Unity屏幕截图、区域截图、读取图片、WebGL长截屏并下载到本地jpg
一、全屏截图并保存到StreamingAssets路径下
Texture2D screenShot;
public Image image;
public void Jietu()
{
StartCoroutine(ScrrenCapture(new Rect(0, 0, Screen.width, Screen.height), 0));
}
IEnumerator ScrrenCapture(Rect rect, int a)
{
screenShot = new Texture2D((int)rect.width, (int)rect.height, TextureFormat.RGB24, false);
yield return new WaitForEndOfFrame();
screenShot.ReadPixels(rect, 0, 0);
screenShot.Apply();
yield return new WaitForSeconds(0.1f);
Sprite sp = Sprite.Create(screenShot, new Rect(0, 0, screenShot.width, screenShot.height), new Vector2(0.5f, 0.5f), 100.0f);
image.sprite = sp;
byte[] bytes = screenShot.EncodeToJPG();
string filename = Application.streamingAssetsPath + "/Images/Screenshot" + DateTime.UtcNow.Ticks + ".png";
File.WriteAllBytes(filename, bytes);
}
二、区域截图并保存到StreamingAssets路径下
Texture2D screenShot;
public Image image;
public Image im;
Texture2D texture2ds;
public void Jietu()
{
StartCoroutine(getScreenTexture(im.rectTransform));
}
public IEnumerator getScreenTexture(RectTransform rectT)
{
yield return new WaitForEndOfFrame();
texture2ds = new Texture2D((int)rectT.rect.width, (int)rectT.rect.height, TextureFormat.RGB24, true);
float x = rectT.localPosition.x + (Screen.width - rectT.rect.width) / 2;
float y = rectT.localPosition.y + (Screen.height - rectT.rect.height) / 2;
Rect position = new Rect(x, y, rectT.rect.width, rectT.rect.height);
texture2ds.ReadPixels(position, 0, 0, true);
texture2ds.Apply();
Sprite sp = Sprite.Create(texture2ds, new Rect(0, 0, texture2ds.width, texture2ds.height), Vector2.zero);
image.sprite = sp;
byte[] bytes = texture2ds.EncodeToJPG();
string filename = Application.streamingAssetsPath + "/Images/Screenshot" + DateTime.UtcNow.Ticks + ".png";
File.WriteAllBytes(filename, bytes);
}
三、unity发布WebGL屏幕长截屏并通过浏览器下载到本地jpg文件
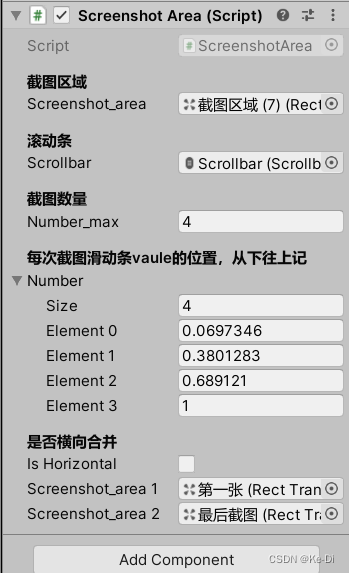
using System.Collections;
using System.IO;
using System.Runtime.InteropServices;
using UnityEngine;
using UnityEngine.UI;
public class ScreenshotArea : MonoBehaviour
{
[Header("截图区域")]
public RectTransform screenshot_area;
[Header("滚动条")]
public Scrollbar scrollbar;
[Header("截图数量")]
public int number_max;
[Header("每次截图滑动条vaule的位置,从下往上记")]
public float[] number;
[Header("是否横向合并")]
public bool isHorizontal;
Texture2D[] texture2ds;
Texture2D merge_image;
string image_name="测试";
public RectTransform screenshot_area1;
public RectTransform screenshot_area2;
private void Start()
{
texture2ds = new Texture2D[number_max];
}
public void OnClick_调用()
{
Screen.fullScreen = true;
StartCoroutine(getScreenTexture(screenshot_area));
}
#region 屏幕多次截图
public IEnumerator getScreenTexture(RectTransform rectT)
{
scrollbar.value = number[0];
yield return new WaitForEndOfFrame();
for (int i = 0; i < number_max; i++)
{
texture2ds[i] = new Texture2D((int)rectT.rect.width, (int)rectT.rect.height, TextureFormat.RGB24, true);
float x = rectT.localPosition.x + (Screen.width - rectT.rect.width) / 2;
float y = rectT.localPosition.y + (Screen.height - rectT.rect.height) / 2;
Rect position = new Rect(x, y, rectT.rect.width, rectT.rect.height);
texture2ds[i].ReadPixels(position, 0, 0, true);
texture2ds[i].Apply();
if (i < number_max - 1)
scrollbar.value = number[i + 1];
if (i == 0)
{
rectT = screenshot_area;
}
if (i == number_max - 2)
{
rectT = screenshot_area1;
}
yield return new WaitForEndOfFrame();
}
merge_image = MergeImage(texture2ds);
#if UNITY_EDITOR
byte[] bytes = merge_image.EncodeToJPG();
string filename = Application.streamingAssetsPath + "/Screenshot" + UnityEngine.Random.Range(0, 1000) + ".png";
File.WriteAllBytes(filename, bytes);
#endif
DownLoad(merge_image);
}
#endregion
#region 下载图片
Sprite sprite;
private void DownLoad(Texture2D screenShot)
{
sprite = Sprite.Create(screenShot, new Rect(0, 0, screenShot.width, screenShot.height), new Vector2(0.5f, 0.5f));
byte[] photoByte = getImageSprite();
if (photoByte != null)
{
DownloadImage(photoByte, image_name+".jpg");
}
else
{
Debug.LogError("<color=red>下载失败</color>");
}
}
private byte[] getImageSprite()
{
if (sprite)
{
return sprite.texture.EncodeToJPG();
}
return null;
}
#endregion
#region 调用js方法下载
[DllImport("__Internal")]
private static extern void ImageDownloader(string str, string fn);
public void DownloadImage(byte[] imageData, string imageFileName = "newpic")
{
#if UNITY_EDITOR
Debug.Log("<color=blue>编辑器无法下载</color>");
#else
if (imageData != null)
{
Debug.Log("Downloading..." + imageFileName);
ImageDownloader(System.Convert.ToBase64String(imageData), imageFileName);
}
#endif
}
#endregion
#region 合并多张图片
public Texture2D MergeImage(Texture2D[] tex)
{
if (tex.Length == 0)
return null;
int width = 0, height = 0;
for (int i = 0; i < tex.Length; i++)
{
if (isHorizontal == false)
{
height += tex[i].height;
if (i > 0)
{
if (tex[i].width > tex[i - 1].width)
{
width = tex[i].width;
}
}
else width = tex[i].width;
}
else
{
width += tex[i].width;
if (i > 0)
{
if (tex[i].height > tex[i - 1].height)
{
height = tex[i].height;
}
}
else height = tex[i].height;
}
}
Texture2D texture2D = new Texture2D(width, height);
int x = 0, y = 0;
for (int i = 0; i < tex.Length; i++)
{
Color32[] color = tex[i].GetPixels32(0);
if (i > 0)
{
if (isHorizontal == false)
{
texture2D.SetPixels32(x, y += tex[i - 1].height, tex[i].width, tex[i].height, color);
}
else
{
texture2D.SetPixels32(x += tex[i - 1].width, y, tex[i].width, tex[i].height, color);
}
}
else
{
texture2D.SetPixels32(x, y, tex[i].width, tex[i].height, color);
}
}
texture2D.Apply();
return texture2D;
}
#endregion
}