CBS多机器人路径规划
单个机器人通过路径规划、运动控制,能够躲避环境中的障碍物,但会面临一个严峻的问题。当一个场景中存在多辆移动机器人时,即使每个机器人都有避障策略,也很容易就会造成道路拥堵、阻塞的情况,而且会随着机器人数量的增加变得更严峻。就像如果道路没有交通指挥系统,人们就会将有些道路挤得水泻不通,形成死锁的局面。为解决此问题,一种基于冲突的多机器人路径搜索方法(Conflict-Base search)应运而生。
CBS基本操作过程
CBS由2个搜索过程组成,底层次的搜索过程负责为每个机器人搜索出一条有效路径,高层次的负责检查路径冲突,并选择出其中代价值最小的分支重新进行底层次的路径搜索,直到高层次的搜索过程发现有效路径为止。
高层次的搜索过程
高层次的搜索过程主要有两个作用:
- 检查路径之间的冲突,并生成新的分支;
- 选出代价值最小的分支进行低层次的搜索;
路径之间的冲突分为同一时刻占据同一个节点和同一时刻调换位置两种类型的冲突,如图(1)所示
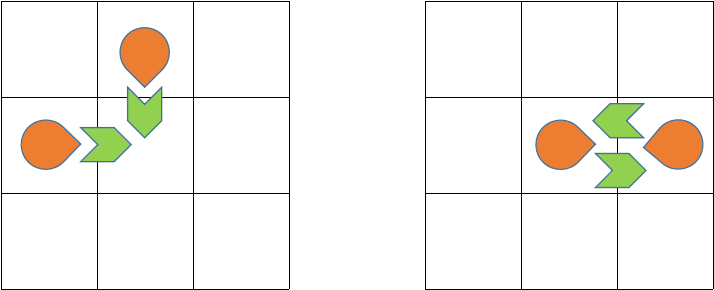
在上述两个过程完成后,选择其中代价值最小的节点进行低层次的路径搜索过程。
低层次的搜索过程
低层次的搜索过程与普通的路径规划方法类似,如Dirkstra、A*等。但其不同之处在于:
- 搜索过程中需要考虑额外的约束,即高层次搜索中添加的冲突;
- 在搜索过程中需要考虑原地等待的情况;
由于在搜索过程中需要考虑到等待的情况,因此将时间也做为一个维度加入到路径搜索过程中,通常每次扩展搜索区域时,时间增加一个单位长度。
通过高低两个搜索过程不断地运行,当问题的复杂程度不高时,能够及时得到比较好的结果。
实例讲解
以下将通过一个简单的实例讲解CBS的基本过程,实例如图2所示。
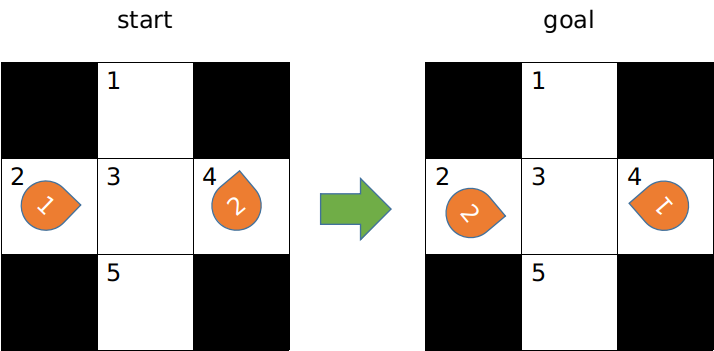
CBS的搜索过程如图3所示。
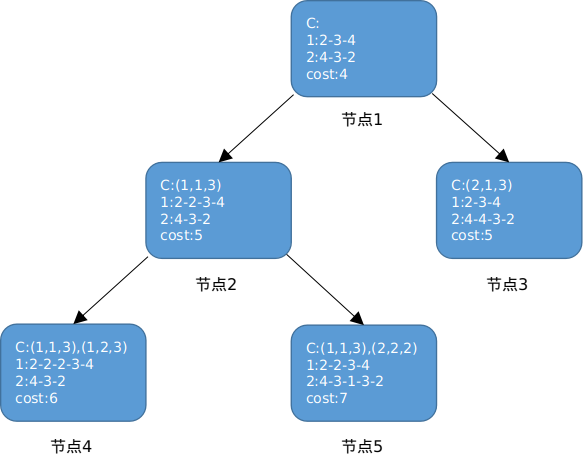
CBS开始时没有冲突约束,每个机器人按照各自的路径规划,得到节点1所示的路径结果,由于路径产生冲突,需要生成新的分支(节点2和节点3),节点2添加冲突为:1号在1时刻(从0时刻开始)不进入位置3,节点3添加冲突为:2号在1时刻不进入位置3。在约束的作用下进行低层次的搜索,节点2和节点3都搜索到了路径,但发生了新的冲突,由于节点2和节点3的代价值相等,可以从左边的节点(节点2)开始生成新的分支:节点4和节点5,然后对节点4和节点5进行低层次的搜索得到路径,最终节点5得到有效路径,搜索过程可以结束。
待改进的地方
虽然CBS做为一个比较优秀的多机器人路径规划器,依然存在一些缺点影响它在实际中的应用。
- 当环境拥挤,机器人数目多时,计算时间比较长,甚至无解;
- 无法判断有些情况是否无解,导致程序无法结束运行,且一直消耗系统内存;
- 实际情况下,机器人需要原地旋转、有加减速度、运行存在误差,需要后续进一步处理才能在实际中运行;
推荐资料
源程序:https://github.com/whoenig/libMultiRobotPlanning
论文:Conflict-based search for optimal multi-agent pathfinding