0xff分析:
0x其实就是16进制,15*16 + 15 = 255
或者:
转化为2进制,就是8个1,因此也是255
/
C#以前都是不支持多返回值,当需要返回多个值的时候,就会感觉比较麻烦,通常的做法有
1.采用ref,out 关键字返回
2.定义类或者结构体 返回对象
C# 6.0出来了新的语法 Tuple 支付返回多个值
示例:
1 class Demo
2 {
3 public void Run()
4 {
5 var num = Exec(5, 6);
6 Console.WriteLine(num.Item1); //第一个返回值
7 Console.WriteLine(num.Item2); //第二个返回值
8
9 Console.WriteLine();
10 var num2 = Exec2(5, 6);
11 Console.WriteLine(num2.Item1); //第一个返回值
12 Console.WriteLine(num2.Item2); //第二个返回值
13 Console.WriteLine(num2.Item3); //第三个返回值
14 }
15
16 public Tuple<int, int> Exec(int a, int b)
17 {
18 Tuple<int, int> tup = new Tuple<int, int>(a + b, a * b);
19 return tup;
20 }
21
22 public Tuple<int, int, int> Exec2(int a, int b)
23 {
24 Tuple<int, int, int> tup = new Tuple<int, int,int>(a + b, a * b, a - b);
25 return tup;
26 }
27
28 }
/
It really depends on what you're going for:
#if DEBUG
: The code in here won't even reach the IL on release.[Conditional("DEBUG")]
: This code will reach the IL, however calls to the method will be omitted unless DEBUG is set when the caller is compiled.
Personally I use both depending on the situation:
Conditional("DEBUG") Example: I use this so that I don't have to go back and edit my code later during release, but during debugging I want to be sure I didn't make any typos. This function checks that I type a property name correctly when trying to use it in my INotifyPropertyChanged stuff.
[Conditional("DEBUG")]
[DebuggerStepThrough]
protected void VerifyPropertyName(String propertyName)
{
if (TypeDescriptor.GetProperties(this)[propertyName] == null)
Debug.Fail(String.Format("Invalid property name. Type: {0}, Name: {1}",
GetType(), propertyName));
}
You really don't want to create a function using #if DEBUG
unless you are willing to wrap every call to that function with the same #if DEBUG
:
#if DEBUG
public void DoSomething() { }
#endif
public void Foo()
{
#if DEBUG
DoSomething(); //This works, but looks FUGLY
#endif
}
versus:
[Conditional("DEBUG")]
public void DoSomething() { }
public void Foo()
{
DoSomething(); //Code compiles and is cleaner, DoSomething always
//exists, however this is only called during DEBUG.
}
#if DEBUG example: I use this when trying to setup different bindings for WCF communication.
#if DEBUG
public const String ENDPOINT = "Localhost";
#else
public const String ENDPOINT = "BasicHttpBinding";
#endif
In the first example, the code all exists, but is just ignored unless DEBUG is on. In the second example, the const ENDPOINT is set to "Localhost" or "BasicHttpBinding" depending on if DEBUG is set or not.
Update: Because this answer is the highest voted answer to the question, I am updating this answer to clarify an important and tricky point. If you choose to use the ConditionalAttribute
, keep in mind that calls are omitted during compilation, and not runtime. That is:
MyLibrary.dll
[Conditional("DEBUG")]
public void A()
{
Console.WriteLine("A");
B();
}
[Conditional("DEBUG")]
public void B()
{
Console.WriteLine("B");
}
When the library is compiled against release mode (i.e. no DEBUG symbol), it will forever have the call to B()
from within A()
omitted, even if a call to A()
is included because DEBUG is defined in the calling assembly.
First and FirstOrDefault
x |= y 等效于 x = x | y
不同的是 x
只计算一次。 | 运算符对整型操作数执行按位逻辑“或”运算,对布尔操作数执行逻辑“或”运算。
不能直接重载 |=
运算符,但用户定义的类型可以重载 | 运算符
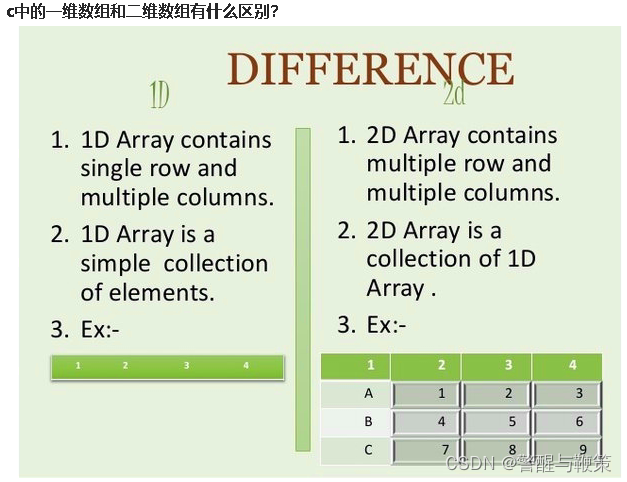
String.PadLeft 方法
返回一个新字符串,该字符串通过在此实例中的字符左侧填充空格来达到指定的总长度,从而实现右对齐。{
private int i = 0;
public A()
{
i += 2;
Debug.LogWarning("A(): "+ i);
}
public A(int a):this()
{
i += a;
Debug.LogWarning("A(int a): " + i);
}
public A(int a, int b) : this(b)
{
i += a;
Debug.LogWarning("A(int a, int b): " + i);
}
public int GetValue()
{
return i;
}
}
Debug.LogWarning(a.GetValue());
{
List<T> list1 = new List<T>();
if (list != null && list.Count > 0)
{
for (int l = 0; l < list.Count; l++)
{
list1.Add(Clone<T>(list[l]));
}
}
return list1;
}
public static T Clone<T>(T RealObject)
{
using (Stream objectStream = new MemoryStream())
{
//利用 System.Runtime.Serialization序列化与反序列化完成引用对象的复制
IFormatter formatter = new BinaryFormatter();
formatter.Serialize(objectStream, RealObject);
objectStream.Seek(0, SeekOrigin.Begin);
return (T)formatter.Deserialize(objectStream);
}
}

public enum SkillType
{
JumpAttack = 0,
FocoAttack,
SwipeAttack,
Skill1,
Appear,
SkillBegin = Skill1,
Skill2,
Skill3,
Skill4,
Skill5
}
skill 1 和 skillBegin 都是3 Appear 是4 skill4 是6
public enum SkillType
{
JumpAttack = 0,
FocoAttack,
SwipeAttack,
Skill1,
SkillBegin = Skill1,
Appear,
Skill2,
Skill3,
Skill4,
Skill5
}
skill 1 和 skillBegin 都是3 Appear 是4 skill4 是7
(1)、C#语法中一个个问号(?)的运算符是指:可以为 null 的类型。
MSDN上面的解释:
在处理数据库和其他包含不可赋值的元素的数据类型时,将 null 赋值给数值类型或布尔型以及日期类型的功能特别有用。例如,数据库中的布尔型字段可以存储值 true 或 false,或者,该字段也可以未定义。
(2)、C#语法中两个问号(??)的运算符是指null 合并运算符,合并运算符为类型转换定义了一个预设值,以防可空类型的值为Null。
MSDN上面的解释:
?? 运算符称为 null 合并运算符,用于定义可以为 null 值的类型和引用类型的默认值。如果此运算符的左操作数不为 null,则此运算符将返回左操作数(左边表达式);否则当左操作数为 null,返回右操作数(右边表达式)。
C# Code:
int? x = null;//定义可空类型变量
int? y = x ?? 1000;//使用合并运算符,当变量x为null时,预设赋值1000
Console.WriteLine(y.ToString()); //1000
/// <summary>
/// Gets a single instance
/// </summary>
public static Log LogInstance
{
get
{
return _log ?? (_log = new Log()); //如果此运算符的左操作数不为 null,则此运算符将返回左操作数;否则返回右操作数。
}
}
1 #region
Enumberable First() or FirstOrDefault()
2 /// <summary>
3 /// 返回序列中的第一个元素;如果序列中不包含任何元素,则返回默认值。
4 /// 如果 source 为空,则返回 default(TSource);否则返回 source 中的第一个元素。
5 /// ArgumentNullException sourcevalue 为 null。
6 /// </summary>
7 public static void FunFirstOrDefault()
8 {
9 //FirstOrDefault()
10 string[] names = { "Haiming QI", "Har", "Adams, Terry", "Andersen, Henriette Thaulow", "Hedlund, Magnus", "Ito, Shu", null };
11 // string[] names = { }; // string 类型的默认值是空
12
13 int[] sexs = { 100, 229, 44, 3, 2, 1 };
14 // int[] sexs = { }; // 因为int 类型的默认值是0. 所以当int[] 数组中没有任何元素时。default value is 0; 如果有元素,则返回第一个元素
15
16 //原方法: public static TSource FirstOrDefault<TSource>(this IEnumerable<TSource> source); // 扩展了IEnumerable<TSource> 接口
17 //string namevalue = names.FirstOrDefault(); // string IEnumerable<string>.FirstOrDefault<string>();
18 int sexvalue = sexs.FirstOrDefault(); // int IEnumerable<int>.FirstOrDefault<string>();
19 //string namevalue = names.DefaultIfEmpty("QI").First();
20 string namevalue = names.FirstOrDefault();
21 Console.WriteLine("FirstOrDefault(): default(TSource) if source is empty; otherwise, the first element in source:{0}", namevalue);
22
23
24 }
25
26 /// <summary>
27 /// 返回序列中的第一个元素。
28 /// 如果 source 中不包含任何元素,则 First<TSource>(IEnumerable<TSource>) 方法将引发异常
29 /// ArgumentNullException sourcevalue 为 null。
30 // InvalidOperationException 源序列为空。
31 /// </summary>
32 public static void FunFirst()
33 {
34 //First()
35 string[] names = { "Haiming QI", "Har", "Adams, Terry", "Andersen, Henriette Thaulow", "Hedlund, Magnus", "Ito, Shu", null };
36 // string[] names = { };
37
38 int[] sexs = { 100, 229, 44, 3, 2, 1 };
39 //int[] sexs = { };
40 int fsex = sexs.First();
41 string fname = names.First(); // 如果序列中没有元素则会发生,InvalidOperationException 异常。 源序列为空。
42
43 Console.WriteLine("First(): Returns the first element of a sequence : {0}", fname);
44
45 }
46 #endregion

以上是我在本地验证的code.
需要注意的是:
这都是扩展了IEnumerable 这个接口。
public static TSource FirstOrDefault<TSource>(this IEnumerable<TSource> source);
First 和 FirstOrDefault 最大的区别在于。 当集合(这个集合可以是:Arry,List,等等)中没有元素的时候。 First 会报异常 InvalidOperationException 源序列为空。
而 FirstOrDefault 则不会。
///
The string class constructor takes an array of characters to create a new string from an array of characters. The following code snippet cre
.Net/C#-Convert char[] to string
char[] chars = {'a', ' ', 's', 't', 'r', 'i', 'n', 'g'};
string s = new string(chars);
char[] chars = {'a', ' ', 's', 't', 'r', 'i', 'n', 'g'};
string s = string.Join("", chars);
//we get "a string"
// or for fun:
string s = string.Join("_", chars);
//we get "a_ _s_t_r_i_n_g"
String mystring = new String(mychararray);
string charToString = new string(CharArray, 0, CharArray.Count());
char[] c = { 'R', 'o', 'c', 'k', '-', '&', '-', 'R', 'o', 'l', 'l' };
string s = String.Concat( c );
Debug.Assert( s.Equals( "Rock-&-Roll" ) );
string 转换成 Char[]
string ss = "abcdefg";
char[] cc = ss.ToCharArray();
Char[] 转换成string
string s = new string(cc);
byte[] 与 string 之间的转换
byte[] bb = Encoding.UTF8.GetBytes(ss);
string s = Encoding.UTF8.GetString(bb);
byte[] 与 char[] 之间的转换
byte[] bb;
char[] cc = Encoding.ASCII.GetChars(bb);
byte[] bb = Encoding.ASCII.GetBytes(cc);
The string class constructor takes an array of characters to create a new string from an array of characters. The following code snippet creates two strings. First from a string and second by direct passing the array in the constructor.
- // Convert char array to string
- char[] chars = new char[10];
- chars[0] = 'M';
- chars[1] = 'a';
- chars[2] = 'h';
- chars[3] = 'e';
- chars[4] = 's';
- chars[5] = 'h';
- string charsStr = new string(chars);
- string charsStr2 = new string(new char[]
- {'T','h','i','s',' ','i','s',' ','a',' ','s','t','r','i','n','g'});
Here is a complete sample code:
- public void StringToCharArray()
- {
- // Convert string to char array
- string sentence = "Mahesh Chand";
- char[] charArr = sentence.ToCharArray();
- foreach (char ch in charArr)
- {
- Console.WriteLine(ch);
- }
- // Convert char array to string
- char[] chars = new char[10];
- chars[0] = 'M';
- chars[1] = 'a';
- chars[2] = 'h';
- chars[3] = 'e';
- chars[4] = 's';
- chars[5] = 'h';
- string charsStr = new string(chars);
- string charsStr2 = new string(new char[]
- {'T','h','i','s',' ','i','s',' ','a',' ','s','t','r','i','n','g'});
- Console.WriteLine("Chars to string: {0}", charsStr);
- Console.WriteLine("Chars to string: {0}", charsStr2);
去掉\0
string b = tempString.Substring(0, length);
string c = tempString.TrimEnd('\0');
string d = System.Text.RegularExpressions.Regex.Replace(tempString, "\0", String.Empty);
string e = tempString.Replace("\0", String.Empty);
//c#清空数组
// Use this for initialization
void Start ()
{
int[] intArray = new int[] { 1, 5, 9, 4 };
Array.Clear(intArray, 0, intArray.Length);//清空第0到第intArray.Length个索引的元素.(包括第0个,不包括第intArray.Length个)
foreach (var item in intArray)
{
Debug.Log(item);
}
}
//
c#计时方法
- //秒表方法一:
- Stopwatch sw = new Stopwatch();
- sw.Start();
- for (int i = 0; i < 10000; i++)
- {
- }
- sw.Stop();
- MessageBox.Show(sw.Elapsed.TotalMilliseconds.ToString());
- //当前时间减去开始时间方法二:
- DateTime begintime = DateTime.Now;
- for (int i = 0; i < 10000; i++)
- {
- }
- TimeSpan ts = DateTime.Now.Subtract(begintime);
- MessageBox.Show(ts.TotalMilliseconds.ToString());
一般md5加密,分为字符串加密和文件加密两种。这里说的加密只是一种不严谨的说法,实际并非加密,只是一种散列算法,其不可逆,即拿到一个md5值不能反向得到源字符串或源文件内容,
#region 1.获得md5值
public static string GetMD5(string msg)
{
StringBuilder sb = new StringBuilder();
using (MD5 md5=MD5.Create())
{
byte[] buffer = Encoding.UTF8.GetBytes(msg);
byte[] newB = md5.ComputeHash(buffer);
foreach (byte item in newB)
{
sb.Append(item.ToString("x2"));
}
}
return sb.ToString();
}
#endregion
#region 2获得一个文件的MD5
public static string GetFileMD5(string filepath)
{
StringBuilder sb = new StringBuilder();
using (MD5 md5=MD5.Create())
{
using (FileStream fs=File.OpenRead(filepath))
{
byte[] newB = md5.ComputeHash(fs);
foreach (byte item in newB)
{
sb.Append(item.ToString("x2"));
}
}
}
return sb.ToString();
}
#endregion
C#MD5加密解密
using System.Security.Cryptography;
using System.IO;
using System.Text;
///MD5加密
public string MD5Encrypt(string pToEncrypt, string sKey)
{
DESCryptoServiceProvider des = new DESCryptoServiceProvider();
byte[] inputByteArray = Encoding.Default.GetBytes(pToEncrypt);
des.Key = ASCIIEncoding.ASCII.GetBytes(sKey);
des.IV = ASCIIEncoding.ASCII.GetBytes(sKey);
MemoryStream ms = new MemoryStream();
CryptoStream cs = new CryptoStream(ms, des.CreateEncryptor(),CryptoStreamMode.Write);
cs.Write(inputByteArray, 0, inputByteArray.Length);
cs.FlushFinalBlock();
StringBuilder ret = new StringBuilder();
foreach(byte b in ms.ToArray())
{
ret.AppendFormat("{0:X2}", b);
}
ret.ToString();
return ret.ToString();
}
///MD5解密
public string MD5Decrypt(string pToDecrypt, string sKey)
{
DESCryptoServiceProvider des = new DESCryptoServiceProvider();
byte[] inputByteArray = new byte[pToDecrypt.Length / 2];
for(int x = 0; x < pToDecrypt.Length / 2; x++)
{
int i = (Convert.ToInt32(pToDecrypt.Substring(x * 2, 2), 16));
inputByteArray[x] = (byte)i;
}
des.Key = ASCIIEncoding.ASCII.GetBytes(sKey);
des.IV = ASCIIEncoding.ASCII.GetBytes(sKey);
MemoryStream ms = new MemoryStream();
CryptoStream cs = new CryptoStream(ms, des.CreateDecryptor(),CryptoStreamMode.Write);
cs.Write(inputByteArray, 0, inputByteArray.Length);
cs.FlushFinalBlock();
StringBuilder ret = new StringBuilder();
return System.Text.Encoding.Default.GetString(ms.ToArray());
}
-------------------------------------------------------------------------------
using System;
using System.Text;
using System.Globalization;
using System.Security.Cryptography;
class DES
{
// 创建Key
public string GenerateKey()
{
DESCryptoServiceProvider desCrypto = (DESCryptoServiceProvider)DESCryptoServiceProvider.Create();
return ASCIIEncoding.ASCII.GetString(desCrypto.Key);
}
// 加密字符串
public string EncryptString(string sInputString, string sKey)
{
byte [] data = Encoding.UTF8.GetBytes(sInputString);
DESCryptoServiceProvider DES = new DESCryptoServiceProvider();
DES.Key = ASCIIEncoding.ASCII.GetBytes(sKey);
DES.IV = ASCIIEncoding.ASCII.GetBytes(sKey);
ICryptoTransform desencrypt = DES.CreateEncryptor();
byte [] result = desencrypt.TransformFinalBlock(data, 0, data.Length);
return BitConverter.ToString(result);
}
// 解密字符串
public string DecryptString(string sInputString, string sKey)
{
string [] sInput = sInputString.Split("-".ToCharArray());
byte [] data = new byte[sInput.Length];
for(int i = 0; i < sInput.Length; i++)
{
data[i] = byte.Parse(sInput[i], NumberStyles.HexNumber);
}
DESCryptoServiceProvider DES = new DESCryptoServiceProvider();
DES.Key = ASCIIEncoding.ASCII.GetBytes(sKey);
DES.IV = ASCIIEncoding.ASCII.GetBytes(sKey);
ICryptoTransform desencrypt = DES.CreateDecryptor();
byte [] result = desencrypt.TransformFinalBlock(data, 0, data.Length);
return Encoding.UTF8.GetString(result);
}
}
class Test
{
static void Main()
{
DES des = new DES();
string key = des.GenerateKey();
string s0 = "中国软件 - csdn.net";
string s1 = des.EncryptString(s0, key);
string s2 = des.DecryptString(s1, key);
Console.WriteLine("原串: [{0}]", s0);
Console.WriteLine("加密: [{0}]", s1);
Console.WriteLine("解密: [{0}]", s2);
}
}
/* 程序输出:
原串: [中国软件 - csdn.net]
加密: [E8-30-D0-F2-2F-66-52-14-45-9A-DC-C5-85-E7-62-9B-AD-B7-82-CF-A8-0A-59-77]
解密: [中国软件 - csdn.net]
*/
从网页上提取用户邮箱为每个邮箱发送一封邮件
WebClient wc = new WebClient();
string html = wc.DownloadString("http://laiba.tianya.cn/tribe/showArticle.jsp?groupId=93803&articleId=255105449041749990113803&curPageNo=1&h=p_1255011420000");
string reg = "[a-zA-Z0-9_\\.]+@[a-zA-Z0-9_\\.]+\\.[a-zA-Z0-9_\\.]+";
MatchCollection matches = Regex.Matches(html, reg);
List<string> listEmail = new List<string>();
foreach (Match mt in matches)
{
listEmail.Add(mt.Groups[0].Value);
}
//------------------以下是创建邮件和发送邮件的过程----------------------
try
{
MailMessage mail = new MailMessage();
mail.From = new MailAddress("****@qq.com");
mail.To.Add("*****@qq.com");
foreach (string email in listEmail)
{
mail.To.Add(email);
}
mail.SubjectEncoding = Encoding.UTF8;
mail.Subject = "测试邮件";
mail.BodyEncoding = Encoding.UTF8;
mail.Body = "c#程序控制控!!!!!!!";
//创建html的邮件内容
AlternateView view = AlternateView.CreateAlternateViewFromString("文字在这里,也可以是<h1>html</h1>的代码<img src=\"cid:img001\" />", Encoding.UTF8, "text/html");
LinkedResource lr = new LinkedResource(@"E:\图片\pics\雷锋.jpg");
lr.ContentId = "img001";
view.LinkedResources.Add(lr);
mail.AlternateViews.Add(view);
//为邮件添加附件
Attachment at = new Attachment(@"D:\项目\chinatt315\members\qiyetupian\batianshengtai01.jpg");
Attachment at1 = new Attachment(@"D:\项目\chinatt315\2011315hd\qytp\piyopiyo2.jpg");
mail.Attachments.Add(at);
mail.Attachments.Add(at1);
SmtpClient smtp = new SmtpClient("pop.qq.com");
smtp.Credentials = new NetworkCredential("用户名", "密码$");
//为每个邮箱发送2封相同的邮件
for (int i = 0; i < 2; i++)
{
smtp.Send(mail);
}
Console.WriteLine("发送成功");
}
catch (Exception ex)
{
Console.WriteLine("发送失败!"+ex.Message);
}
Console.ReadKey();
///
C#实现文件Move和Copy操作
public
static
void
MoveFolder(
string
sourcePath,
string
destPath)
{
if
(Directory.Exists(sourcePath))
{
if
(!Directory.Exists(destPath))
{
//目标目录不存在则创建
try
{
Directory.CreateDirectory(destPath);
}
catch
(Exception ex)
{
throw
new
Exception(
"创建目标目录失败:"
+ ex.Message);
}
}
//获得源文件下所有文件
List<
string
> files =
new
List<
string
>(Directory.GetFiles(sourcePath));
files.ForEach(c =>
{
string
destFile = Path.Combine(
new
string
[] { destPath, Path.GetFileName(c) });
//覆盖模式
if
(File.Exists(destFile))
{
File.Delete(destFile);
}
File.Move(c, destFile);
});
//获得源文件下所有目录文件
List<
string
> folders =
new
List<
string
>(Directory.GetDirectories(sourcePath));
folders.ForEach(c =>
{
string
destDir = Path.Combine(
new
string
[] { destPath, Path.GetFileName(c) });
//Directory.Move必须要在同一个根目录下移动才有效,不能在不同卷中移动。
//Directory.Move(c, destDir);
//采用递归的方法实现
MoveFolder(c, destDir);
});
}
else
{}
}
public
static
void
CopyFilefolder(
string
sourceFilePath,
string
targetFilePath)
{
//获取源文件夹中的所有非目录文件
string
[] files = Directory.GetFiles(sourceFilePath);
string
fileName;
string
destFile;
//如果目标文件夹不存在,则新建目标文件夹
if
(!Directory.Exists(targetFilePath))
{
Directory.CreateDirectory(targetFilePath);
}
//将获取到的文件一个一个拷贝到目标文件夹中
foreach
(
string
s
in
files)
{
fileName = Path.GetFileName(s);
destFile = Path.Combine(targetFilePath, fileName);
File.Copy(s, destFile,
true
);
}
//上面一段在MSDN上可以看到源码
//获取并存储源文件夹中的文件夹名
string
[] filefolders = Directory.GetFiles(sourceFilePath);
//创建Directoryinfo实例
DirectoryInfo dirinfo =
new
DirectoryInfo(sourceFilePath);
//获取得源文件夹下的所有子文件夹名
DirectoryInfo[] subFileFolder = dirinfo.GetDirectories();
for
(
int
j = 0; j < subFileFolder.Length; j++)
{
//获取所有子文件夹名
string
subSourcePath = sourceFilePath +
"\\"
+ subFileFolder[j].ToString();
string
subTargetPath = targetFilePath +
"\\"
+ subFileFolder[j].ToString();
//把得到的子文件夹当成新的源文件夹,递归调用CopyFilefolder
CopyFilefolder(subSourcePath, subTargetPath);
}
}
C# 复制文件及文件夹
/// <summary>
/// 复制文件夹及文件
/// </summary>
/// <param name="sourceFolder">原文件路径</param>
/// <param name="destFolder">目标文件路径</param>
/// <returns></returns>
public static int CopyFolder(string sourceFolder, string destFolder)
{
try
{
//如果目标路径不存在,则创建目标路径
if (!System.IO.Directory.Exists(destFolder))
{
System.IO.Directory.CreateDirectory(destFolder);
}
//得到原文件根目录下的所有文件
string[] files = System.IO.Directory.GetFiles(sourceFolder);
foreach (string file in files)
{
string name = System.IO.Path.GetFileName(file);
string dest = System.IO.Path.Combine(destFolder, name);
System.IO.File.Copy(file, dest);//复制文件
}
//得到原文件根目录下的所有文件夹
string[] folders = System.IO.Directory.GetDirectories(sourceFolder);
foreach (string folder in folders)
{
string name = System.IO.Path.GetFileName(folder);
string dest = System.IO.Path.Combine(destFolder, name);
CopyFolder(folder, dest);//构建目标路径,递归复制文件
}
return 1;
}
catch (Exception e)
{
return -1;
}
}
private void CopyDirectory(string srcdir, string desdir)
{
if (srcdir.LastIndexOf("\\") == (srcdir.Length - 1))
{
srcdir = srcdir.Substring(0, srcdir.Length - 1);
}
string folderName = srcdir.Substring(srcdir.LastIndexOf("\\") + 1);
string desfolderdir = "";
if (desdir.LastIndexOf("\\") == (desdir.Length - 1))
{
desfolderdir = desdir + folderName;
}
else
{
desfolderdir = desdir + "\\" + folderName;
}
string[] filenames = Directory.GetFileSystemEntries(srcdir);
foreach (string file in filenames)// 遍历所有的文件和目录
{
if (Directory.Exists(file))// 先当作目录处理如果存在这个目录就递归Copy该目录下面的文件
{
string currentdir = desfolderdir + "\\" + file.Substring(file.LastIndexOf("\\") + 1);
if (!Directory.Exists(currentdir))
{
Directory.CreateDirectory(currentdir);
}
CopyDirectory(file, desfolderdir);
}
else // 否则直接copy文件
{
string srcfileName = file.Substring(file.LastIndexOf("\\") + 1);
srcfileName = desfolderdir + "\\" + srcfileName;
if (!Directory.Exists(desfolderdir))
{
Directory.CreateDirectory(desfolderdir);
}
File.Copy(file, srcfileName, true);
}
}
}
FileUtil.MoveFileOrDirectory(fileSrc, dirDst); windows 路径 file 后缀
/// <summary>
/// 拷贝文件到另一个文件夹下
/// </summary>
/// <param name="sourceName">源文件路径</param>
/// <param name="folderPath">目标路径(目标文件夹)</param>
public void CopyToFile(string sourceName, string folderPath)
{
//例子:
//源文件路径
//string sourceName = @"D:\Source\Test.txt";
//目标路径:项目下的NewTest文件夹,(如果没有就创建该文件夹)
//string folderPath = Path.Combine(AppDomain.CurrentDomain.BaseDirectory, "NewTest");
if (!Directory.Exists(folderPath))
{
Directory.CreateDirectory(folderPath);
}
//当前文件如果不用新的文件名,那么就用原文件文件名
string fileName = Path.GetFileName(sourceName);
//这里可以给文件换个新名字,如下:
//string fileName = string.Format("{0}.{1}", "newFileText", "txt");
//目标整体路径
string targetPath = Path.Combine(folderPath, fileName);
//Copy到新文件下
FileInfo file = new FileInfo(sourceName);
if (file.Exists)
{
//true 为覆盖已存在的同名文件,false 为不覆盖
file.CopyTo(targetPath, true);
}
}
源目录的文件,目标目录的文件
FileStream实现大文件复制
public
static
class
FileHelper
{
/// <summary>
/// 复制大文件
/// </summary>
/// <param name="fromPath">源文件的路径</param>
/// <param name="toPath">文件保存的路径</param>
/// <param name="eachReadLength">每次读取的长度</param>
/// <returns>是否复制成功</returns>
public
static
bool
CopyFile(
string
fromPath,
string
toPath,
int
eachReadLength)
{
//将源文件 读取成文件流
FileStream fromFile =
new
FileStream(fromPath, FileMode.Open, FileAccess.Read);
//已追加的方式 写入文件流
FileStream toFile =
new
FileStream(toPath, FileMode.Append, FileAccess.Write);
//实际读取的文件长度
int
toCopyLength = 0;
//如果每次读取的长度小于 源文件的长度 分段读取
if
(eachReadLength < fromFile.Length)
{
byte
[] buffer =
new
byte
[eachReadLength];
long
copied = 0;
while
(copied <= fromFile.Length - eachReadLength)
{
toCopyLength = fromFile.Read(buffer, 0, eachReadLength);
fromFile.Flush();
toFile.Write(buffer, 0, eachReadLength);
toFile.Flush();
//流的当前位置
toFile.Position = fromFile.Position;
copied += toCopyLength;
}
int
left = (
int
)(fromFile.Length - copied);
toCopyLength = fromFile.Read(buffer, 0, left);
fromFile.Flush();
toFile.Write(buffer, 0, left);
toFile.Flush();
}
else
{
//如果每次拷贝的文件长度大于源文件的长度 则将实际文件长度直接拷贝
byte
[] buffer =
new
byte
[fromFile.Length];
fromFile.Read(buffer, 0, buffer.Length);
fromFile.Flush();
toFile.Write(buffer, 0, buffer.Length);
toFile.Flush();
}
fromFile.Close();
toFile.Close();
return
true
;
}
}
class
Program
{
static
void
Main(
string
[] args)
{
Stopwatch watch =
new
Stopwatch();
watch.Start();
if
(FileHelper.CopyFile(
@"D:\安装文件\新建文件夹\SQLSVRENT_2008R2_CHS.iso"
,
@"F:\SQLSVRENT_2008R2_CHS.iso"
, 1024 * 1024 * 5))
{
watch.Stop();
Console.WriteLine(
"拷贝完成,耗时:"
+ watch.Elapsed.Seconds+
"秒"
);
}
Console.Read();
}
} // 4.3G 25s完成
C#进行文件读写、创建、复制、移动、删除的方法
1.文件夹创建、移动、删除
//创建文件夹
Directory.CreateDirectory(Server.MapPath(
"a"
));
Directory.CreateDirectory(Server.MapPath(
"b"
));
Directory.CreateDirectory(Server.MapPath(
"c"
));
//移动b到a
Directory.Move(Server.MapPath(
"b"
), Server.MapPath(
"a//b"
));
//删除c
Directory.Delete(Server.MapPath(
"c"
));
2.文件创建、复制、移动、删除
//创建文件
//使用File.Create创建再复制/移动/删除时会提示:
//文件正由另一进程使用,因此该进程无法访问该文件
//改用 FileStream 获取 File.Create
//返回的 System.IO.FileStream再进行关闭就无此问题
FileStream fs;
fs = File.Create(Server.MapPath(
"a.txt"
));
fs.Close();
fs = File.Create(Server.MapPath(
"b.txt"
));
fs.Close();
fs = File.Create(Server.MapPath(
"c.txt"
));
fs.Close();
//复制文件
File.Copy(Server.MapPath(
"a.txt"
),Server.MapPath(
"a//a.txt"
));
//移动文件
File.Move(Server.MapPath(
"b.txt"
),Server.MapPath(
"a//b.txt"
));
File.Move(Server.MapPath(
"c.txt"
),Server.MapPath(
"a//c.txt"
));
//删除文件
File.Delete(Server.MapPath(
"a.txt"
));
3.遍历文件夹中的文件和子文件夹并显示其属性
if
(Directory.Exists(Server.MapPath(
"a"
)))
{
//所有子文件夹
foreach
(
string
item inDirectory.GetDirectories(Server.MapPath(
"a"
)))
{
Response.Write(
"<b>文件夹:"
+ item +
"</b><br/>"
);
DirectoryInfo directoryinfo =
new
DirectoryInfo(item);
Response.Write(
"名称:"
+directoryinfo.Name +
"<br/>"
);
Response.Write(
"路径:"
+directoryinfo.FullName +
"<br/>"
);
Response.Write(
"创建时间:"
+directoryinfo.CreationTime +
"<br/>"
);
Response.Write(
"上次访问时间:"
+directoryinfo.LastAccessTime+
"<br/>"
);
Response.Write(
"上次修改时间:"
+directoryinfo.LastWriteTime+
"<br/>"
);
Response.Write(
"父文件夹:"
+directoryinfo.Parent+
"<br/>"
);
Response.Write(
"所在根目录:"
+directoryinfo.Root+
"<br/>"
);
Response.Write(
"<br/>"
);
}
//所有子文件
foreach
(
string
item inDirectory.GetFiles(Server.MapPath(
"a"
)))
{
Response.Write(
"<b>文件:"
+ item +
"</b><br/>"
);
FileInfo fileinfo =
new
FileInfo(item);
Response.Write(
"名称:"
+ fileinfo.Name +
"<br/>"
);
Response.Write(
"扩展名:"
+ fileinfo.Extension+
"<br/>"
);
Response.Write(
"路径:"
+ fileinfo.FullName+
"<br/>"
);
Response.Write(
"大小:"
+ fileinfo.Length+
"<br/>"
);
Response.Write(
"创建时间:"
+ fileinfo.CreationTime+
"<br/>"
);
Response.Write(
"上次访问时间:"
+ fileinfo.LastAccessTime+
"<br/>"
);
Response.Write(
"上次修改时间:"
+ fileinfo.LastWriteTime+
"<br/>"
);
Response.Write(
"所在文件夹:"
+ fileinfo.DirectoryName+
"<br/>"
);
Response.Write(
"文件属性:"
+ fileinfo.Attributes+
"<br/>"
);
Response.Write(
"<br/>"
);
}
}
4.文件读写
if
(File.Exists(Server.MapPath(
"a//a.txt"
)))
{
StreamWriter streamwrite = newStreamWriter(Server.MapPath(
"a//a.txt"
));
streamwrite.WriteLine(
"木子屋"
);
streamwrite.WriteLine(
"https://www.jb51.net/"
);
streamwrite.Write(
"2008-04-13"
);
streamwrite.Close();
StreamReader streamreader = newStreamReader(Server.MapPath(
"a//a.txt"
));
Response.Write(streamreader.ReadLine());
Response.Write(streamreader.ReadToEnd());
streamreader.Close();
}
/
C#中绝对路径获取:
在C#中可以通过下面的方式获取绝对路径,不过获取的绝对路径是C#项目运行后生成的exe可执行文件的绝对路径。
//第一种方式
string path = Directory.GetCurrentDirectory();
//第二种方式
string startuppath = Application.StartupPath; //获取的路径为exe文件所在的路径
两种方式获取的路径如下图所示:
获取上级目录
通过上面方法获取绝对路径之后可以通过代码获取上下级的相对路径,
第一种
DirectoryInfo path = new DirectoryInfo(Application.StartupPath);
textBox3.Text = path.Parent.Parent.FullName;//上 2层目录
在FullName前面添加几个“.Parent”就跳到当前目录的上几级目录。如图所示
第二种
DirectoryInfo path = new DirectoryInfo(string.Format(@"{0}..\..\..\", Application.StartupPath));//上两层目录
textBox3.Text = path.FullName;
这种方式也是可以跳到任意前面几级目录,跳到几级目录取决于“…\”的个数,代码中的方式可以跳到前面两级目录。如下图所示:
第三种
string Path = Application.StartupPath.Substring(0, Application.StartupPath.LastIndexOf(@"\"));//上一层目录
textBox3.Text = Path;
这种方式可以跳到上一级目录。
///
C#有个函数Sort()
,默认是按升序排序,可直接应用于对List
集合的排序。
示例
- using System;
- using System.Collections.Generic;
- class Program
- {
- static void Main()
- {
- List<int> numbers = new List<int>() { 20, 10, 3, 40 };
- numbers.Sort();
- Console.WriteLine(":::按升序排序:::");
- foreach (var result in numbers)
- {
- Console.WriteLine(result);
- }
- }
- }
输出结果
按升序排序:::
- 3
- 10
- 20
- 40
要让Sort()
按降序排序,要用到CompareTo()
比较函数。
C#中的List的Sort
函数中的比较函数CompareTo
有三种结果 1, -1 ,0分别代表大,小,相等。默认List
的排序是升序排序。
在比较函数CompareTo()
中,如果 x>y return 1;
则是按照升序排列。如果x>y return -1;
则是按照降序排列。这就是1和-1大小的含义。其实你非要这么写 x<y return 1;
则也表示降序排列。不过大家一般习惯x>y return 1;
升序,如果想要降序只需return -1;即可。
系统List默认的排序是升序,如果你想要降序,可以直接在比较函数前面加个负号,把返回结果由1变成-1即可。
示例
- using System;
- using System.Collections.Generic;
- class Program
- {
- static void Main()
- {
- List<int> numbers = new List<int>() { 20, 10, 3, 40 };
- numbers.Sort((x, y) => -x.CompareTo(y));
- Console.WriteLine(":::按降序排序:::");
- foreach (var result in numbers)
- {
- Console.WriteLine(result);
- }
- }
- }
输出结果
按降序排序:::
- 40
- 20
- 10
- 3
需要按数字的第一位排序时,又该怎样写这个代码呢?
其实,我们只需把集合的元素第一位先转化为字符,再进行排序,即可。
示例
- using System;
- using System.Collections.Generic;
- class Program
- {
- static void Main()
- {
- List<int> numbers = new List<int>() { 20, 10, 3, 40 };
- numbers.Sort((a, b) => (a.ToString()[0].CompareTo(b.ToString()[0])));
- Console.WriteLine(":::按数字的第一位排序:::");
- foreach (var result in numbers)
- {
- Console.WriteLine(result);
- }
- }
- }
输出结果
按数字的第一位排序:::
- 10
- 20
- 3
- 40
C# 默认排序方法Sort、Reverse
排序Sort,倒序Reverse
//默认是元素第一个字母按升序
list.Sort();
//将List里面元素顺序反转
list.Reverse();
//从第二个元素开始,反转4个元素
//结果list里最后的顺序变成"Ha", "Jay", "Lily", "Tom", "Hunter", "Jim", "Kuku", "Locu"
list.Reverse(1,4);
C#自定义排序的4种方法
List<T>.Sort();
List<T>.Sort(IComparer<T> Comparer);
List<T>.Sort(int index, int count, IComparer<T> Comparer);
List<T>.Sort(Comparison<T> comparison);
1
2
3
4
实现目标
假设存在一个People类,包含Name、Age属性,在客户端中创建List保存多个实例,希望对List中的内容根据Name和Age参数进行排序,排序规则为,先按姓名升序排序,如果姓名相同再按年龄的升序排序:
class People
{
public People(string name, int age) { Name = name; Age = age; }
public string Name { get; set; } //姓名
public int Age { get; set; } //年龄
}
// 客户端
class Client
{
static void Main(string[] args)
{
List<People> peopleList = new List<People>();
peopleList.Add(new People("张三", 22));
peopleList.Add(new People("张三", 24));
peopleList.Add(new People("李四", 18));
peopleList.Add(new People("王五", 16));
peopleList.Add(new People("王五", 30));
}
}
方法一、继承IComparable接口,实现CompareTo()方法
对People类继承IComparable接口,实现CompareTo()方法
该方法为系统默认的方法,单一参数时会默认进行升序排序。但遇到多参数(Name、Age)排序时,我们需要对该默认方法进行修改。
方法一:People类继承IComparable接口,实现CompareTo()方法
IComparable:定义由值类型或类实现的通用比较方法,旨在创建特定于类型的比较方法以对实例进行排序。
原理:自行实现的CompareTo()方法会在list.Sort()内部进行元素两两比较,最终实现排序
class People : IComparable<People>
{
public People(string name, int age) { Name = name;Age = age; }
public string Name { get; set; }
public int Age { get; set; }
// list.Sort()时会根据该CompareTo()进行自定义比较
public int CompareTo(People other)
{
if (this.Name != other.Name)
{
return this.Name.CompareTo(other.Name);
}
else if (this.Age != other.Age)
{
return this.Age.CompareTo(other.Age);
}
else return 0;
}
}
// 客户端
peopleList.Sort();
// OUTPUT:
// 李四 18
// 王五 16
// 王五 30
// 张三 22
// 张三 24
方法二:增加外部比较类,继承IComparer接口、实现Compare()方法
增加People类的外部比较类,继承IComparer接口、实现Compare()方法
区别于上述继承IComparable的方法,该方法不可在People内继承实现IComparer接口,而是需要新建比较方法类进行接口实现
方法二:新建PeopleComparer类、继承IComparer接口、实现Compare()方法
原理:list.Sort()将PeopleComparer类的实例作为参数,在内部使用Compare()方法进行两两比较,最终实现排序(注:上述方法为CompareTo(),此处为Compare()方法)
// 自定义比较方法类
class PeopleComparer : IComparer<People>
{
// 区别于CompareTo()单参数,此处为双参数
public int Compare(People x, People y)
{
if (x.Name != y.Name)
{
return x.Name.CompareTo(y.Name);
}
else if (x.Age != y.Age)
{
return x.Age.CompareTo(y.Age);
}
else return 0;
}
}
// 客户端
// 传入参数为自定义比较类的实例
peopleList.Sort(new PeopleComparer());
// OUTPUT:
// 李四 18
// 王五 16
// 王五 30
// 张三 22
// 张三 24
同理,List.Sort(int index, int count, IComparer Comparer) 方法的参数:待排元素起始索引、待排元素个数、排序方法
方法三、采用泛型委托 Comparison,绑定自定义的比较方法
区别于上述继承接口的方法,此方法的参数为 泛型委托 Comparison
委托原型:public delegate int Comparison(T x, T y);
方法三:依照委托的使用方法,首先创建委托实例MyComparison,并绑定到自定义的比较方法PeopleComparison()上,最终调用list.Sort()时 将委托实例传入
原理:list.Sort()根据传入的委托方法,进行两两元素比较最终实现排序
// 客户端
class Client
{
// 方法0 自定义比较方法
public static int PeopleComparison(People p1, People p2)
{
if (p1.Name != p2.Name)
{
return p1.Name.CompareTo(p2.Name);
}
else if (p1.Age != p2.Age)
{
return p1.Age.CompareTo(p2.Age);
}
else return 0;
}
static void Main(string[] args)
{
/ 创建list ... /
// 方法0 创建委托实例并绑定
Comparison<People> MyComparison = PeopleComparison;
// 传入该实例实现比较方法
peopleList.Sort(MyComparison);
// OUTPUT:
// 李四 18
// 王五 16
// 王五 30
// 张三 22
// 张三 24
}
}
泛型委托用 Lambda表达式
此外,既然Comparison是泛型委托,则完全可以用 Lambda表达式 进行描述:
// Lambda表达式实现Comparison委托
peopleList.Sort((p1, p2) =>
{
if (p1.Name != p2.Name)
{
return p2.Name.CompareTo(p1.Name);
}
else if (p1.Age != p2.Age)
{
return p2.Age.CompareTo(p1.Age);
}
else return 0;
});
// OUTPUT:
// 张三 24
// 张三 22
// 王五 30
// 王五 16
// 李四 18
虽然本文仅使用了List一种容器对Sort()方法进行阐述,但是不同容器的使用Sort()的方法大相径庭,因为核心的原理都是应用两种接口及泛型委托:
两种接口:IComparable 、 IComparer
泛型委托:Comparison