/
What is an Addressable Asset?
Making an asset "Addressable"allows you to use that asset's unique address to call it from anywhere. Whetherthat asset resides in the local application or on a content delivery network,the Addressable Asset System locates and returns it. You can load a singleAddressable Asset via its address, or load many Addressable Assets using acustom group label that you define.
Why use Addressable Assets?
Traditional means of structuring gameassets make it challenging to efficiently load content. Addressables shortenyour iteration cycles, allowing you to devote more time to designing, coding,and testing your application.
Iteration time: Referring to content by its address is extremely efficient. Optimizations to the content no longer require changes to your code.
Dependency management: The system returns all dependencies of the requested content, so that all meshes, shaders, animations, and so forth load before returning the content to you.
Memory management: The system unloads assets as well as loading them, counting references automatically and providing a robust profiler to help you spot potential memory problems.
Content packing: Because the system maps and understands complex dependency chains, it allows for efficient packing of bundles, even when moving or renaming assets. You can easily prepare assets for both local and remote deployment, to support downloadable content and reduced application size.
What about my existing game?
The Addressable Asset System providesa migration path for upgrading, whether youuse direct references, Resource folders, or asset bundles.
/
AddressableAssets Overview
The Addressable Assets System consists of two packages:
Addressable Assets package (primary package)
Scriptable Build Pipeline package (dependency)
When you install the Addressable Assets package, the ScriptableBuild Pipeline package installs at the same time.
Concepts
The following concepts are referenced throughout thisdocumentation:
Address: An asset's location identifier for easy runtime retrieval.
AddressableAssetData directory: Stores your Addressable Asset metadata in your Project’s Assets directory.
Asset group: A set of Addressable Assets available for build-time processing.
Asset group schema: Defines a set of data that you can assign to a group and use during the build.
AssetReference: An object that operates like a direct reference, but with deferred initialization. The AssetReference object stores the GUID as an Addressable that you can load on demand.
Asynchronous loading: Allows the location of the asset and its dependencies to change throughout the course of your development without changing the game code. Asynchronous loading is foundational to the Addressable Asset System.
Build script: Runs asset group processors to package assets, and provides the mapping between addresses and Resource locations for the Resource Manager.
Label: Provides an additional Addressable Asset identifier for runtime loading of similar items (for example, Addressables.DownloadDependenciesAsync("spaceHazards");).
///
Gettingstarted with Addressable Assets
Installing the Addressable Assets package
Important:The Addressable Asset System requires Unity version 2018.3 or later.
To install this package, follow the instructions in the PackageManager documentation.
Preparing Addressable Assets
Marking assets as Addressable
There are two ways to mark an asset as Addressable in the UnityEditor:
In the object's Inspector.
In the Addressables window.
Using the Inspector
In your Project window, selectthe desired asset to view its Inspector. In the Inspector, click the Address checkboxand enter a name by which to identify the asset.
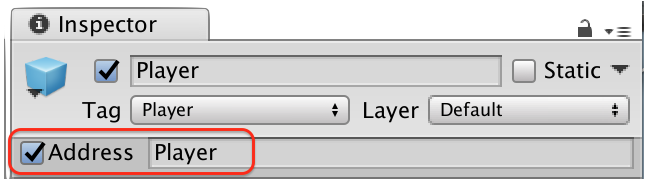
Using the Addressables window
Select Window > AssetManagement > Addressables toopen the Addressables window. Next, drag the desiredasset from your Project window intoone of the asset groups in the Addressables window.
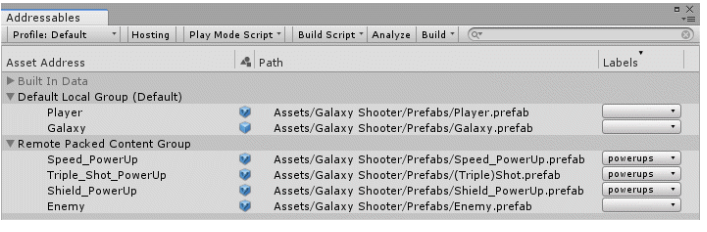
Specifying an address
The default address for your asset is the path to the asset inyour Project (for example, Assets/images/myImage.png).To change the asset's address from the Addressables window,right-click the asset and select Rename.
When you first start using Addressable Assets, the system savessome edit-time and run-time data assets for your Project in the Assets/AddressableAssetsData file,which should be added to your version control check-in.
Building your Addressable content
The Addressables Asset System needs to build your content intofiles that can be consumed by the running game before you build theapplication. This step is not automatic. You can build this content via theEditor or API:
To build content in the Editor, open the Addressables window, then select Build > Build Player Content.
To build content using the API, use AddressableAssetSettings.BuildPlayerContent().
Using Addressable Assets
Loading or instantiating by address
You can load or instantiate an Addressable Asset at run-time.Loading an asset loads all dependencies into memory (including the asset'sbundle data if applicable), allowing you to use the asset when you need to.This does not actually put the desired asset into your scene. To add the assetto your scene you must instantiate. Using Addressables instantiation intefraceswill load the asset, then immediately adds it to your Scene.
To access an asset from your game script using a string address,declare the UnityEngine.AddressableAssets namespace,then call the following methods:
Addressables.LoadAssetAsync<GameObject>("AssetAddress");
This loads the asset with the specified address.
Addressables.InstantiateAsync("AssetAddress");
This instantiates the asset with the specified address into yourScene.
Note: LoadAssetAsync and InstantiateAsync areasynchronous operations. You may provide a callback to work with the asset whenit finishes loading (see documentation on Async operation handling for moreinformation).
using System.Collections;
using System.Collections.Generic;
using UnityEngine.AddressableAssets;
using UnityEngine;
public class AddressablesExample : MonoBehaviour {
GameObject myGameObject;
...
Addressables.LoadAssetAsync<GameObject>("AssetAddress").Completed += OnLoadDone;
}
privatevoidOnLoadDone(UnityEngine.ResourceManagement.AsyncOperations.AsyncOperationHandle<GameObject> obj)
{
// In a production environment, you should add exception handling to catch scenarios such as a null result.
myGameObject = obj.Result;
}
}
Sub-assets and components
Sub-assets and components are special cases for asset loading.
Components: You cannot load a GameObject's component directly as an asset. You must load or instantiate the GameObject, then retrieve the component reference from it.
Sub-assets: The system supports loading sub-assets, but requires special syntax. Examples of potential sub-assets include sprites in a sprite sheet, or animation clips in an FBX file. To load them, use the following example syntax:
Addressables.LoadAssetAsync<IList<Sprite>>("MySpriteSheetAddress");
Using the AssetReference class
The AssetReference classprovides a way to access Addressable Assets without needing to know theiraddresses. To access an Addressable Asset using the AssetReference class:
Select a GameObject from your Scene hierarchy or Project window.
In the Inspector, click the Add Component button, then select the component type. Any serializable component can support an AssetReference variable (for example, a game script, ScriptableObject, or other serializable class).
Add a public AssetReference variable in the component (for example, public AssetReference explosion;).
In the Inspector, select which Addressable Asset to link to the object, by either dragging the asset from the Project window into the exposed AssetReference field, or choosing from the dropdown of previously defined Addressable Assets in your Project (shown below).
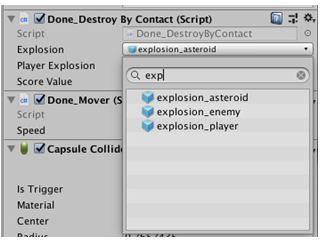
To load or instantiate an AssetReference asset,call its corresponding method. For example:
AssetRefMember.LoadAssetAsync<GameObject>();
or
AssetRefMember.InstantiateAsync(pos, rot);
Note:As with normal Addressable Assets, LoadAssetAsync and InstantiateAsync areasynchronous operations. You may provide a callback to work with the asset whenit finishes loading (see documentation on Async operation handling for moreinformation).
Buildconsiderations
Local data in StreamingAssets
The Addressable Asset System needs some files at runtime to knowwhat to load and how to load it. Those files are generated when you buildAddressables data and wind up in the StreamingAssets folder,which is a special folder in Unity that includes all its files in the build.When you build Addressables content, the system stages those files in theLibrary. Then, when you build the application, the system copies the requiredfiles over to StreamingAssets, builds, and deletes them fromthe folder. This way, you can build data for multiple platforms while onlyhaving the relevant data included in each build.
In addition to the Addressables-specific data, any groups thatbuild their data for local use will also use the Library platform-specificstaging location. To verify that this works, set your build path and load pathsto profile variables starting with [UnityEngine.AddressableAssets.Addressables.BuildPath] and {UnityEngine.AddressableAssets.Addressables.RuntimePath} respectively.You can specify these settings in the AddressableAssetSettings Inspector(by default, this object is located in your Project's Assets/AddressableAssetsData directory).
Downloading in Advance
Calling the Addressables.DownloadDependenciesAsync() methodloads the dependencies for the address or label that you pass in. Typically,this is the asset bundle.
The AsyncOperationHandle structreturned by this call includes a PercentComplete attributethat you can use to monitor and display download progress. You can also havethe app wait until the content has loaded.
If you wish to ask the user for consent prior to download, use Addressables.GetDownloadSize() toreturn how much space is needed to download the content from a given address orlabel. Note that this takes into account any previously downloaded bundles thatare still in Unity's asset bundle cache.
While it can be advantageous to download assets for your app inadvance, there are instances where you might choose not do so. For example:
If your app has a large amount of online content, and you generally expect users to only ever interact with a portion of it.
You have an app that must be connected online to function. If all your app's content is in small bundles, you might choose to download content as needed.
Rather than using the percent complete value to wait until thecontent is loaded, you can use the preload functionality to show that thedownload has started, then continue on. This implementation would require aloading or waiting screen to handle instances where the asset has not finishedloading by the time it's needed.
Building for multiple platforms
The Addressable Asset System generates asset bundles containingyour Addressable Assets when building application content. Asset bundles areplatform-dependant, and thus must be rebuilt for every unique platform youintend to support.
By default, when building Addressables app data, data for yourgiven platform is stored in platform-specific subdirectories of theAddressables build path(s). The runtime path accounts for these platformfolders, and points to the applicable app data.
Note:If you use the Addressables BuildScriptPackedPlayMode scriptin the Editor Play mode, Addressables will attempt to load data for yourcurrent active build target. As such, issues may arise if your current buildtarget data isn't compatible with your current Editor platform. For more information,see documentation on Playmode scripts.
//
AddressableAssets development cycle
One of the key benefits of Addressable Assets is decoupling howyou arrange, build, and load your content. Traditionally, these facets of developmentare heavily tied together.
Traditional asset management
If you arrange content in Resources directories,it gets built into the base application and you must load the content using the Resources.Load method,supplying the path to the resource. To access content stored elsewhere, youwould use direct references or asset bundles. If you use asset bundles, youwould again load by path, tying your load and organization strategies together.If your asset bundles are remote, or have dependencies on other bundles, youhave to write code to manage downloading, loading, and unloading all of yourbundles.
Addressable Asset management
Giving an asset an address allows you to load it using thataddress, no matter where it is in your Project or how you built the asset. Youcan change an Addressable Asset’s path or filename without issue. You can alsomove the Addressable Asset from the Resources folder, orfrom a local build destination, to some other build location (including remoteones), without ever changing your loading code.
Asset group schemas
Schemas define a set of data. You can attach schemas to assetgroups in the Inspector. The set of schemas attached to a group defines how thebuild processes its contents. For example, when building in packed mode, groupswith the BundledAssetGroupSchema schemaattached to them act as sources for asset bundles. You can combine sets ofschemas into templates that you use to define new groups. You can add schematemplates via the AddressableAssetsSettings Inspector.
Build scripts
Build scripts are represented as ScriptableObject assetsin the Project that implement the IDataBuilder interface.Users can create their own build scripts and add them to the AddressableAssetSettings objectthrough its Inspector. To apply a build script in the Addressableswindow (Window > AssetManagement > Addressables), select BuildScript, and choose a dropdown option. Currently, there arethree scripts implemented to support the full application build, and three Playmode scripts for iterating in the Editor.
Playmode scripts
The Addressable Assets package has three build scripts that createPlay mode data to help you accelerate app development.
Fastmode
Fast mode (BuildScriptFastMode)allows you to run the game quickly as you work through the flow of your game.Fast mode loads assets directly through the asset database for quick iterationwith no analysis or asset bundle creation.
Virtualmode
Virtual mode (BuildScriptVirtualMode)analyzes content for layout and dependencies without creating asset bundles.Assets load from the asset database though the ResourceManager,as if they were loaded through bundles. To see when bundles load or unloadduring game play, view the asset usage in the Addressable Profiler window (Window > AssetManagement > Addressable Profiler).
Virtual mode helps you simulate load strategies and tweak yourcontent groups to find the right balance for a production release.
PackedPlay mode
Packed Play mode (BuildScriptPackedPlayMode)uses asset bundles that are already built. This mode most closely matches adeployed application build, but it requires you to build the data as a separatestep. If you aren't modifying assets, this mode is the fastest since it doesnot process any data when entering Play mode. You must either build the contentfor this mode in the Addressables window (Window > AssetManagement > Addressables) byselecting Build > BuildPlayer Content, or using the AddressableAssetSettings.BuildPlayerContent() methodin your game script.
Choosing the right script
To apply a Play mode script, from the Addressableswindow menu (Window > AssetManagement > Addressables), select PlayMode Script and choose from the dropdown options. Eachmode has its own time and place during development and deployment. Thefollowing table illustrates stages of the development cycle, in which aparticular mode is useful.
Design | Develop | Build | Test / Play | Publish | |
Fast | x | x | In-Editor only | ||
Virtual | x | x | Asset bundle layout | In-Editor only | |
Packed | Asset bundles | x | x |
Analysisand debugging
By default, Addressable Assets only logs warnings and errors.You can enable detailed logging by opening the Player settingswindow (Edit > ProjectSettings > Player), navigating tothe OtherSettings > Configuration section,and adding "ADDRESSABLES_LOG_ALL" tothe ScriptingDefine Symbols field.
You can also disable exceptions by unchecking the LogRuntime Exceptions option in the AddressableAssetSettings objectInspector. You can implement the ResourceManager.ExceptionHandler propertywith your own exception handler if desired, but this should be done afterAddressables finishes runtime initialization (see below).
Initializationobjects
You can attach objects to the Addressable Assets settings andpass them to the initialization process at runtime. The CacheInitializationSettings objectcontrols Unity's caching API at runtime. To create your own initializationobject, create a ScriptableObject that implements the IObjectInitializationDataProvider interface.This is the Editor component of the system responsible for creating the ObjectInitializationData thatis serialized with the runtime data.
Contentupdate workflow
Unity recommends structuring your game content into twocategories:
Static content that you never expect to update.
Dynamic content that you expect to update.
In this structure, static content ships with the application (ordownloads soon after install), and resides in very few large bundles. Dynamiccontent resides online, ideally in smaller bundles to minimize the amount ofdata needed for each update. One of the goals of the Addressable Assets Systemis to make this structure easy to work with and modify without having to changeyour scripts.
However, the Addressable Assets System can also accommodatesituations that require changes to the "static" content, when youdon't want to publish a whole new application build.
Howit works
Addressables uses a content catalog to map an address to eachasset, specifying where and how to load it. In order to provide your app withthe ability to modify that mapping, your original app must be aware of anonline copy of this catalog. To set that up, enable the BuildRemote Catalog setting on the AddressableAssetSettings Inspector.This ensures that a copy of the catalog gets built to and loaded from thespecified paths. This load path cannot change once your app has shipped. Thecontent update process creates a new version of the catalog (with the same filename) to overwrite the file at the previously specified load path.
Building an application generates a unique app content versionstring, which identifies what content catalog each app should load. A givenserver can contain catalogs of multiple versions of your app without conflict.We store the data we need in the addressables_content_state.bin file.This includes the version string, along with hash information for any assetthat is contained in a group marked as StaticContent. Bydefault, this file is located in the same folder as your AddressableAssetSettings.asset file.
The addressables_content_state.bin filecontains hash and dependency information for every StaticContent assetgroup in the Addressables system. All groups building to the StreamingAssets foldershould be marked as StaticContent, thoughlarge remote groups may also benefit from this designation. During the nextstep (preparing for content update, described below), this hash informationdetermines if any StaticContent groupscontain changed assets, and thus need those assets moved elsewhere.
Preparing for content updates
If you have modified assets in any StaticContent groups,you'll need to run the Prepare For Content Update command.This will take any modified asset out of the static groups and move them to anew group. To generate the new asset groups:
Open the Addressables window in the Unity Editor (Window > Asset Management > Addressable Assets).
In the Addressables window, select Build on the top menu bar, then Prepare For Content Update.
In the Build Data File dialog that opens, select the addressables_content_state.bin file (by default, this is located in the Assets/AddressableAssetsData Project directory.
This data is used to determine which assets or dependencies havebeen modified since the application was last built. The system moves theseassets to a new group in preparation for the content update build.
Note:This command will do nothing if all your changes are confined to non-staticgroups.
Important:Before running the prepare operation, Unity recommends branching your versioncontrol system. The prepare operation rearranges your asset groups in a waysuited for updating content. Branching ensures that next time you ship a newplayer, you can return to your preferred content arrangement.
Building for content updates
To build for a content update:
Open the Addressables window in the Unity Editor (Window > Asset Management > Addressable Assets).
In the Addressables window, select Build on the top menu, then Build For Content Update.
In the Build Data File dialog that opens, select the build folder of an existing application build. The build folder must contain an addressables_content_state.bin file.
The build generates a content catalog, a hash file, and theasset bundles.
The generated content catalog has the same name as the catalogin the selected application build, overwriting the old catalog and hash file.The application loads the hash file to determine if a new catalog is available.The system loads unmodified assets from existing bundles that were shipped withthe application or already downloaded.
The system uses the content version string and locationinformation from the addressables_content_state.bin fileto create the asset bundles. Asset bundles that do not contain updated contentare written using the same file names as those in the build selected for theupdate. If an asset bundle contains updated content, a new asset bundle isgenerated that contains the updated content, with a new file name so that itcan coexist with the original. Only asset bundles with new file names must becopied to the location that hosts your content.
The system also builds asset bundles for static content, but youdo not need to upload them to the content hosting location, as no Addressablesasset entries reference them.
Content update examples
In this example, a shipped application is aware of the followinggroups:
Local_Static | Remote_Static | Remote_NonStatic |
AssetA | AssetL | AssetX |
AssetB | AssetM | AssetY |
AssetC | AssetN | AssetZ |
As this version is live, there are players that have Local_Static ontheir devices, and potentially have either or both of the remote bundles cachedlocally.
If you modify one asset from each group (AssetA, AssetL,and AssetX), then run PrepareFor Content Update, the results in your local Addressablesettings are now:
Local_Static | Remote_Static | Remote_NonStatic | content_update_group (non-static) |
AssetX | AssetA | ||
AssetB | AssetM | AssetY | AssetL |
AssetC | AssetN | AssetZ |
Note that the prepare operation actually edits the staticgroups, which may seem counter intuitive. The key, however, is that the systembuilds the above layout, but discards the build results for any static groups.As such, you end up with the following from a player's perspective:
Local_Static |
AssetA |
AssetB |
AssetC |
The Local_Static bundleis already on player devices, which you can't change. This old version of AssetA isno longer referenced. Instead, it is stuck on player devices as dead data.
Remote_Static |
AssetL |
AssetM |
AssetN |
The Remote_Static bundleis unchanged. If it is not already cached on a player's device, it willdownload when AssetM or AssetN isrequested. Like AssetA, this old version of AssetL isno longer referenced.
Remote_NonStatic (old) |
AssetX |
AssetY |
AssetZ |
The Remote_NonStatic bundleis now old. You could delete it from the server, but either way it will not bedownloaded from this point forward. If cached, it will eventually leave thecache. Like AssetA and AssetL,this old version of AssetX is no longer referenced.
Remote_NonStatic (new) |
AssetX |
AssetY |
AssetZ |
The old Remote_NonStatic bundleis replaced with a new version, distinguished by its hash file. The modifiedversion of AssetX is updated with this newbundle.
content_update_group |
AssetA |
AssetL |
The content_update_group bundleconsists of the modified assets that will be referenced moving forward.
Here are the implications of this example:
Any changed local assets remain unused on the user's device forever.
If the user already cached a non-static bundle, they will need to re-download the bundle, including the unchanged assets (in this instance, for example, AssetY and AssetZ). Ideally, the user has not cached the bundle, in which case they simply need to download the new Remote_NonStatic bundle.
If the user has already cached the Static_Remote bundle, they only need to download the updated asset (in this instance, AssetL via content_update_group). This is ideal in this case. If the user has not cached the bundle, they must download both the new AssetL via content_update_group and the now-defunct AssetL via the untouched Remote_Static bundle. Regardless of the initial cache state, at some point the user will have the defunct AssetL on their device, cached indefinitely despite never being accessed.
The best setup for your remote content will depend on yourspecific use case.
//
AssetHosting Services
Overview
Hosting Services provide an integrated facility for usingAddressable Assets configuration data to serve packed content to local ornetwork-connected application builds from within the Unity Editor. HostingServices are designed to improve iteration velocity when testing packedcontent, and can also be used to serve content to connected clients on localand remote networks.
Packed mode testing and iteration
Moving from Editor Play mode testing to platform applicationbuild testing introduces complexities and time costs to the developmentprocess. Hosting Services provide extensible Editor-embedded content deliveryservices that map directly to your Addressables group configuration. Using acustom Addressables profile, you can quickly configure your application to loadall content from the Unity Editor itself. This includes builds deployed tomobile devices, or any other platform, that have network access to yourdevelopment system.
Turn-key content server
You can deploy Asset Hosting Services into a server environmentby running in batch mode (headless) to host content for both intranet- andinternet-facing Unity application clients.
Setup
This article details the initial setup of Asset Hosting Servicesfor your Project. While the setup guide focuses on Editor workflows, you can usethe API to configure Hosting Services by setting the HostingServicesManager propertyof the AddressableAssetSettings class.
Configuring a new Hosting Service
Use the Hosting window toadd, configure, and enable new Hosting Services. In the Editor, select Window > AssetManagement > Hosting Services, orclick the Hosting button from the Addressableswindow menu to access the Hosting window.
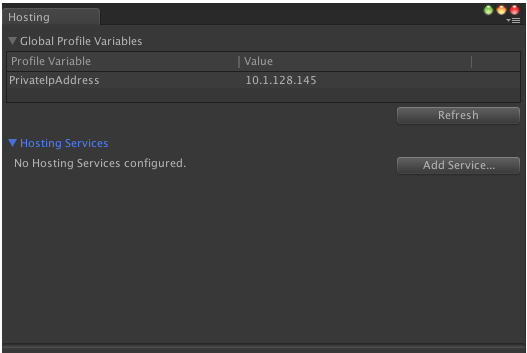
To add a new Hosting Service, click the AddService button.
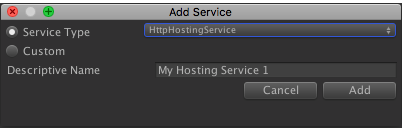
In the Add Service dialogthat appears, you can select a predefined service type or define a customservice type. To use a predefined service type, choose from the ServiceType drop-down options. Use the DescriptiveName field enter a name for the service.
Note:For more information on implementing custom hosting service types, see thesection on custom services.
The newly added service appears in the HostingServices section of the Hosting window, anddefaults to the disabled state. To initiate the service, click the EnableService button.
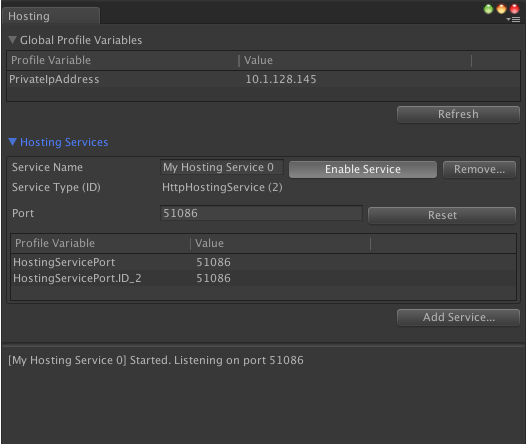
The HTTP Hosting Service automatically assigns a port numberwhen it starts. The port number is saved and reused between Unity sessions. Tochoose a different port, either assign a specific port number in the Port field,or use the Reset button to randomly assign adifferent port.
Note:If you reset the port number, you must execute a full application build togenerate and embed the correct URL.
The HTTP Hosting Service is now enabled and ready to servecontent from the directory specified in the BuildPath ofeach asset group.
Profilesetup
When working with Hosting Services during development, Unityrecommends creating a profile that configures all asset groups to load contentfrom the Hosting Service using a directory or directories created specificallyfor that purpose.
In the Addressables window menu(Window > AssetManagement > Addressable Assets),select Profiles > InspectProfile Settings. You can also access these settings via the AddressableAssetSettings Inspector.
Next, create a new profile. In the following example, the newprofile is called "Editor Hosted".
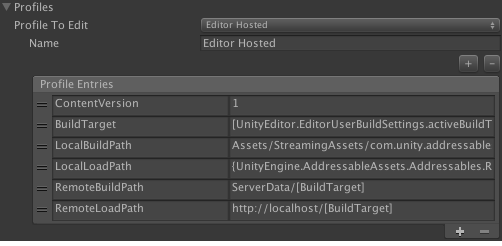
Modify the loading path fields to instead load from the HostingService. HttpHostingService isa URL that uses the local IP address and the port assigned to the service. Fromthe Hostingwindow, you can use the profile variables named PrivateIpAddress and HostingServicePort toconstruct the url (for example, http://[PrivateIpAddress]:[HostingServicePort]).
Additionally, you should modify all build path variables topoint to a common directory outside of the Project's Assets folder.
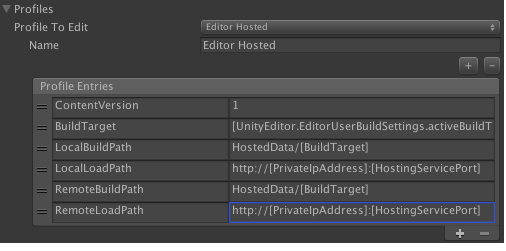
Verify that each group is configured correctly. Ensure that the BuildPath and LoadPath pathsare set to their respective profile keys that are modified for use with HostingServices. In this example, you can see how the profile variables in the LoadPath areexpanded to build a correct base URL for loading from Hosted Services.
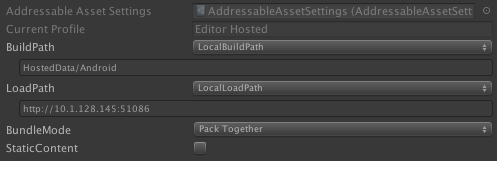
Finally, select the new profile from the Addressableswindow, create a build, and deploy to the target device. TheUnity Editor now serves all load requests from the application through the HttpHostingService service.You can now make additions and changes to content without redeployment. Rebuildthe Addressable content, and relaunch the already deployed application torefresh the content.
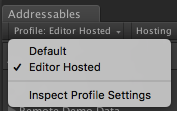
Batchmode
You can also use Hosting Services to serve content from theUnity Editor running in batch mode. To do so, launch Unity from the commandline with the following options:
-batchMode -executeMethod UnityEditor.AddressableAssets.HostingServicesManager.BatchMode
This loads the Hosting Services configuration from the default AddressableAssetSettings object,and starts all configured services.
To use an alternative AddressableAssetSettings configuration,create your own static method entry point, to call through the UnityEditor.AddressableAssets.HostingServicesManager.BatchMode(AddressableAssetSettings
settings) overload.
Custom Services
Hosting Services are designed to be extensible, allowing you toimplement your own custom logic for serving content-loading requests from theAddressable Assets System. For example:
Support a custom IResourceProvider that uses a non-HTTP protocol for downloading content.
Manage an external process for serving content that matches your production CDN solution (such as an Apache HTTP server).
Implementing a custom service
The HostingServicesManager canmanage any class that implements an IHostingService interface(for more details on method parameters and return values, see the API documentation.
To create a new custom service:
Follow the steps outlined in the configuring a new Hosting Service section above to access the Add Service dialog.
Select Custom, then drag and drop the applicable script into the field, or select it from the object picker. The dialog validates that the selected script implements the IHostingService interface.
To finish adding the service, click the Add button.
Moving forward, your custom service will appear in the ServiceType drop-down options.
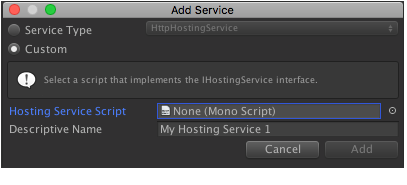
///
Memorymanagement
Mirroring load and unload
When working with Addressable Assets, the primary way to ensureproper memory management is to mirror your load and unload calls correctly. Howyou do so depends on your asset types and load methods. In all cases, however,the release method can either take the loaded asset, or an operation handlereturned by the load. For example, during Scene creation (described below) theload returns a AsyncOperationHandle<SceneInstance>,which you can release via this returned handle, or by keeping up with the handle.Result (inthis case, a SceneInstance).
Assetloading
To load an asset, use Addressables.LoadAssetAsync or Addressables.LoadAssetsAsync.
This loads the asset into memory without instantiating it. Everytime the load call executes, it adds one to the ref-count for each assetloaded. If you call LoadAssetAsync threetimes with the same address, you will get three different instances of an AsyncOperationHandle structback, all referencing the same underlying operation. That operation has aref-count of three for the corresponding asset. If the load succeeds, theresulting AsyncOperationHandle structcontains the asset in the .Result property.You can use the loaded asset to instantiate using Unity's built-ininstantiation methods, which does not increment the Addressables ref-count.
To unload the asset, use the Addressables.Release method,which decrements the ref-count. When a given asset's ref-count is zero, thatasset is ready to be unloaded, and decrements the ref-count of anydependencies.
Note:The asset may or may not be unloaded immediately, contingent on existingdependencies. For more information, read the section on when memory is cleared.
Sceneloading
To load a Scene, use Addressables.LoadSceneAsync.You can use this method to load a Scene in Single mode,which closes all open Scenes, or in Additive mode(for more information, see documentation on Scene mode loading.
To unload a Scene, use Addressables.UnloadSceneAsync,or open a new Scene in Single mode.You can open a new Scene by either using the Addressables interface, or usingthe SceneManager.LoadScene or SceneManager.LoadSceneAsync methods.Opening a new Scene closes the current one, properly decrementing theref-count.
GameObject instantiation
To load and instantiate a GameObject asset, use Addressables.InstantiateAsync. This instantiatesthe Prefab located by the specified location parameter. The Addressables system willload the Prefab and its dependencies, incrementing the ref-count of allassociated assets.
Calling InstantiateAsync three timeson the same address results in all dependent assets having a ref-count ofthree. Unlike calling LoadAssetAsync three times, however,each InstantiateAsync call returnsan AsyncOperationHandle pointing to aunique operation. This is because the result of each InstantiateAsync is a uniqueinstance. Another distinction between InstantiateAsync and other load calls isthe optional trackHandle parameter.When set to false, you must keep the AsyncOperationHandle to use whilereleasing your instance. This is more efficient, but requires more developmenteffort.
To destroy an instantiated gameObject, use Addressables.ReleaseInstance, or close theScene that contains the instantiated object. This Scene can have been loaded(and thus closed) in Additive or Single mode. This Scene can also have been loadedusing either the Addressables or SceneManagement API. As notedabove, if you set trackHandle to false, you can only call Addressables.ReleaseInstance with thehandle, not with the actual GameObject.
Note: If you call Addressables.ReleaseInstance on aninstance that was not created using the Addressables API, or was created with trackHandle==false, the systemdetects that and returns false to indicate that the method was unable torelease the specified instance. The instance will not be destroyed in thiscase.
Addressables.InstantiateAsync has someassociated overhead, so if you need to instantiate the same objects hundreds oftimes per frame, consider loading via the Addressables API, then instantiatingthrough other methods. In this case, you would call Addressables.LoadAssetAsync, then save theresult and call GameObject.Instantiate() for thatresult. This allows flexibility to call Instantiate in a synchronous way. The downside is thatthe Addressables system has no knowledge of how many instances you created,which can lead to memory issues if not properly managed. For example, a Prefabreferencing a texture would no longer have a valid loaded texture to reference,causing rendering issues (or worse). These sorts of problems can be hard totrack down as you may not immediately trigger the memory unload (see section on clearing memory, below).
The Addressable Profiler
Use the Addressable Profiler window tomonitor the ref-counts of all Addressables system operations. To access thewindow in the Editor, select Window > AssetManagement > Addressable Profiler.
Important:In order to view data in the profiler, you must enable the SendProfiler Events setting in your AddressableAssetSettings object'sInspector.
The following information is available in the profiler:
A white vertical line indicates the frame in which a load request occurred.
A blue background indicates that an asset is currently loaded.
The green part of the graph indicates an asset's current ref-count.
When ismemory cleared?
An asset no longer being referenced (indicated by the end of ablue section in the profiler) does not necessarily mean that asset wasunloaded. A common applicable scenario involves multiple assets in an assetbundle. For example:
You have three assets (tree, tank, and cow) in an asset bundle (stuff).
When tree loads, the profiler displays a single ref-count for tree, and one for stuff.
Later, when tank loads, the profiler displays a single ref-count for both tree and tank, and two ref-counts for the stuff bundle.
If you release tree, it's ref-count becomes zero, and the blue bar goes away.
In this example, the tree assetis not actually unloaded at this point. You can load an asset bundle, or itspartial contents, but you cannot partially unload an asset bundle. No asset in stuff willunload until the bundle itself is completely unloaded. The exception to thisrule is the engine interface Resources.UnloadUnusedAssets.Executing this method in the above scenario will cause tree tounload. Because the Addressables system cannot be aware of these events, theprofiler graph only reflects the Addressables ref-counts (not exactly whatmemory holds). Note that if you choose to use Resources.UnloadUnusedAssets,it is a very slow operation, and should only be called on a screen that won'tshow any hitches (such as a loading screen).
//
Asyncoperation handling
Several methods from the Addressables APIreturn an AsyncOperationHandle struct.The main purpose of this handle is to allow access to the status and result ofan operation. The result of the operation is valid until you call Addressables.Release or Addressables.ReleaseInstance withthe operation (for more information on releasing assets, see documentation on memorymanagement.
When the operation completes, the AsyncOperationHandle.Status propertyis either AsyncOperationStatus.Succeeded or AsyncOperationStatus.Failed.If successful, you can access the result through the AsyncOperationHandle.Result property.
You can either check the operation status periodically, orregister for a completed callback using the AsyncOperationHandle.Complete event.When you no longer need the asset provided by a returned AsyncOperationHandle struct,you should release itusing the Addressables.Release method.
Type vs typeless handles
Most Addressables APImethods return a generic AsyncOperationHandle<T>struct,allowing type safety for the AsyncOperationHandle.Completed event,and for the AsyncOperationHandle.Result object.There is also a non-generic AsyncOperationHandle struct,and you can convert between the two handles as desired.
Note that a runtime exception occurs if you attempt to cast anon-generic handle to a generic handle of an incorrect type. For example:
AsyncOperationHandle<Texture2D> textureHandle = Addressables.LoadAssetAsync<Texture2D>("mytexture");
// Convert the AsyncOperationHandle<Texture2D> to an AsyncOperationHandle:
AsyncOperationHandle nonGenericHandle = textureHandle;
// Convert the AsyncOperationHandle to an AsyncOperationHandle<Texture2D>:
AsyncOperationHandle<Texture2D> textureHandle2 = nonGenericHandle.Convert<Texture2D>();
// This will throw and exception because Texture2D is required:
AsyncOperationHandle<Texture> textureHandle3 = nonGenericHandle.Convert<Texture>();
AsyncOperationHandle use case examples
Register a listener for completion events using the AsyncOperationHandle.Completed callback:
private void TextureHandle_Completed(AsyncOperationHandle<Texture2D> handle) {
if (handle.Status == AsyncOperationStatus.Succeeded) {
Texture2D result = handle.Result;
// The texture is ready for use.
}
}
void Start() {
AsyncOperationHandle<Texture2D> textureHandle = Addressables.LoadAsset<Texture2D>("mytexture");
textureHandle.Completed += TextureHandle_Completed;
}
AsyncOperationHandle implements IEnumerator soit can be yielded in coroutines:
public IEnumerator Start() {
AsyncOperationHandle<Texture2D> handle = Addressables.LoadAssetAsync<Texture2D>("mytexture");
yield return handle;
if (handle.Status == AsyncOperationStatus.Succeeded) {
Texture2D texture = handle.Result;
// The texture is ready for use.
// ...
// Release the asset after its use:
Addressables.Release(handle);
}
}
Addressables also supports asynchronous await throughthe AsyncOperationHandle.Task property:
public async Start() {
AsyncOperationHandle<Texture2D> handle = Addressables.LoadAssetAsync<Texture2D>("mytexture");
await handle.Task;
// The task is complete. Be sure to check the Status is succeessful before storing the Result.
}
PleaseNote:
Loading scenes with SceneManager.LoadSceneAsync with allowSceneActivation set to falseor using Addressables.LoadSceneAsync and passingin false for the activateOnLoad parameter canlead to subsequent async operations being blocked and unable to complete.Please checkout the allowSceneActivation documentationhere: https://docs.unity3d.com/ScriptReference/AsyncOperation-allowSceneActivation.html
/
Customoperations
The IResourceProvider APIallows you to extend the loading process by defining locations and dependenciesin a data-driven manner.
In some cases, you might want to create a custom operation. The IResourceProvider APIis internally built on top of these custom operations.
Creating custom operations
Create custom operations by deriving from the AsyncOperationBase classand overriding the desired virtual methods. You can pass the derived operationto the ResourceManager.StartOperation methodto start the operation and receive an AsyncOperationHandle struct.Operations started this way are registered with the ResourceManager andappear in the AddressablesProfiler.
Executing the operation
The ResourceManager invokesthe AsyncOperationBase.Execute methodfor your custom operation once the optional dependent operation completes.
Completion handling
When your custom operation completes, call AsyncOperationBase.Complete onyour custom operation object. You can call this within the Execute methodor defer it to outside the call. Calling AsyncOperationBase.Complete notifiesthe ResourceManager thatthe operation is complete and will invoke the associated AsyncOperationHandle.Completed events.
Terminating the operation
The ResourceManager invokes AsyncOperationBase.Destroy methodfor your custom operation when you release the AsyncOperationHandle thatreferences it. This is where you should release any memory or resourcesassociated with your custom operation.
AddressablesAnalyze
Analyze is a tool that gathers information on your Projects'Addressables layout. In some cases, Analyze may take appropriate actions toclean up the state of your Project. In others, Analyze is purely aninformational tool that allows you to make more informed decisions about yourAddressables layout.
Using Analyze
In the Editor, open the Addressables window (Window > AssetManagement > Addressable Assets),then click the Analyze button inthe menu to open the Analyze window.
The Analyze window displays a list of Analyze rules, along withthe following operations:
Analyze Secelcted Rules
Fix Selected Rules
Clear Selected Rules
The analyze operation
The analyze operation is the information-gathering step of therule. Running this action on a rule or set of rules gathers data about thebuild, dependency maps, and more. Each rule is responsible for gathering thedesired data and reporting it back as a list of AnalyzeResult objects.
No action should be taken to modify any data or the state of theProject during the analyze step. Based on the data gathered in this step, the fix operationmay be the appropriate course of action. Some rules, however, only contain ananalyze step, as no reasonably appropriate and universal action can be takenbased on the information gathered. Check Scene to Addressable Duplicate Dependencies and Check Resources to Addressable Duplicate Dependencies areexamples of such rules.
Rules that are purely informational and contain no fix operationare categorized as Unfixable Rules. Thosethat do have a fix operation are categorized as Fixable Rules.
Thefix operation
For Fixable Rules, you maychoose to run the fix operation. This uses data gathered during the analyzestep to perform any necessary modifications and resolve the issues.
Check Duplicate Group Dependencies isan example of a fixable rule, because there is a reasonably appropriate actionthat can be taken to resolve the issues detected in the analysis.
Theclear step
This operation will remove any data gathered by the analysis andupdate the TreeView accordingly.
ProvidedAnalyze rules
Fixablerules
Check Duplicate Group Dependencies
This rule checks for potentially duplicated assets, by scanning allgroups with BundledAssetGroupSchemas andprojecting the asset group layout. This essentially requires triggering a fullbuild, so this check is time-consuming and performance-intensive.
Issues:Duplicated assets result from assets in different groups sharing dependencies,for example two Prefabs that share a material existing in different Addressablegroups. That material (and any of its dependencies) would be pulled into bothgroups containing the Prefabs. To prevent this, the material must be marked asAddressable, either with one of the Prefabs, or in its own space, therebyputting the material and its dependencies in a separate Addressable group.
Resolution:If this check discovers any issues, running the fix operation on this rulecreates a new Addressable group in which to move all dependent assets.
Exceptions:If you have an asset containing multiple objects, it is possible for differentgroups to only pull in portions of the asset, and not actually duplicate. AnFBX with many meshes is an example of this. If one mesh is in"GroupA" and another is in "GroupB", this rule will thinkthat the FBX is shared, and extract it into its own group if you run the fixoperation. In this edge case, running the fix operation is actually harmful, asneither group would have the full FBX asset.
Also note that duplicate assets may not always be an issue. Ifassets will never be requested by the same set of users (for example,region-specific assets), then duplicate dependencies may be desired, or atleast inconsequential. Each Project is unique, so fixing duplicate assetdependencies should be evaluated on a case by case basis.
Unfixablerules
Check Resources to Addressable DuplicateDependencies
This rule detects if any assets or asset dependencies are duplicatedbetween built Addressable data and assets residing in a Resources folder.
Issues:These duplications mean that data will be included in both the applicationbuild and the Addressables build.
Resolution:This rule is unfixable, because no appropriate action exists. It is purelyinformational, alerting you to the redundancy. You must decide how to proceedand what action to take, if any. One example of a possible manual fix is tomove the offending asset(s) out of the Resources folder, andmake them Addressable.
Check Scene to Addressable Duplicate Dependencies
This rule detects any assets or asset dependencies that are sharedbetween the Scenes in the Editor Scene list and Addressables.
Issues:These duplications mean that data will be included in both the applicationbuild and the Addressables build.
Resolution:It is purely informational, alerting you to the redundancy. You must decide howto proceed and what action to take, if any. One example of a possible manualfix is to pull the built-in Scene(s) with duplicated references out of BuildSettings and make it an Addressable Scene.
ExtendingAnalyze
Each unique Project may require additional Analyze rules beyondwhat comes pre-packaged. The Addressable Assets System allows you to createyour own custom rule classes.
AnalyzeRule objects
Create a new child class of the AnalyzeRule class,overriding the following properties:
CanFix tells Analyze if the rule is deemed fixable or not.
ruleName is the display name you'll see for this rule in the Analyze window.
You'll also need to override the following methods, which aredetailed below:
List<AnalyzeResult> RefreshAnalysis(AddressableAssetSettings settings)
void FixIssues(AddressableAssetSettings settings)
void ClearAnalysis().
Note:If your rule is designated unfixable, you don't have to override the FixIssues method.
RefreshAnalysis
This is your analyze operation. In this method, perform anycalculations you'd like and cache any data you might need for a potential fix.The return value is a List<AnalyzeResult> list.After you'd gathered your data, create a new AnalyzeResult foreach entry in your analysis, containing the data as a string for the firstparameter and a MessageType for the second (tooptionally designate the message type as a warning or error). Return the listof objects you create.
If you need to make child elements in the TreeView fora particular AnalyzeResult object,you can delineate the parent item and any children with kDelimiter.Include the delimiter between the parent and child items.
FixIssues
This is your fix operation. If there is an appropriate action totake in response to the analyze step, execute it here.
ClearAnalysis
This is your clear operation. Any data you cached in the analyzestep can be cleaned or removed in this function. The TreeView willupdate to reflect the lack of data.
Adding custom rules to the GUI
A custom rule must register itself with the GUI class using AnalyzeWindow.RegisterNewRule<RuleType>(),in order to show up in the Analyze window. Forexample:
class MyRule : AnalyzeRule {}
[InitializeOnLoad]
class RegisterMyRule
{
staticRegisterMyRule()
{
AnalyzeWindow.RegisterNewRule<MyRule>();
}
}
/
Upgradingto the Addressables system
This article details how to modify your existing Project to takeadvantage of Addressable Assets. There are three traditional methods forreferencing assets:
Direct References: Add assets directly into components or Scenes, which the application loads automatically.
Resource Folders: Add assets to your Resource folder and load them by filename.
Asset Bundles: Add assets to asset bundles, then load them with their dependencies by file path.
The direct reference method
To migrate from this approach, follow these steps:
Replace your direct references to objects with asset references (for example, public GameObject directRefMember; becomes public AssetReference AssetRefMember;).
Drag assets onto the appropriate component’s Inspector, as you would for a direct reference.
If you'd like to load an asset based on an object rather than a string name, instantiate it directly from the AssetReference object you created in your setup (for example, AssetRefMember.LoadAssetAsync<GameObject>(); or AssetRefMember.InstantiateAsync(pos, rot);).
Note:The Addressable Asset System loads assets asynchronously. When you update yourdirect references to asset references, you must also update your code tooperate asynchronously.
The Resource folders method
When you mark an asset in a Resources folder asAddressable, the system automatically moves the asset from the Resources folderto a new folder in your Project named Resources_moved. The defaultaddress for a moved asset is the old path, omitting the folder name. Forexample, your loading code might change from Resources.LoadAsync<GameObject>("desert/tank.prefab"); to Addressables.LoadAssetAsync<GameObject>("desert/tank.prefab");.
The asset bundles method
When you open the Addressables window,Unity offers to convert all asset bundles into Addressable Asset groups. Thisis the easiest way to migrate your code.
If you choose to convert your Assets manually, click the Ignore button.Then, either use the direct reference or resource folder methods previouslydescribed.
The default path for the address of an asset is its file path.If you use the path as the asset's address, you'd load the asset in the samemanner as you would load from a bundle. The Addressable Asset System handlesthe loading of the bundle and all its dependencies.
//
Upgradingto the Addressables system
This article details how to modify your existing Project to takeadvantage of Addressable Assets. There are three traditional methods forreferencing assets:
Direct References: Add assets directly into components or Scenes, which the application loads automatically.
Resource Folders: Add assets to your Resource folder and load them by filename.
Asset Bundles: Add assets to asset bundles, then load them with their dependencies by file path.
The direct reference method
To migrate from this approach, follow these steps:
Replace your direct references to objects with asset references (for example, public GameObject directRefMember; becomes public AssetReference AssetRefMember;).
Drag assets onto the appropriate component’s Inspector, as you would for a direct reference.
If you'd like to load an asset based on an object rather than a string name, instantiate it directly from the AssetReference object you created in your setup (for example, AssetRefMember.LoadAssetAsync<GameObject>(); or AssetRefMember.InstantiateAsync(pos, rot);).
Note:The Addressable Asset System loads assets asynchronously. When you update yourdirect references to asset references, you must also update your code tooperate asynchronously.
The Resource folders method
When you mark an asset in a Resources folder asAddressable, the system automatically moves the asset from the Resources folderto a new folder in your Project named Resources_moved. The defaultaddress for a moved asset is the old path, omitting the folder name. Forexample, your loading code might change from Resources.LoadAsync<GameObject>("desert/tank.prefab"); to Addressables.LoadAssetAsync<GameObject>("desert/tank.prefab");.
The asset bundles method
When you open the Addressables window,Unity offers to convert all asset bundles into Addressable Asset groups. Thisis the easiest way to migrate your code.
If you choose to convert your Assets manually, click the Ignore button.Then, either use the direct reference or resource folder methods previouslydescribed.
The default path for the address of an asset is its file path.If you use the path as the asset's address, you'd load the asset in the samemanner as you would load from a bundle. The Addressable Asset System handlesthe loading of the bundle and all its dependencies.
https://docs.unity3d.com/Packages/com.unity.addressables@1.1/manual/index.html