unity 《专题系列》资源打包 Asset Bundles
第三节 Asset Bundles 加载
一、AssetBundle的加载常用的的几种方式
1、AssetBundle.LoadFromMemoryAsync;
2、AssetBundle.LoadFromFile;
3、WWW.LoadFromCacheOrDownload (注意可能会逐渐弃用掉);
4、UnityWebRequest;
二、AssetBundle的加载
1、新建一个场景LoadAssetBundleScene,并且在MainCamera上新建个脚本,如下图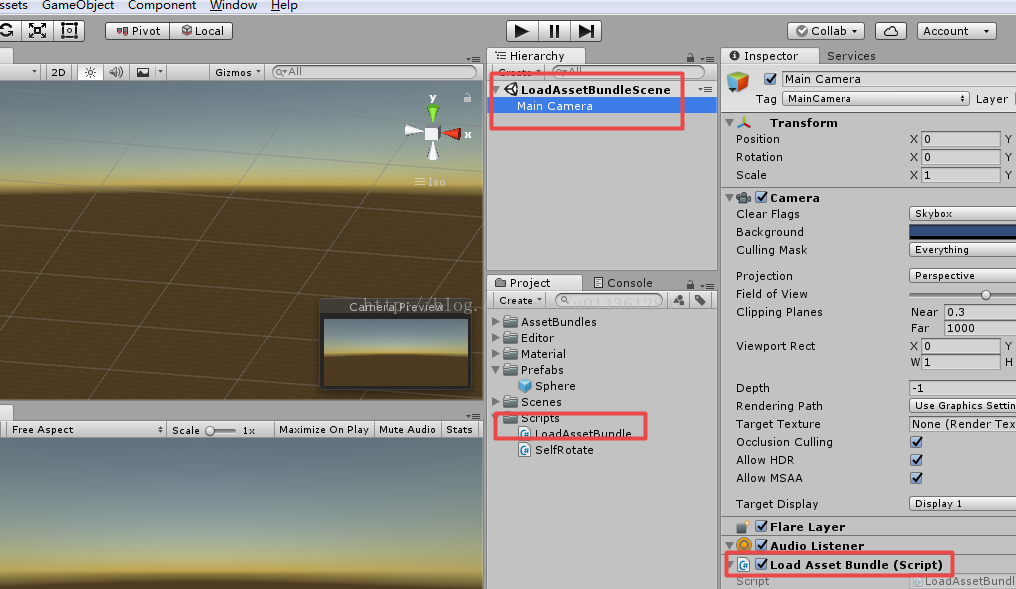
2.1、AssetBundle.LoadFromMemoryAsync 方法加载,在脚本中添加如下代码,运行结果,如下图
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using System.IO;
public class LoadAssetBundle : MonoBehaviour {
// Use this for initialization
IEnumerator Start () {
//1.1 LoadFromMemoryAsync 方法加载
//AssetBundle的存放路径
string path = "Assets/AssetBundles/sphereab.unityab";
AssetBundleCreateRequest request = AssetBundle.LoadFromMemoryAsync(File.ReadAllBytes(path));
//等待异步加载请求完成
yield return request;
AssetBundle ab = request.assetBundle;
//取得所需要的资源
GameObject go = ab.LoadAsset<GameObject>("Sphere");
//加载生成到场景中
Instantiate(go);
}
}
2.2、AssetBundle.LoadFromMemory 方法加载,在脚本中添加如下代码,运行结果,如下图using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using System.IO;
public class LoadAssetBundle : MonoBehaviour {
// Use this for initialization
void Start () {
//1.2 LoadFromMemory 方法加载
//AssetBundle的存放路径
string path = "Assets/AssetBundles/sphereab.unityab";
AssetBundle ab = AssetBundle.LoadFromMemory(File.ReadAllBytes(path));
//取得所需要的资源
GameObject go = ab.LoadAsset<GameObject>("Sphere");
//加载生成到场景中
Instantiate(go);
}
}
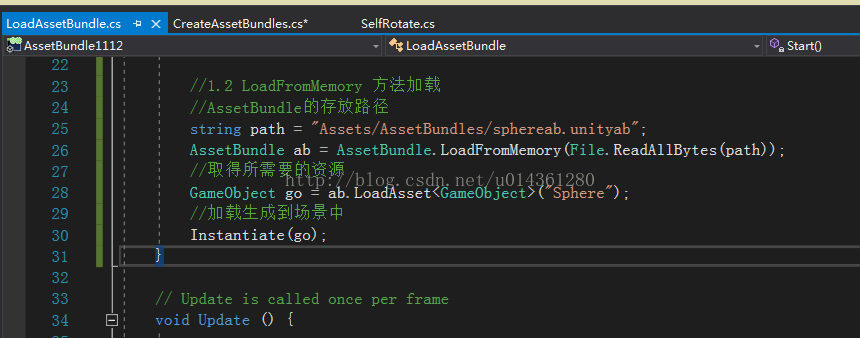
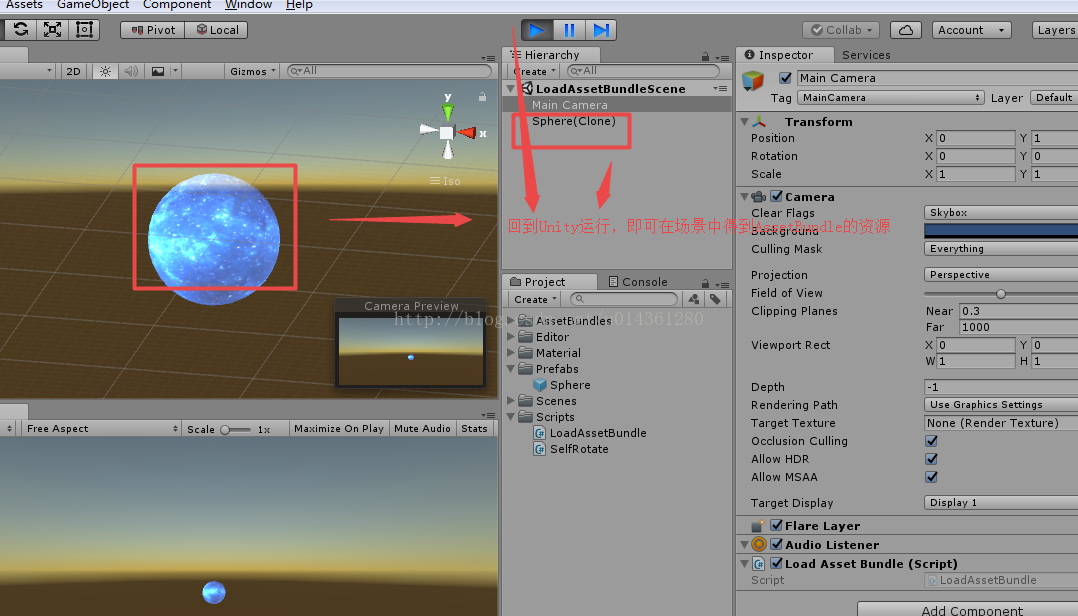
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using System.IO;
public class LoadAssetBundle : MonoBehaviour {
// Use this for initialization
void Start () {
//1.2 LoadFromMemory 方法加载
//AssetBundle的存放路径
string path = "Assets/AssetBundles/sphereab.unityab";
AssetBundle ab = AssetBundle.LoadFromMemory(File.ReadAllBytes(path));
//取得所需要的资源
GameObject go = ab.LoadAsset<GameObject>("Sphere");
//加载生成到场景中
Instantiate(go);
}
}
3.1、AssetBundle.LoadFromFile 方法加载,在脚本中添加如下代码,运行结果,如下图
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using System.IO;
public class LoadAssetBundle : MonoBehaviour {
// Use this for initialization
IEnumerator Start () {
//2.1 LoadFromFileAsync 方法加载
//AssetBundle的存放路径
string path = "Assets/AssetBundles/sphereab.unityab";
AssetBundleCreateRequest request = AssetBundle.LoadFromFileAsync(path);
//等待异步加载请求完成
yield return request;
AssetBundle ab = request.assetBundle;
//取得所需要的资源
GameObject go = ab.LoadAsset<GameObject>("Sphere");
//加载生成到场景中
Instantiate(go);
}
}
3.2、AssetBundle.LoadFromFile 方法加载,在脚本中添加如下代码,运行结果,如下图
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using System.IO;
public class LoadAssetBundle : MonoBehaviour {
// Use this for initialization
void Start () {
//2.2 LoadFromFile 方法加载
//AssetBundle的存放路径
string path = "Assets/AssetBundles/sphereab.unityab";
AssetBundle ab = AssetBundle.LoadFromFile(path);
//取得所需要的资源
GameObject go = ab.LoadAsset<GameObject>("Sphere");
//加载生成到场景中
Instantiate(go);
}
1、把“NetBox2”在一个没有中文的路径文件夹下,并新建个文本文件,随便编辑文字,如下图
2、把文本文件改名为“index.html”,双击NetBox2.exe运行,如下图
3、随后把构建好的AssetBundle包放在该文件夹下,即可模拟服务器http://127.0.0.1/*或http://localhost/*访问该资源,如下图
四、WWW 和 UnityWebRequest 加载
注意:1)链接Http时,对应的网页要打开;2)路径正反斜杠注意;
1、WWW 方法加载,在脚本中添加如下代码,运行结果,如下图
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using System.IO;
public class LoadAssetBundle : MonoBehaviour {
// Use this for initialization
IEnumerator Start () {
//3 WWW 方法加载
//判断Cache是否准备好
while (Caching.ready == false) {
yield return null;
}
//AssetBundle本地的存放路径
//string url = @"file:///D:\Unity2017\Unity2017Project\AssetBundle1112\Assets\AssetBundles\sphereab.unityab";
//AssetBundle服务器的存放路径
//string url = @"http:\\localhost\AssetBundles\sphereab.unityab";
string url = @"http:\\127.0.0.1\AssetBundles\sphereab.unityab";
WWW www = WWW.LoadFromCacheOrDownload(url, 1);
yield return www;
// 判断WWW是否有错误信息,有则退出
if (string.IsNullOrEmpty(www.error) == false) {
Debug.Log(www.error);
yield break;
}
AssetBundle ab = www.assetBundle;
//取得所需要的资源
GameObject go = ab.LoadAsset<GameObject>("Sphere");
//加载生成到场景中
Instantiate(go);
}
}
2、UnityWebRequest 方法加载,在脚本中添加如下代码,运行结果,如下图
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using System.IO;
using UnityEngine.Networking;
public class LoadAssetBundle : MonoBehaviour {
// Use this for initialization
IEnumerator Start () {
//4 UnityWebRequest 方法加载
//AssetBundle本地的存放路径
//string url = @"file:///D:\Unity2017\Unity2017Project\AssetBundle1112\Assets\AssetBundles\sphereab.unityab";
//AssetBundle服务器的存放路径
//string url = @"http://localhost/AssetBundles/sphereab.unityab";
string url = @"http://127.0.0.1/AssetBundles/sphereab.unityab";
UnityWebRequest request = UnityWebRequest.GetAssetBundle(url);
yield return request.SendWebRequest();
AssetBundle ab = DownloadHandlerAssetBundle.GetContent(request);
//AssetBundle ab = (request.downloadHandler as DownloadHandlerAssetBundle).assetBundle;
//取得所需要的资源
GameObject go = ab.LoadAsset<GameObject>("Sphere");
//加载生成到场景中
Instantiate(go);
}
}
参考资料: