//#define USE_TESTCONSOLE
using System.Collections.Generic;
using UnityEngine;
namespace Consolation
{
/// <summary>
/// A console to display Unity's debug logs in-game.
/// </summary>
class TestConsole : MonoBehaviour
{
#if USE_TESTCONSOLE
struct Log
{
public string message;
public string stackTrace;
public LogType type;
}
#region Inspector Settings
/// <summary>
/// The hotkey to show and hide the console window.
/// </summary>
public KeyCode toggleKey = KeyCode.BackQuote;
/// <summary>
/// Whether to open the window by shaking the device (mobile-only).
/// </summary>
public bool shakeToOpen = true;
/// <summary>
/// The (squared) acceleration above which the window should open.
/// </summary>
public float shakeAcceleration = 3f;
/// <summary>
/// Whether to only keep a certain number of logs.
///
/// Setting this can be helpful if memory usage is a concern.
/// </summary>
public bool restrictLogCount = false;
/// <summary>
/// Number of logs to keep before removing old ones.
/// </summary>
public int maxLogs = 1000;
#endregion
readonly List<Log> logs = new List<Log>();
Vector2 scrollPosition;
bool visible;
bool collapse;
// Visual elements:
static readonly Dictionary<LogType, Color> logTypeColors = new Dictionary<LogType, Color>
{
{ LogType.Assert, Color.white },
{ LogType.Error, Color.red },
{ LogType.Exception, Color.red },
{ LogType.Log, Color.white },
{ LogType.Warning, Color.yellow },
};
const string windowTitle = "Console";
const int margin = 20;
static readonly GUIContent clearLabel = new GUIContent("Clear", "Clear the contents of the console.");
static readonly GUIContent collapseLabel = new GUIContent("Collapse", "Hide repeated messages.");
readonly Rect titleBarRect = new Rect(0, 0, 10000, 20);
Rect windowRect = new Rect(margin, margin, Screen.width - (margin * 2), Screen.height - (margin * 2));
void OnEnable()
{
#if UNITY_5
Application.logMessageReceived += HandleLog;
#else
Application.RegisterLogCallback(HandleLog);
#endif
}
void OnDisable()
{
#if UNITY_5
Application.logMessageReceived -= HandleLog;
#else
Application.RegisterLogCallback(null);
#endif
}
void Update()
{
if (Input.GetKeyDown(toggleKey))
{
visible = !visible;
}
if (shakeToOpen && Input.acceleration.sqrMagnitude > shakeAcceleration)
{
visible = true;
}
}
void OnGUI()
{
if (!visible)
{
return;
}
windowRect = GUILayout.Window(123456, windowRect, DrawConsoleWindow, windowTitle);
}
/// <summary>
/// Displays a window that lists the recorded logs.
/// </summary>
/// <param name="windowID">Window ID.</param>
void DrawConsoleWindow(int windowID)
{
DrawLogsList();
DrawToolbar();
// Allow the window to be dragged by its title bar.
GUI.DragWindow(titleBarRect);
}
/// <summary>
/// Displays a scrollable list of logs.
/// </summary>
void DrawLogsList()
{
scrollPosition = GUILayout.BeginScrollView(scrollPosition);
// Iterate through the recorded logs.
for (var i = 0; i < logs.Count; i++)
{
var log = logs[i];
// Combine identical messages if collapse option is chosen.
if (collapse && i > 0)
{
var previousMessage = logs[i - 1].message;
if (log.message == previousMessage)
{
continue;
}
}
GUI.contentColor = logTypeColors[log.type];
GUILayout.Label(log.message);
}
GUILayout.EndScrollView();
// Ensure GUI colour is reset before drawing other components.
GUI.contentColor = Color.white;
}
/// <summary>
/// Displays options for filtering and changing the logs list.
/// </summary>
void DrawToolbar()
{
GUILayout.BeginHorizontal();
if (GUILayout.Button(clearLabel))
{
logs.Clear();
}
collapse = GUILayout.Toggle(collapse, collapseLabel, GUILayout.ExpandWidth(false));
GUILayout.EndHorizontal();
}
/// <summary>
/// Records a log from the log callback.
/// </summary>
/// <param name="message">Message.</param>
/// <param name="stackTrace">Trace of where the message came from.</param>
/// <param name="type">Type of message (error, exception, warning, assert).</param>
void HandleLog(string message, string stackTrace, LogType type)
{
logs.Add(new Log
{
message = message,
stackTrace = stackTrace,
type = type,
});
TrimExcessLogs();
}
/// <summary>
/// Removes old logs that exceed the maximum number allowed.
/// </summary>
void TrimExcessLogs()
{
if (!restrictLogCount)
{
return;
}
var amountToRemove = Mathf.Max(logs.Count - maxLogs, 0);
if (amountToRemove == 0)
{
return;
}
logs.RemoveRange(0, amountToRemove);
}
#endif
}
然后,你还需要一个控制台窗口
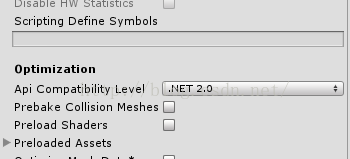
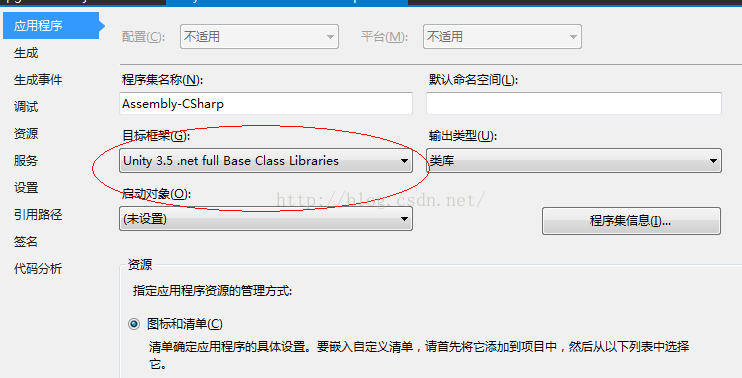
同样,我写了代码屏蔽的宏定义。使用的时候启用就可以了。
using System.Collections.Generic;
using UnityEngine;
namespace Consolation
{
/// <summary>
/// A console to display Unity's debug logs in-game.
/// </summary>
class TestConsole : MonoBehaviour
{
#if USE_TESTCONSOLE
struct Log
{
public string message;
public string stackTrace;
public LogType type;
}
#region Inspector Settings
/// <summary>
/// The hotkey to show and hide the console window.
/// </summary>
public KeyCode toggleKey = KeyCode.BackQuote;
/// <summary>
/// Whether to open the window by shaking the device (mobile-only).
/// </summary>
public bool shakeToOpen = true;
/// <summary>
/// The (squared) acceleration above which the window should open.
/// </summary>
public float shakeAcceleration = 3f;
/// <summary>
/// Whether to only keep a certain number of logs.
///
/// Setting this can be helpful if memory usage is a concern.
/// </summary>
public bool restrictLogCount = false;
/// <summary>
/// Number of logs to keep before removing old ones.
/// </summary>
public int maxLogs = 1000;
#endregion
readonly List<Log> logs = new List<Log>();
Vector2 scrollPosition;
bool visible;
bool collapse;
// Visual elements:
static readonly Dictionary<LogType, Color> logTypeColors = new Dictionary<LogType, Color>
{
{ LogType.Assert, Color.white },
{ LogType.Error, Color.red },
{ LogType.Exception, Color.red },
{ LogType.Log, Color.white },
{ LogType.Warning, Color.yellow },
};
const string windowTitle = "Console";
const int margin = 20;
static readonly GUIContent clearLabel = new GUIContent("Clear", "Clear the contents of the console.");
static readonly GUIContent collapseLabel = new GUIContent("Collapse", "Hide repeated messages.");
readonly Rect titleBarRect = new Rect(0, 0, 10000, 20);
Rect windowRect = new Rect(margin, margin, Screen.width - (margin * 2), Screen.height - (margin * 2));
void OnEnable()
{
#if UNITY_5
Application.logMessageReceived += HandleLog;
#else
Application.RegisterLogCallback(HandleLog);
#endif
}
void OnDisable()
{
#if UNITY_5
Application.logMessageReceived -= HandleLog;
#else
Application.RegisterLogCallback(null);
#endif
}
void Update()
{
if (Input.GetKeyDown(toggleKey))
{
visible = !visible;
}
if (shakeToOpen && Input.acceleration.sqrMagnitude > shakeAcceleration)
{
visible = true;
}
}
void OnGUI()
{
if (!visible)
{
return;
}
windowRect = GUILayout.Window(123456, windowRect, DrawConsoleWindow, windowTitle);
}
/// <summary>
/// Displays a window that lists the recorded logs.
/// </summary>
/// <param name="windowID">Window ID.</param>
void DrawConsoleWindow(int windowID)
{
DrawLogsList();
DrawToolbar();
// Allow the window to be dragged by its title bar.
GUI.DragWindow(titleBarRect);
}
/// <summary>
/// Displays a scrollable list of logs.
/// </summary>
void DrawLogsList()
{
scrollPosition = GUILayout.BeginScrollView(scrollPosition);
// Iterate through the recorded logs.
for (var i = 0; i < logs.Count; i++)
{
var log = logs[i];
// Combine identical messages if collapse option is chosen.
if (collapse && i > 0)
{
var previousMessage = logs[i - 1].message;
if (log.message == previousMessage)
{
continue;
}
}
GUI.contentColor = logTypeColors[log.type];
GUILayout.Label(log.message);
}
GUILayout.EndScrollView();
// Ensure GUI colour is reset before drawing other components.
GUI.contentColor = Color.white;
}
/// <summary>
/// Displays options for filtering and changing the logs list.
/// </summary>
void DrawToolbar()
{
GUILayout.BeginHorizontal();
if (GUILayout.Button(clearLabel))
{
logs.Clear();
}
collapse = GUILayout.Toggle(collapse, collapseLabel, GUILayout.ExpandWidth(false));
GUILayout.EndHorizontal();
}
/// <summary>
/// Records a log from the log callback.
/// </summary>
/// <param name="message">Message.</param>
/// <param name="stackTrace">Trace of where the message came from.</param>
/// <param name="type">Type of message (error, exception, warning, assert).</param>
void HandleLog(string message, string stackTrace, LogType type)
{
logs.Add(new Log
{
message = message,
stackTrace = stackTrace,
type = type,
});
TrimExcessLogs();
}
/// <summary>
/// Removes old logs that exceed the maximum number allowed.
/// </summary>
void TrimExcessLogs()
{
if (!restrictLogCount)
{
return;
}
var amountToRemove = Mathf.Max(logs.Count - maxLogs, 0);
if (amountToRemove == 0)
{
return;
}
logs.RemoveRange(0, amountToRemove);
}
#endif
}
}
随便写个C#代码,在Update中测试的。
直接写在屏幕上的代码
代码是歪果仁写的,但是很好用。
首先,输入的代码:
名字为ConsoleInput.cs
如下:
当你试图编译代码时候,你会发现居然编译报错,各种找不到。
Console.CursorLeft等大量的方法和变量都找不到。
这是因为Untiy5 默认的目标框架Unity3.5 .net sbu base class Libraries.。在Player Setting中,可设置为.Net 2.0。
这就可以改变了VS下的编译环境了。
但是要是你想要修改编译环境为你想要的其他呢?
四、那么问题来了?怎么修改编译环境的目标框架呢?
你肯定不会是想出这个问题的第一人?所以那就有人来解决问题;
动态的修改你的FrameWork,就可以解答这个问题。
代码:UpgradeVSProject.cs
同样,我写了代码屏蔽的宏定义。使用的时候启用就可以了。
就可以自动升高版本到4.0上,当然你也可以修改代码来升高到其他版本的。
注意:这个代码很明显,需要放置在Editor文件夹下。
五、测试结果
我写了个几行的测试代码: