Lemmings1
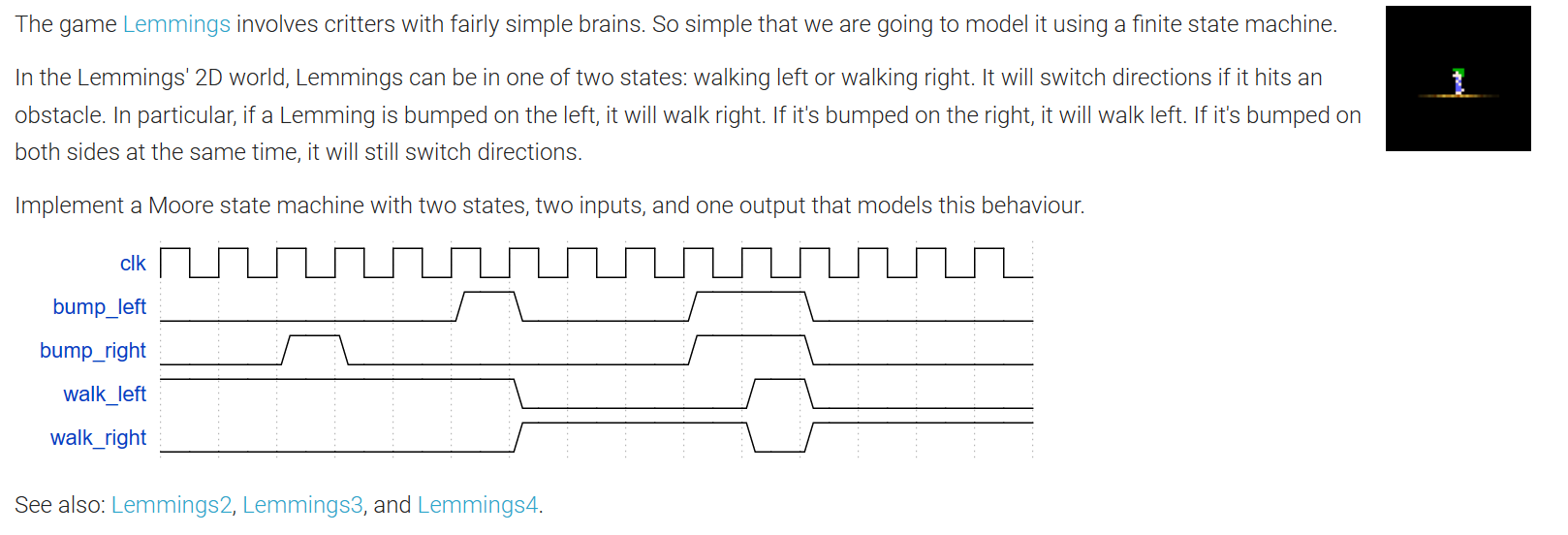
module top_module(
input clk,
input areset, // Freshly brainwashed Lemmings walk left.
input bump_left,
input bump_right,
output walk_left,
output walk_right); //
// parameter LEFT=0, RIGHT=1, ...
parameter LEFT=0, RIGHT=1;
reg state, next_state;
always @(*) begin
// State transition logic
if(bump_left && ~bump_right) next_state=RIGHT;
else if(bump_right && ~bump_left) next_state=LEFT;
else begin
case(state)
LEFT: begin if(bump_right&bump_left) next_state=RIGHT;
else next_state=LEFT; end
RIGHT: begin if(bump_right&bump_left) next_state=LEFT;
else next_state=RIGHT; end
endcase
end
end
always @(posedge clk, posedge areset) begin
// State flip-flops with asynchronous reset
if(areset) state<=LEFT;
else state<=next_state;
end
// Output logic
// assign walk_left = (state == ...);
// assign walk_right = (state == ...);
assign walk_left=state?0:1;
assign walk_right=state?1:0;
endmodule
Lemmings2(掉落)
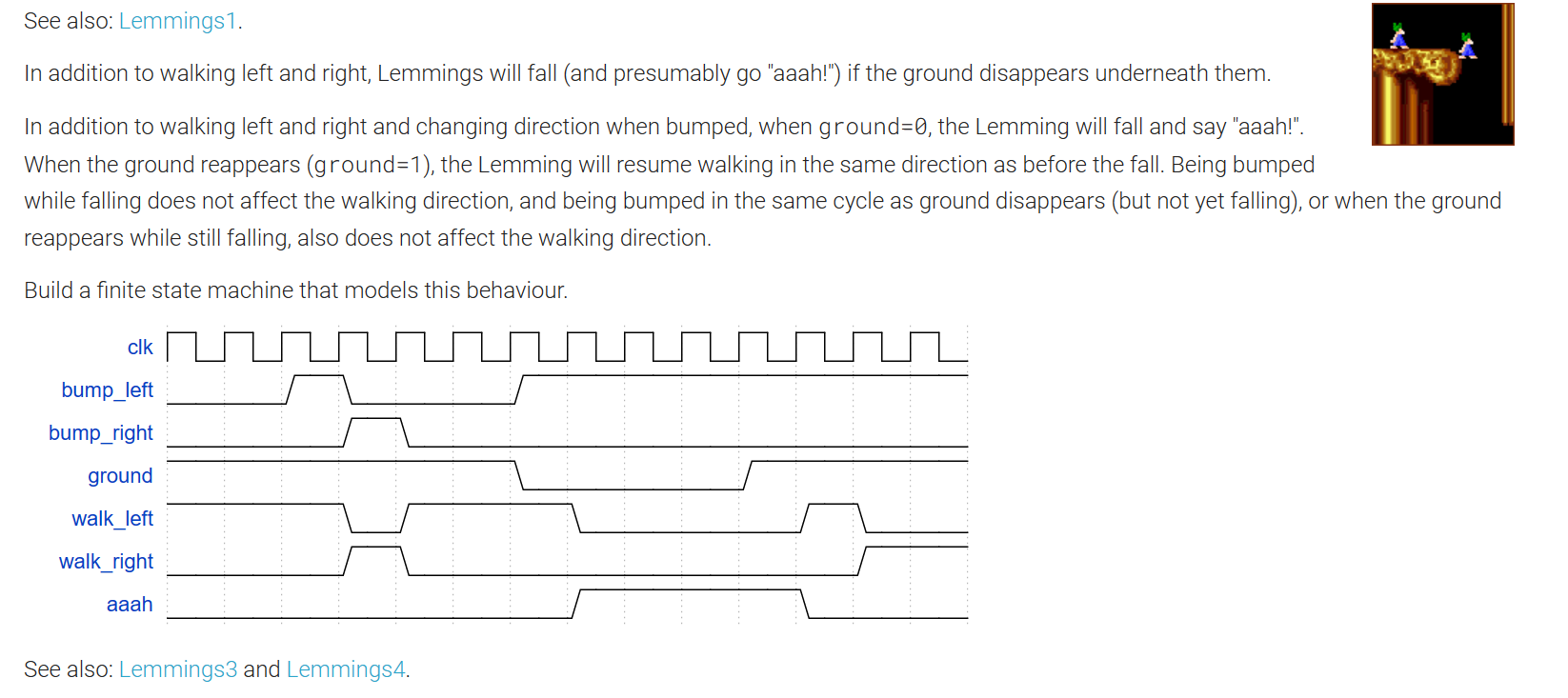
//错误代码:逻辑错误,缺少两种状态
module top_module(
input clk,
input areset, // Freshly brainwashed Lemmings walk left.
input bump_left,
input bump_right,
input ground,
output walk_left,
output walk_right,
output aaah );
parameter LEFT=0, RIGHT=1;
reg state, next_state;
always@(*) begin
if(bump_left & ~bump_right) next_state=RIGHT;
else if(~bump_right & bump_left) next_state=LEFT;
else begin
case(state)
LEFT: if(bump_left & bump_right) next_state=RIGHT;
else next_state=LEFT;
RIGHT: if(bump_left & bump_right) next_state=LEFT;
else next_state=RIGHT;
endcase
end
end
always@(posedge clk or posedge areset) begin
if(areset) state<=LEFT;
else state<=next_state;
end
always@(posedge clk or posedge areset) begin
if(areset) aaah<=0;
else begin
if(ground) aaah<=0;
else aaah<=1;
end
end
always@(posedge clk or posedge areset) begin
if(areset) begin walk_left<=1; walk_right<=0; end
else begin
if(ground) begin walk_left<=state?0:1; walk_right<=state?1:0; end
else begin walk_left<=0; walk_right<=0; end
end
end
endmodule
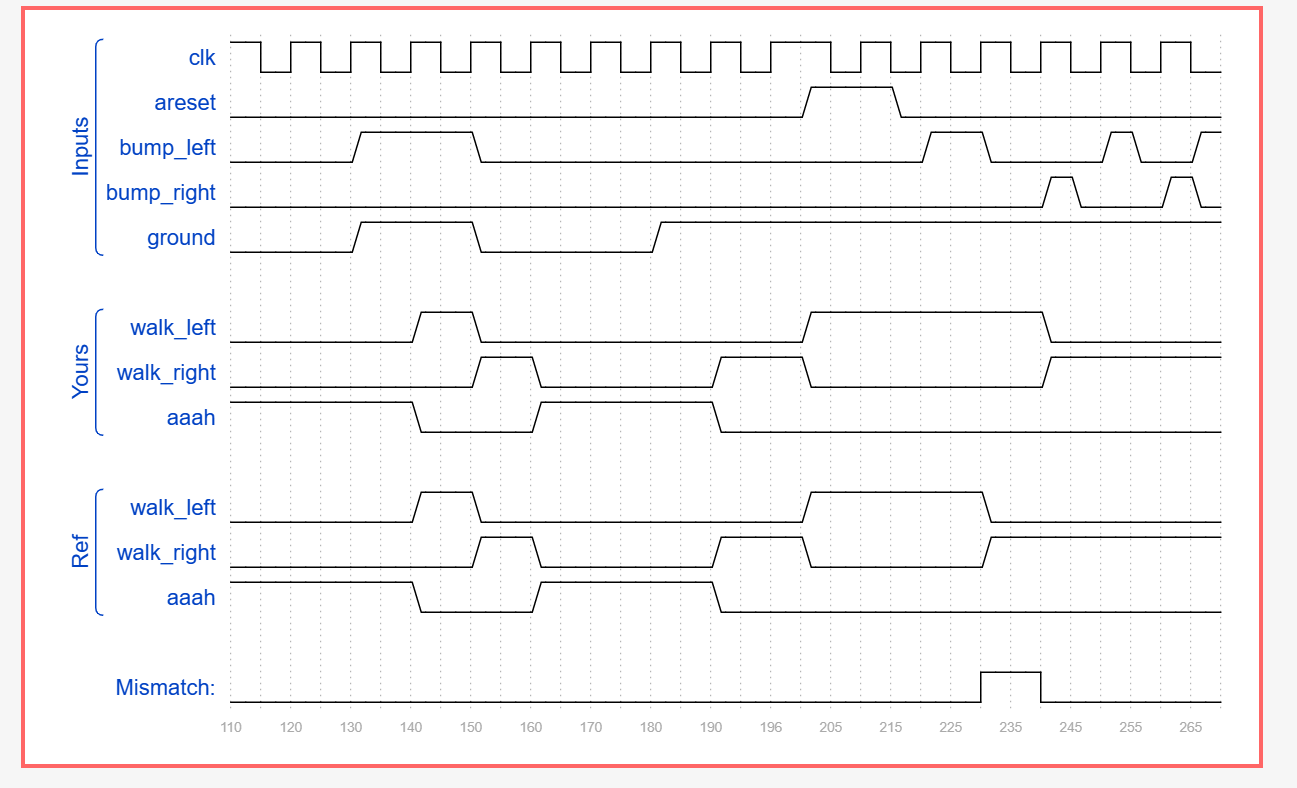
正确代码: 注意:三目运算符为右结合
module top_module(
input clk,
input areset, // Freshly brainwashed Lemmings walk left.
input bump_left,
input bump_right,
input ground,
output walk_left,
output walk_right,
output aaah );
parameter LEFT=2'd00, RIGHT=2'd01, D_LEFT=2'd11, D_RIGHT=2'd10;
reg [1:0]state;
reg [1:0]next_state;
always@(*) begin
case(state)
LEFT:next_state<=(~ground)?D_LEFT:(bump_left)?RIGHT:LEFT;
RIGHT:next_state<=(~ground)?D_RIGHT:(bump_right)?LEFT:RIGHT;
D_LEFT:next_state<=(ground)?LEFT:D_LEFT;
D_RIGHT:next_state<=(ground)?RIGHT:D_RIGHT;
endcase
end
always@(posedge clk or posedge areset) begin
if(areset) begin
state<=LEFT; end
else
state<=next_state;
end
assign walk_left=(state==LEFT);
assign walk_right=(state==RIGHT);
assign aaah=(state==D_LEFT | state==D_RIGHT);
endmodule
Lemmings3 (挖掘)
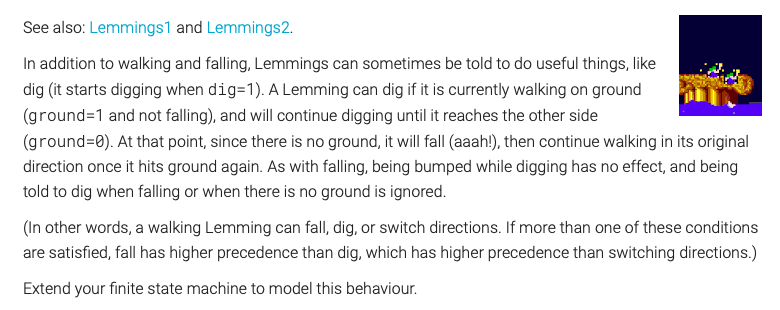
module top_module(
input clk,
input areset, // Freshly brainwashed Lemmings walk left.
input bump_left,
input bump_right,
input ground,
input dig,
output walk_left,
output walk_right,
output aaah,
output digging );
parameter left=0, right=1, d_left=2, d_right=3, digging_left=4, digging_right=5;
reg [2:0]state;
reg [2:0]next_state;
always@(*) begin
case(state)
left: next_state=(~ground)?d_left:(dig?digging_left:(bump_left?right:left));
right: next_state=(~ground)?d_right:(dig?digging_right:(bump_right?left:right));
d_right: next_state=(~ground)?d_right:right;
d_left: next_state=(~ground)?d_left:left;
digging_left: next_state=(~ground)?d_left:digging_left;
digging_right: next_state=(~ground)?d_right:digging_right;
endcase
end
always@(posedge clk or posedge areset) begin
if(areset) begin state<=left; end
else state<=next_state;
end
assign walk_left=(state==left);
assign walk_right=(state==right);
assign aaah=(state==d_left | state==d_right);
assign digging=(state==digging_left | state==digging_right);
endmodule
Lemmings4(死亡)
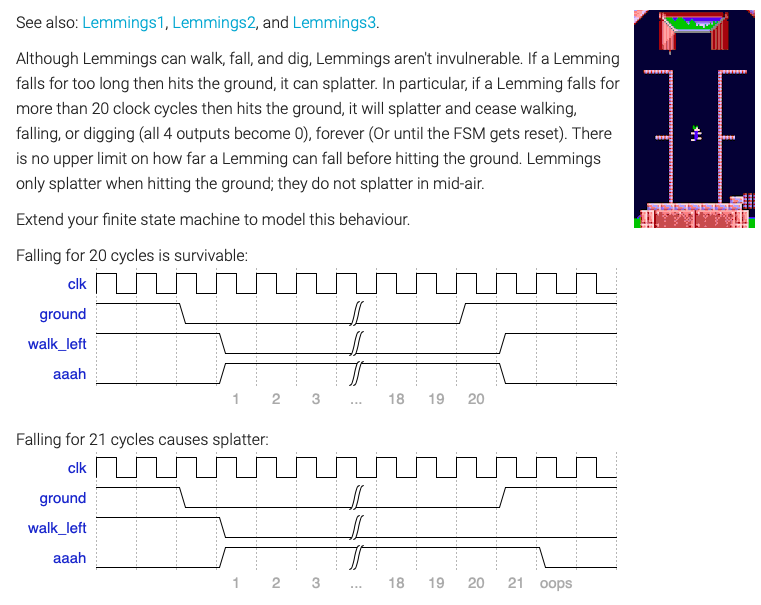
module top_module(
input clk,
input areset, // Freshly brainwashed Lemmings walk left.
input bump_left,
input bump_right,
input ground,
input dig,
output walk_left,
output walk_right,
output aaah,
output digging);
parameter left=0,right=1,d_left=2,d_right=3,dig_left=4,dig_right=5,die=6;
reg [3:0]state;
reg [3:0]next_state;
reg [7:0]fall_time;
always@(*) begin
case(state)
left: next_state=(~ground)?d_left:(dig?dig_left:(bump_left?right:left));
right: next_state=(~ground)?d_right:(dig?dig_right:(bump_right?left:right));
d_right: next_state=(fall_time>19&&ground)?die:(~ground)?d_right:right;
d_left: next_state=(fall_time>19&&ground)?die:(~ground)?d_left:left;
dig_left: next_state=(~ground)?d_left:dig_left;
dig_right: next_state=(~ground)?d_right:dig_right;
die: next_state=die;
endcase
end
always@(posedge clk, posedge areset) begin
if(areset) begin state<=left; fall_time<=0; end//缺少fall_time出错
else begin
state<=next_state;
if(state==d_right && next_state==d_right) fall_time++;
else if(state==d_right&&next_state==right) fall_time=0;
if(state==d_left && next_state==d_left) fall_time++;
else if(state==d_left&&next_state==left) fall_time=0;//不能直接写成else,为die时出错
end
end
assign walk_left=(state==left);
assign walk_right=(state==right);
assign aaah=(state==d_right | state==d_left);
assign digging=(state==dig_left | state==dig_right);
endmodule
每次都缺东少西!!!