外观模式:为子系统中的一组接口提供一个统一的入口。外观模式定义了一个高层接口,这个接口使得这一子系统更加容易使用。
模式优点:
-
它对客户端屏蔽了子系统组件,减少了客户端所需处理的对象数目,并使得子系统使用起来更加容易
-
它实现了子系统与客户端之间的松耦合关系,这使得子系统的变化不会影响到调用它的客户端,只需要调整外观类即可
-
一个子系统的修改对其他子系统没有任何影响,而且子系统的内部变化也不会影响到外观对象
模式缺点:
-
不能很好地限制客户端直接使用子系统类,如果对客户端访问子系统类做太多的限制则减少了可变性和灵活性
-
如果设计不当,增加新的子系统可能需要修改外观类的源代码,违背了开闭原则
模式适用环境:
-
要为访问一系列复杂的子系统提供一个简单入口
-
客户端程序与多个子系统之间存在很大的依赖性
-
在层次化结构中,可以使用外观模式的定义系统中每一层的入口,层与层之间不直接产生联系,而是通过外观类建立联系,降低层之间的耦合度
UML
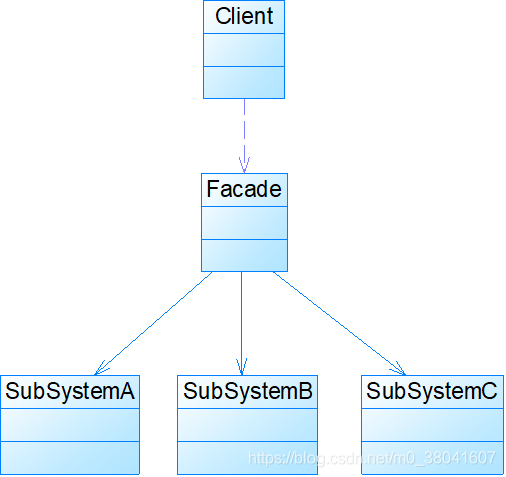
外观模式原型
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class FacadePrototype : MonoBehaviour
{
// Start is called before the first frame update
void Start()
{
Facade facade = new Facade();
facade.SystemFunctionA();
facade.SystemFunctionB();
facade.SystemFunctionC();
}
// Update is called once per frame
void Update()
{
}
}
public class Facade
{
private SystemA systemA;
private SystemB systemB;
private SystemC systemC;
public Facade()
{
systemA = new SystemA();
systemB = new SystemB();
systemC = new SystemC();
}
public void SystemFunctionA()
{
systemA.SystemFunction();
}
public void SystemFunctionB()
{
systemB.SystemFunction();
}
public void SystemFunctionC()
{
systemC.SystemFunction();
}
}
public class ISystem
{
public virtual void SystemFunction() { }
}
public class SystemA : ISystem
{
public override void SystemFunction()
{
base.SystemFunction();
Debug.Log("执行系统A功能");//系统功能可能需要依赖一个或多个子系统提供
}
}
public class SystemB : ISystem
{
public override void SystemFunction()
{
base.SystemFunction();
Debug.Log("执行系统B功能");
}
}
public class SystemC : ISystem
{
public override void SystemFunction()
{
base.SystemFunction();
Debug.Log("执行系统C功能");
}
}
简单demo
demo:手机开通套餐
20元套餐(30条短信 100M流量 100分钟通话)
30元套餐(50条短信 200M流量 150分钟通话)
50元套餐(100条短信 500M流量 200分钟通话)
消息
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class SMS
{
public void SMS_30()
{
Debug.Log("30条短信");
}
public void SMS_50()
{
Debug.Log("50条短信");
}
public void SMS_100()
{
Debug.Log("100条短信");
}
}
流量
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class Flow
{
public void Flow_100()
{
Debug.Log("100M流量");
}
public void Flow_200()
{
Debug.Log("200M流量");
}
public void Flow_500()
{
Debug.Log("500M流量");
}
}
通话时间
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class CallTime
{
public void Call_100()
{
Debug.Log("通话100分钟");
}
public void Call_150()
{
Debug.Log("通话150分钟");
}
public void Call_200()
{
Debug.Log("通话200分钟");
}
}
手机套餐外观类
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PhoneFacade
{
private SMS sMS;
private Flow flow;
private CallTime callTime;
public PhoneFacade()
{
sMS = new SMS();
flow = new Flow();
callTime = new CallTime();
}
public void SetMial_20()//20元套餐接口
{
Debug.Log("开通20元套餐");
sMS.SMS_30();
flow.Flow_100();
callTime.Call_100();
}
public void SetMial_30()//30元套餐接口
{
Debug.Log("开通30元套餐");
sMS.SMS_50();
flow.Flow_200();
callTime.Call_150();
}
public void SetMial_50()//50元套餐接口
{
Debug.Log("开通50元套餐");
sMS.SMS_100();
flow.Flow_200();
callTime.Call_150();
}
}
调用类测试
using System.Colections;
using System.Collections.Generic;
using UnityEngine;
public class User : MonoBehaviour
{
void Start()
{
PhoneFacade phoneFacade = new PhoneFacade();
phoneFacade.SetMial_20();
Debug.Log("******************************************");
phoneFacade.SetMial_30();
Debug.Log("******************************************");
phoneFacade.SetMial_50();
}
}