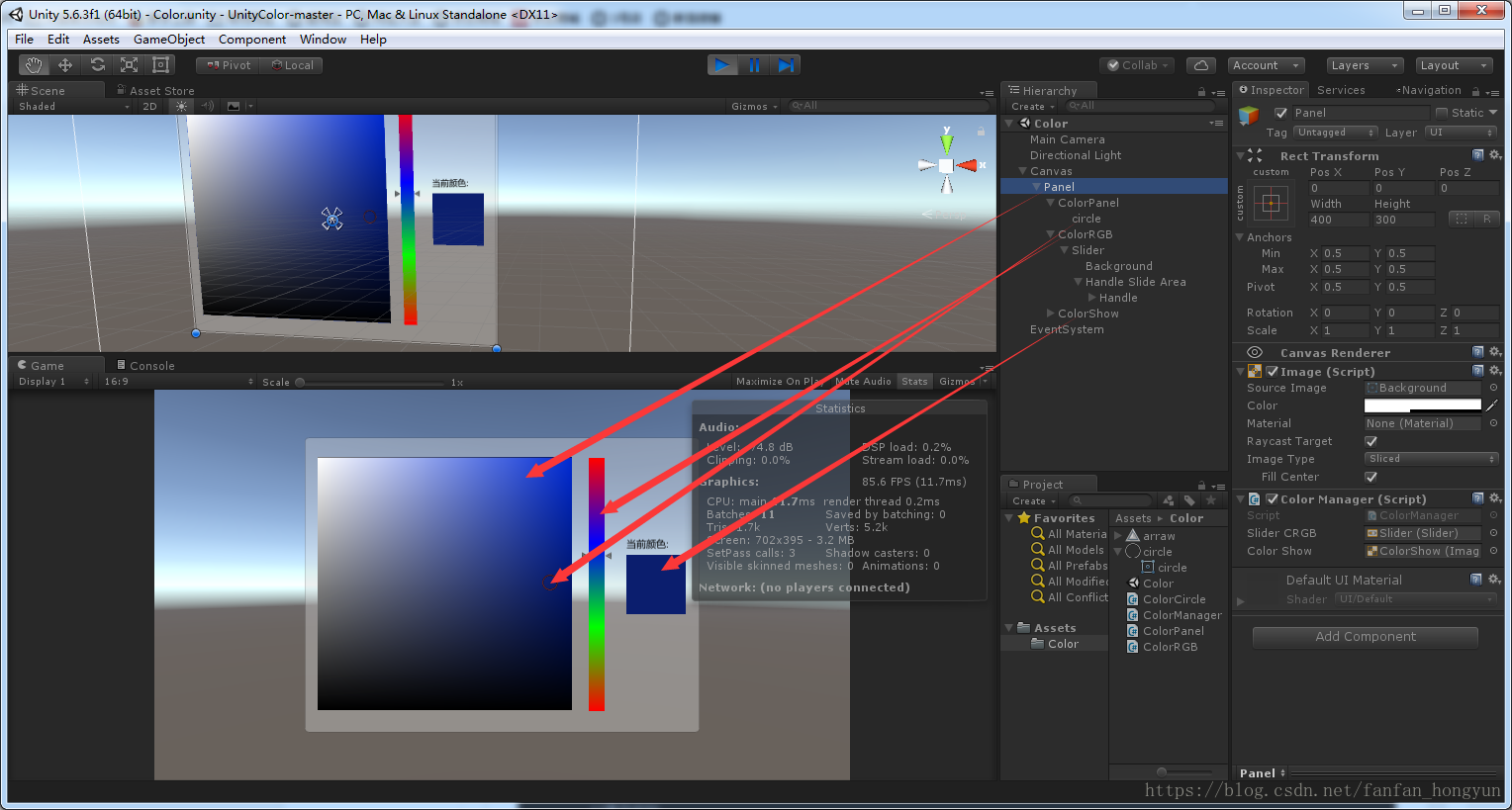